Sample Chaincode
The following is an example chaincode for reading and writing data. You can also refer to other chaincodes in the official examples provided by Fabric.
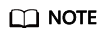
When creating a Java project, you can create a Maven or Gradle project to import the dependency package. A Gradle project is used in the following example.
/* Before importing the following code to the build.gradle file of the project, delete or comment out the original content in this file.*/ buildscript { repositories { mavenLocal() maven{ url "https://mirrors.huaweicloud.com/repository/maven/" } } dependencies { classpath 'com.github.jengelman.gradle.plugins:shadow:2.0.4' } } apply plugin: 'com.github.johnrengelman.shadow' apply plugin: 'java' sourceCompatibility = 1.8 //Code repository where the dependency package is searched and downloaded. repositories { mavenLocal() maven{ url "https://mirrors.huaweicloud.com/repository/maven/" } maven{ url "https://jitpack.io" }/* If JSON Schema cannot be imported, try to add it to this repository.*/ } //Import the dependency package required by the code. dependencies { compile group: 'org.hyperledger.fabric-chaincode-java', name: 'fabric-chaincode-shim', version: '2.2.+' testCompile group: 'junit', name: 'junit', version: '4.12' //testCompile indicates that this package is used only for debugging. testCompile 'org.mockito:mockito-core:2.4.1' } shadowJar { baseName = 'chaincode' version = null classifier = null manifest { //The path must be the same as that of the class that extends ChaincodeBase. attributes 'Main-Class': 'org.hyperledger.fabric.example.SimpleChaincode' } }
//The following is the content in the SimpleChaincode class. package org.hyperledger.fabric.example;//The actual location of the chaincode file, which is usually automatically generated. //You only need to configure the required packages in Maven or Gradle. The packages are imported automatically. import java.util.List; import com.google.protobuf.ByteString; import org.apache.commons.logging.Log; import org.apache.commons.logging.LogFactory; import org.hyperledger.fabric.shim.ChaincodeBase; import org.hyperledger.fabric.shim.ChaincodeStub; import static java.nio.charset.StandardCharsets.UTF_8; // SimpleChaincode example simple Chaincode implementation public class SimpleChaincode extends ChaincodeBase { private static Log logger = LogFactory.getLog(SimpleChaincode.class); @Override public Response init(ChaincodeStub stub) { logger.info("Init"); return newSuccessResponse(); } @Override public Response invoke(ChaincodeStub stub) { try { logger.info("Invoke java simple chaincode"); String func = stub.getFunction(); List<String> params = stub.getParameters(); if (func.equals("insert")) { return insert(stub, params); } if (func.equals("query")) { return query(stub, params); } return newErrorResponse("Invalid invoke function name. Expecting one of: [\"insert\", \"query\"]"); } catch (Throwable e) { return newErrorResponse(e); } } // The Insert method implements the data storage function and stores the key-value on the chain private Response insert(ChaincodeStub stub, List<String> args) { if (args.size() != 2) { return newErrorResponse("Incorrect number of arguments. Expecting 2"); } String key = args.get(0); String val = args.get(1); stub.putState(key, ByteString.copyFrom(val, UTF_8).toByteArray()); return newSuccessResponse(); } // The Query method implements the data query function by invoking the API to query the value of the key // API to query the value corresponding to a key private Response query(ChaincodeStub stub, List<String> args) { if (args.size() != 1) { return newErrorResponse("Incorrect number of arguments. Expecting name of the person to query"); } String key = args.get(0); // Get the value of key String val = stub.getStringState(key); if (val == null) { String jsonResp = "{\"Error\":\"Null val for " + key + "\"}"; return newErrorResponse(jsonResp); } logger.info(String.format("Query Response:\nkey: %s, val: %s\n", key, val)); return newSuccessResponse(val, ByteString.copyFrom(val, UTF_8).toByteArray()); } public static void main(String[] args) { new SimpleChaincode().start(args); } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot