Common Functions
The HCL supports various built-in functions you can call by function name for processing strings, calculating values, encrypting values, and converting types. The syntax is as follows:
<Function name>(<Argument 1>, <Argument 2>...)
This section summarizes common functions in HCL and uses examples to describe their usage. For details about the complete list of supported functions, see Terraform Functions.
String Functions
Name |
Description |
Example Value |
Output |
---|---|---|---|
format |
Produces a string by formatting a number of other values according to a specification string. |
format("Hello, %s!", "cloud") |
Hello, cloud! |
lower |
Converts all letters in the given string to lowercase. |
lower("HELLO") |
hello |
upper |
Converts all letters in the given string to uppercase. |
upper("hello") |
HELLO |
join |
Produces a string by concatenating together all elements of a given list of strings with the given delimiter. |
join(", ", ["One", "Two", "Three"]) |
One, Two, Three |
split |
Produces a list by dividing a given string at all occurrences of a given separator. |
split(", ", "One, Two, Three") |
["One", "Two", "Three"] |
substr |
Extracts a substring from a given string by offset and length. |
substr("hello world!", 1, 4) |
ello |
replace |
Searches a given string for another given substring, and replaces each occurrence with a given replacement string. |
replace("hello, cloud!", "h", "H") |
Hello, cloud! |
Numeric Functions
Name |
Description |
Example Value |
Output |
---|---|---|---|
abs |
Returns the absolute value of the given number. |
abs(-12.4) |
12.4 |
max |
Takes one or more numbers and returns the greatest number from the set. |
max(12, 54, 6) max([12, 54, 6]...) |
54 54 |
min |
Takes one or more numbers and returns the smallest number from the set. |
min(12, 54, 6) min([12, 54, 6]...) |
6 6 |
log |
Returns the logarithm of a given number in a given base. |
log(16, 2) |
4 |
power |
Calculates an exponent, by raising its first argument to the power of the second argument. |
power(3, 2) |
9 |
Collection Functions
Name |
Description |
Example Value |
Output |
---|---|---|---|
element |
Retrieves a single element from a list by an index. |
element(["One", "Two", "Three"], 1) |
Two |
index |
Finds the element index for a given value in a list. If the given value is not present in the list, an error is reported. |
index(["a", "b", "c"], "b") |
1 |
lookup |
Retrieves the value of a single element from a map, given its key. If the given key does not exist, the given default value is returned instead. |
lookup({IT="A", CT="B"}, "IT", "G") lookup({IT="A", CT="B"}, "IE", "G") |
A G |
flatten |
Replaces any elements that are lists with a flattened sequence of the list contents. |
flatten([["a", "b"], [], ["c"]]) |
["a", "b", "c"] |
keys |
Returns a list containing the keys from a map. |
keys({a=1, b=2, c=3}) |
["a", "b", "c"] |
length |
Determines the length of a given list, map, or string. |
length(["One", "Two", "Three"]) length({IT="A", CT="B"}) length("Hello, cloud!") |
3 2 13 |
Type Conversion Functions
Name |
Description |
Example Value |
Output |
---|---|---|---|
toset |
Converts a list value to a set value. |
toset(["One", "Two", "One"]) |
["One", "Two"] |
tolist |
Converts a set value to a list value. |
toset(["One", "Two", "Three"]) |
["One", "Two", "Three"] |
tonumber |
Converts a string value to a number value. |
tonumber("33") |
33 |
tostring |
Converts a number value to a string value. |
tostring(33) |
"33" |
Encoding Functions
Name |
Description |
Example Value |
Output |
---|---|---|---|
base64encode |
Encodes a UTF-8 string using Base64. |
base64encode("Hello, cloud!") |
SGVsbG8sIGNsb3VkIQ== |
base64decode |
Decodes a Base64-encoded string to its original UTF-8 string. (If the bytes after Base64 decoding are not valid UTF-8, an error is reported.) |
base64decode("SGVsbG8sIGNsb3VkIQ==") |
Hello, cloud! |
base64gzip |
Compresses a UTF-8 string with gzip and then encodes the result using Base64. |
base64gzip("Hello, cloud!") |
H4sIAAAAAAAA//JIzcnJ11FIzskvTVEEAAAA//8BAAD//wbrhYUNAAAA |
Hash and Crypto Functions
Name |
Description |
Example Value |
Output |
---|---|---|---|
sha256 |
Computes the SHA256 hash (hexadecimal) of a given string. |
sha256("Hello, cloud!") |
0ad167d1e3ac8e9f4e4f7ba83e92d0e3838177e959858631c770caaed8cc5e3a |
sha512 |
Computes the SHA512 hash (hexadecimal) of a given string. |
sha512("Hello, cloud!") |
6eb6ed9fc4edffaf90e742e7697f6cc7d8548e98aa4d5aa74982e5cdf78359e84a3ae9f226313b2dec765bf1ea4c83922dbfe4a61636d585da44ffbd7e900f56 |
base64sha256 |
Computes the SHA256 hash of a given string and encodes it using Base64. |
base64sha256("Hello, cloud!") |
CtFn0eOsjp9OT3uoPpLQ44OBd+lZhYYxx3DKrtjMXjo= |
base64sha512 |
Computes the SHA512 hash of a given string and encodes it using Base64. |
base64sha512("Hello, cloud!") |
brbtn8Tt/6+Q50LnaX9sx9hUjpiqTVqnSYLlzfeDWehKOunyJjE7Lex2W/HqTIOSLb/kphY21YXaRP+9fpAPVg== |
md5 |
Computes the MD5 hash of a given string. |
md5("hello world") |
5eb63bbbe01eeed093cb22bb8f5acdc3 |
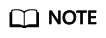
The output of base64sha512("Hello, cloud!") is not equal to that of base64encode(sha512("Hello, cloud!")), because the hexadecimal output of sha512 is Unicode-encoded in Terraform, not UTF-8.
Filesystem Functions
Name |
Description |
Example Value |
Output |
---|---|---|---|
abspath |
Converts a string containing a filesystem path to an absolute path. |
abspath("./hello.txt") |
/home/demo/test/terraform/hello.txt |
dirname |
Removes the last portion from a string containing a filesystem path. |
dirname("foo/bar/baz.txt") |
foo/bar |
basename |
Removes all except the last portion from a string containing a filesystem path. |
basename("foo/bar/baz.txt") |
baz.txt |
file |
Reads the contents of a file at the given path and returns them as a string. |
file("./hello.txt") |
Hello, cloud! |
filebase64 |
Reads the contents of a file at the given path and returns them as a Base64-encoded string. |
filebase64("./hello.txt") |
SGVsbG8sIGNsb3VkIQ== |
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot