Chaincode Debugging
To debug a chaincode, you can use MockStub to perform unit tests on the chaincode. To obtain the chaincode used in this section, go to the BCS console and click Use Cases. Download Chaincode_Java_Local_Demo in the Java SDK Demo area.
Adding the Dependency
To use the mock() method, add the Mockito dependency.
- Gradle
- Maven
Writing Test Code
If the test folder does not exist during project creation, create it under src. Select test\java under Gradle Source Sets, and create the SimpleChaincodeTest.java test file, as shown in the following figures:
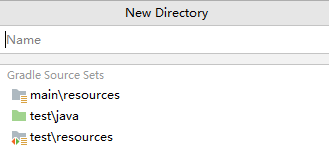
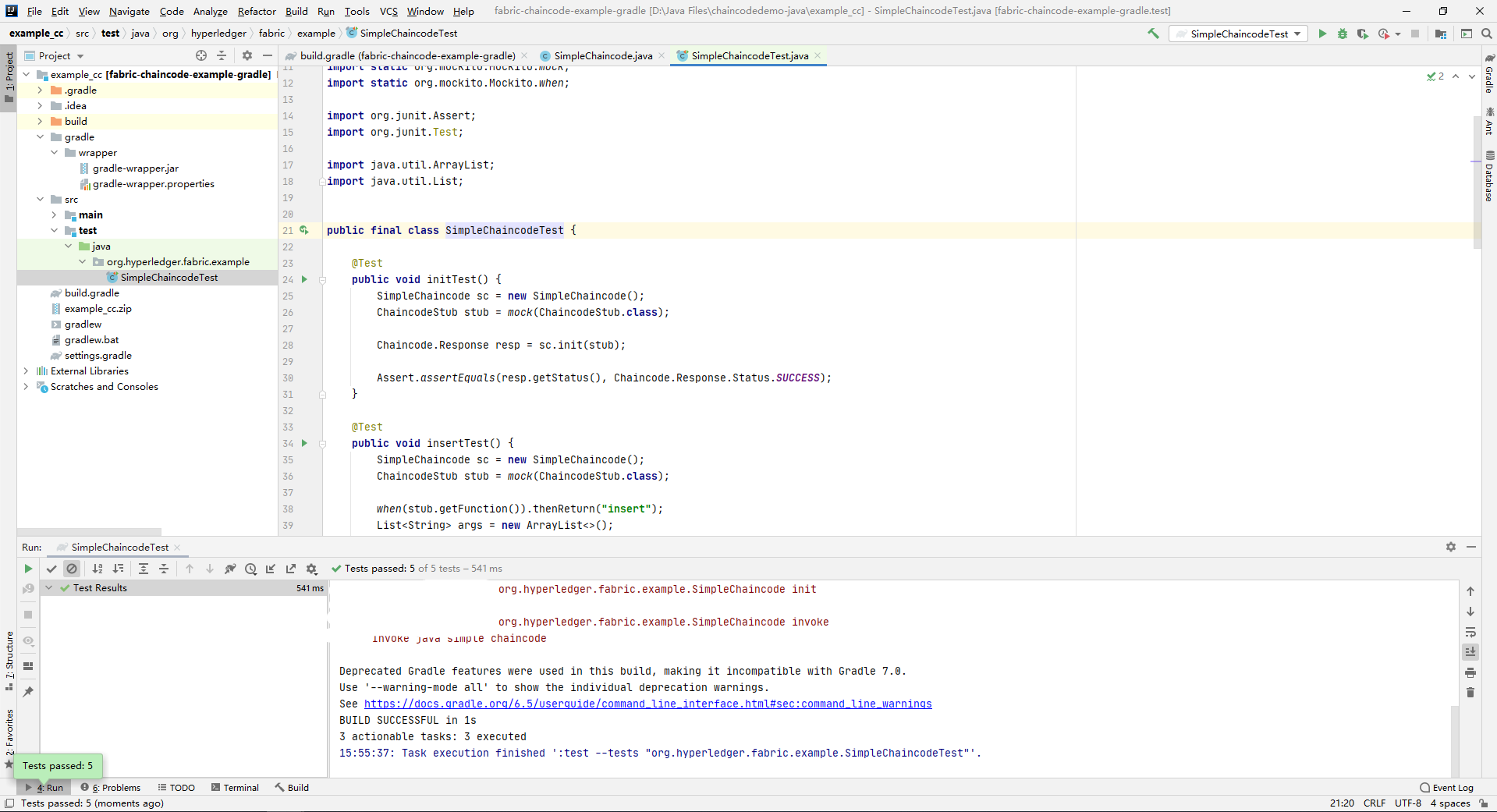
The content of the SimpleChaincodeTest.java test code is as follows:
import org.hyperledger.fabric.example.SimpleChaincode; import org.hyperledger.fabric.shim.Chaincode; import org.hyperledger.fabric.shim.ChaincodeStub; import org.junit.Assert; import org.junit.Test; import java.util.ArrayList; import java.util.List; import static org.mockito.Mockito.mock; import static org.mockito.Mockito.when; public final class SimpleChaincodeTest { @Test public void initTest() { SimpleChaincode sc = new SimpleChaincode(); ChaincodeStub stub = mock(ChaincodeStub.class); Chaincode.Response resp = sc.init(stub); Assert.assertEquals(resp.getStatus(), Chaincode.Response.Status.SUCCESS); } @Test public void insertTest() { SimpleChaincode sc = new SimpleChaincode(); ChaincodeStub stub = mock(ChaincodeStub.class); when(stub.getFunction()).thenReturn("insert"); List<String> args = new ArrayList<>(); args.add("a"); args.add("100"); when(stub.getParameters()).thenReturn(args); Chaincode.Response resp = sc.invoke(stub); Assert.assertEquals(resp.getStatus(), Chaincode.Response.Status.SUCCESS); } @Test public void insertTooManyArgsTest() { SimpleChaincode sc = new SimpleChaincode(); ChaincodeStub stub = mock(ChaincodeStub.class); when(stub.getFunction()).thenReturn("insert"); List<String> args = new ArrayList<>(); args.add("a"); args.add("100"); args.add("b"); args.add("100"); when(stub.getParameters()).thenReturn(args); Chaincode.Response resp = sc.invoke(stub); Assert.assertEquals(resp.getMessage(), "Incorrect number of arguments. Expecting 2"); } @Test public void queryTest() { SimpleChaincode sc = new SimpleChaincode(); ChaincodeStub stub = mock(ChaincodeStub.class); when(stub.getFunction()).thenReturn("query"); List<String> args = new ArrayList<>(); args.add("a"); when(stub.getParameters()).thenReturn(args); when(stub.getStringState("a")).thenReturn("100"); Chaincode.Response resp = sc.invoke(stub); Assert.assertEquals(resp.getMessage(), "100"); } @Test public void queryNoExistTest() { SimpleChaincode sc = new SimpleChaincode(); ChaincodeStub stub = mock(ChaincodeStub.class); when(stub.getFunction()).thenReturn("query"); List<String> args = new ArrayList<>(); args.add("a"); when(stub.getParameters()).thenReturn(args); when(stub.getStringState("a")).thenReturn(null); Chaincode.Response resp = sc.invoke(stub); Assert.assertEquals(resp.getMessage(), "{\"Error\":\"Null val for a\"}"); } }
Debugging
In SimpleChaincodeTest.java, click Run Test on the left of SimpleChaincodeTest.
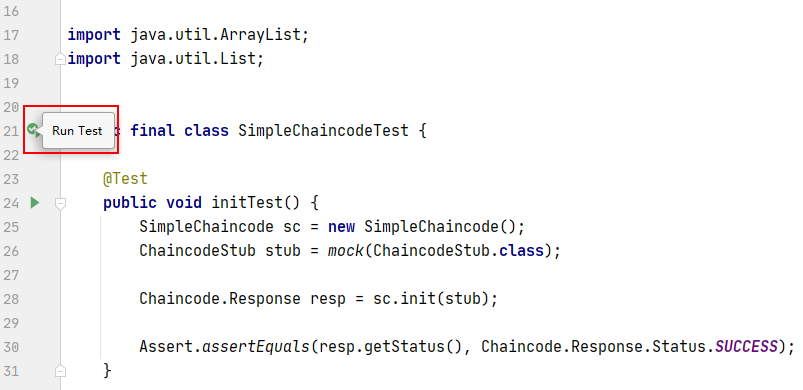
If the following information is displayed, the chaincode debugging is successful:
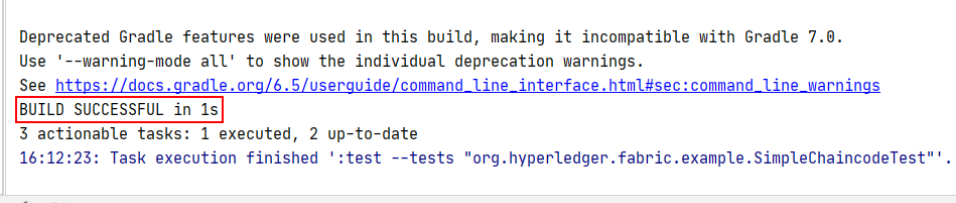
If the following information is displayed, the chaincode debugging failed. Edit the chaincode or check the logic of the test code based on the displayed information.
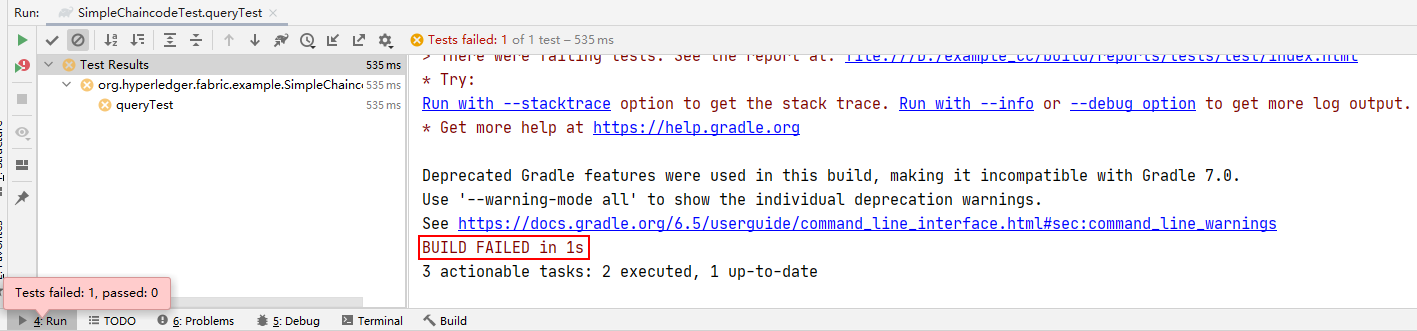
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot