Customizing the Bottom Menu Bar
You can add, delete, and customize menu items on the bottom menu bar of a meeting screen.
There are two types of bottom menu bars and customization methods:
1. ConfAudioToolbar: customizes the bottom menu bar for voice meetings (see Customizing a Menu for Voice Meetings).
2. ConfVideoToolbar: customizes the bottom menu bar for video meetings (see Customizing a Menu for Video Meetings).
Application Scenarios
Menu items on the bottom menu bar need to be adjusted.
Precautions
1. sdkConfig takes effect only when this configuration is passed during SDK initialization.
Customizing Menu Settings
Sample Code
1 2 3 4 |
ToolBarMenuProxy toolBarMenuProxy = new ToolBarMenuProxy(); toolBarMenuProxy.setConfAudioToolbarHandle(new CustomConfAudioToolbarHandle()); toolBarMenuProxy.setConfVideoToolbarHandle(new CustomConfVideoToolbarHandle()); sdkConfig.setToolBarMenuProxy(toolBarMenuProxy); |
Customizing a Menu for Voice Meetings


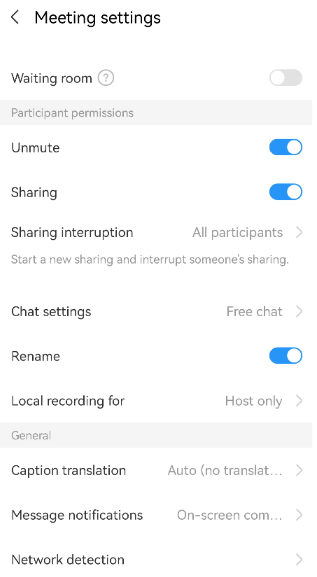
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 |
/** * Demo of customizing a toolbar for voice meetings */ public class CustomConfAudioToolbarHandle implements IToolbarMenuStrategy { @Override public List<IConfMenu> buildMenuItems() { List<IConfMenu> confMenus = new ArrayList<>(); confMenus.add(new MicMenu()); confMenus.add(new SpeakerMenu()); confMenus.add(new ParticipantMenu()); // [Demo] Add a custom menu. confMenus.add(new CustomMenu()); confMenus.add(new MoreMenu()); return confMenus; } @Override public List<IConfMenu> buildMoreMenuItems() { List<IConfMenu> confMenus = new ArrayList<>(); confMenus.add(new ConnectAudioMenu()); confMenus.add(new DisconnectAudioMenu()); confMenus.add(new ChatMenu()); confMenus.add(new InterpretMenu()); confMenus.add(new HandsUpOrDownMenu()); confMenus.add(new InviteMoreMenu()); confMenus.add(new OpenOrCloseRecordMenu()); confMenus.add(new SubtitlesMenu()); confMenus.add(new CloudLiveMenu()); confMenus.add(new VoteMenu()); confMenus.add(new ConfSettingMenu()); // [Demo] Add a custom menu. confMenus.add(new CustomMenu()); return confMenus; } @Override public List<IConfMenu> buildSettingMenuItems() { List<IConfMenu> menus = new ArrayList<>(); List<IConfMenu> audioVideomenuChildren = new ArrayList<>(); IConfMenu howlDetectMenu = new SwitchMenu(R.id.hwmconf_confsetting_whistle_detection, R.string.hwmconf_mine_calling_setting_howl_auto_mute, true); IConfMenu noiseReductionMenu = new SwitchMenu(R.id.hwmconf_confsetting_noise_reduction, R.string.hwmconf_audio_noise_reduction, true); audioVideomenuChildren.add(howlDetectMenu); audioVideomenuChildren.add(noiseReductionMenu); MenuContainer audioVideoMenuContainer = new MenuContainer(R.id.hwmconf_setting_menu_audio_video, R.string.hwmconf_setting_audio_video, audioVideomenuChildren); List<IConfMenu> securityMenuChildren = new ArrayList<>(); // Lock the meeting. IConfMenu lockConfMenu = new SwitchMenu(R.id.hwmconf_confsetting_lock_meeting, R.string.hwmconf_lock, false); securityMenuChildren.add(lockConfMenu); // Set users who can join the meeting. IConfMenu allowJoinConfMenu = new TextMenu(R.id.hwmconf_confsetting_allow_join_conf, R.string.hwmconf_allow_incoming_call, R.string.hwmconf_everyone); securityMenuChildren.add(allowJoinConfMenu); // Enable the waiting room. IConfMenu enableWaitingRoomMenu = new SwitchMenu(R.id.hwmconf_confsetting_enable_waiting_room, R.string.hwmconf_enable_waiting_room, false); securityMenuChildren.add(enableWaitingRoomMenu); MenuContainer securityMenuContainer = new MenuContainer(R.id.hwmconf_setting_menu_security, R.string.hwmconf_setting_safe, securityMenuChildren); List<IConfMenu> attendeePermissionMenuChildren = new ArrayList<>(); // Allow unmuting. IConfMenu allowReleaseMuteMenu = new SwitchMenu(R.id.hwmconf_confsetting_allow_unmute, R.string.hwmconf_allow_ummute_self, false); IConfMenu allowShareMuteMenu = new SwitchMenu(R.id.hwmconf_confsetting_allow_sharing, R.string.hwmconf_allow_sharing, false); // Set the sharing interruption permission. IConfMenu preemptShareTypeMenu = new TextMenu(R.id.hwmconf_confsetting_preempt_share_type, R.string.hwmconf_preempt_share_permission, R.string.hwmconf_preempt_share_only_host); // Set the chat permission. IConfMenu chatPermissionMenu = new TextMenu(R.id.hwmconf_confsetting_chat_permission, R.string.hwmconf_chat_settings, R.string.hwmconf_private_chat_free); // Allow renaming. IConfMenu allowRenameMenu = new SwitchMenu(R.id.hwmconf_confsetting_allow_rename, R.string.hwmconf_set_allow_rename, true); IConfMenu allowLocalRecordMenu = new RouterMenu(R.id.hwmconf_confsetting_local_record_permission, R.string.hwmconf_allow_record, ""); attendeePermissionMenuChildren.add(allowReleaseMuteMenu); attendeePermissionMenuChildren.add(allowShareMuteMenu); attendeePermissionMenuChildren.add(preemptShareTypeMenu); attendeePermissionMenuChildren.add(chatPermissionMenu); attendeePermissionMenuChildren.add(allowRenameMenu); attendeePermissionMenuChildren.add(allowLocalRecordMenu); MenuContainer attendeePermissionMenuContainer = new MenuContainer(R.id.hwmconf_setting_menu_paticipant_permission, R.string.hwmconf_setting_participant_permission, attendeePermissionMenuChildren); List<IConfMenu> commonMenuChildren = new ArrayList<>(); // Set the caption translation language. IConfMenu subtitlesLanguageMenu = new TextMenu(R.id.hwmconf_confsetting_subtitles_language, R.string.hwmconf_subtitle_translation_language, R.string.hwmconf_auto_no_translation); commonMenuChildren.add(subtitlesLanguageMenu); // Configure chat message notification. IConfMenu chatMessageRemindMenu = new TextMenu(R.id.hwmconf_confsetting_chat_message_remind, R.string.hwmconf_message_notify_mode, R.string.hwmconf_bubble_message_mode); commonMenuChildren.add(chatMessageRemindMenu); // Check the network quality. IConfMenu networkDetectMenu = new RouterMenu(R.id.hwmconf_confsetting_network_detect, R.string.hwmconf_network_check, "cloudlink://hwmeeting/networkdetection"); commonMenuChildren.add(networkDetectMenu); MenuContainer commonMenuContainer = new MenuContainer(R.id.hwmconf_setting_menu_common, R.string.hwmconf_setting_common, commonMenuChildren); menus.add(audioVideoMenuContainer); menus.add(securityMenuContainer); menus.add(attendeePermissionMenuContainer); menus.add(commonMenuContainer); return menus; } } |
Customizing a Menu for Video Meetings



Sample Code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 |
/** * Demo of customizing a toolbar for video meetings */ public class CustomConfVideoToolbarHandle implements IToolbarMenuStrategy { @Override public List<IConfMenu> buildMenuItems() { List<IConfMenu> confMenus = new ArrayList<>(); confMenus.add(new MicMenu()); confMenus.add(new CameraMenu()); confMenus.add(new ParticipantMenu()); // [Demo] Add a custom menu. confMenus.add(new CustomMenu()); confMenus.add(new MoreMenu()); return confMenus; } @Override public List<IConfMenu> buildMoreMenuItems() { List<IConfMenu> confMenus = new ArrayList<>(); confMenus.add(new ConnectAudioMenu()); confMenus.add(new DisconnectAudioMenu()); confMenus.add(new ChatMenu()); confMenus.add(new InterpretMenu()); confMenus.add(new HandsUpOrDownMenu()); confMenus.add(new InviteMoreMenu()); confMenus.add(new OpenOrCloseRecordMenu()); confMenus.add(new SubtitlesMenu()); confMenus.add(new CloudLiveMenu()); confMenus.add(new SwitchCameraMenu()); confMenus.add(new VirtualBackgroundMenu()); confMenus.add(new VoteMenu()); confMenus.add(new ConfSettingMenu()); // [Demo] Add a custom menu for disabling voice prompts. confMenus.add(new CustomDisableVoicePromptsMenu()); // [Demo] Add a custom menu. confMenus.add(new CustomMenu()); return confMenus; } @Override public List<IConfMenu> buildSettingMenuItems() { List<IConfMenu> menus = new ArrayList<>(); List<IConfMenu> audioVideomenuChildren = new ArrayList<>(); IConfMenu howlDetectMenu = new SwitchMenu(R.id.hwmconf_confsetting_whistle_detection, R.string.hwmconf_mine_calling_setting_howl_auto_mute, true); IConfMenu beautyMenu = new SwitchMenu(R.id.hwmconf_confsetting_beauty, R.string.hwmconf_setting_beauty, true); IConfMenu mirrorMenu = new SwitchMenu(R.id.hwmconf_confsetting_video_mirror, R.string.hwmconf_video_mirroring, true); IConfMenu pipMenu = new SwitchMenu(R.id.hwmconf_confsetting_hide_self, R.string.hwmconf_setting_pip, true); IConfMenu directionMenu = new RouterMenu(R.id.hwmconf_confsetting_camera_direction, R.string.hwmconf_auto, "cloudlink://hwmeeting/cameradirection"); IConfMenu noiseReductionMenu = new SwitchMenu(R.id.hwmconf_confsetting_noise_reduction, R.string.hwmconf_audio_noise_reduction, true); IConfMenu highResMenu = new SwitchMenu(R.id.hwmconf_confsetting_high_resolution, R.string.hwmconf_mine_calling_setting_high_resolution, true); IConfMenu enableBrightenMenu = new SwitchMenu(R.id.hwmconf_confsetting_enable_brighten, R.string.hwmconf_enhance_video_quality, false); IConfMenu pictureRatioMenu = new TextMenu(R.id.hwmconf_confsetting_picture_ratio, R.string.hwmconf_picture_ratio, R.string.hwmconf_picture_ratio_original_size); audioVideomenuChildren.add(directionMenu); audioVideomenuChildren.add(howlDetectMenu); audioVideomenuChildren.add(beautyMenu); audioVideomenuChildren.add(mirrorMenu); audioVideomenuChildren.add(pipMenu); audioVideomenuChildren.add(noiseReductionMenu); audioVideomenuChildren.add(highResMenu); audioVideomenuChildren.add(enableBrightenMenu); audioVideomenuChildren.add(pictureRatioMenu); MenuContainer audioVideoMenuContainer = new MenuContainer(R.id.hwmconf_setting_menu_audio_video, R.string.hwmconf_setting_audio_video, audioVideomenuChildren); List<IConfMenu> securityMenuChildren = new ArrayList<>(); // Lock the meeting. IConfMenu lockConfMenu = new SwitchMenu(R.id.hwmconf_confsetting_lock_meeting, R.string.hwmconf_lock, false); securityMenuChildren.add(lockConfMenu); // Set users who can join the meeting. IConfMenu allowJoinConfMenu = new TextMenu(R.id.hwmconf_confsetting_allow_join_conf, R.string.hwmconf_allow_incoming_call, R.string.hwmconf_everyone); securityMenuChildren.add(allowJoinConfMenu); IConfMenu enableWaitingRoomMenu = new SwitchMenu(R.id.hwmconf_confsetting_enable_waiting_room, R.string.hwmconf_enable_waiting_room, false); securityMenuChildren.add(enableWaitingRoomMenu); MenuContainer securityMenuContainer = new MenuContainer(R.id.hwmconf_setting_menu_security, R.string.hwmconf_setting_safe, securityMenuChildren); List<IConfMenu> attendeePermissionMenuChildren = new ArrayList<>(); // Allow unmuting. IConfMenu allowReleaseMuteMenu = new SwitchMenu(R.id.hwmconf_confsetting_allow_unmute, R.string.hwmconf_allow_ummute_self, false); // Allow participants to enable the camera. IConfMenu cameraRestrictionSwitch = new SwitchMenu(R.id.hwmconf_confsetting_allow_open_camera, R.string.hwmconf_allow_open_video, false); IConfMenu cameraRestrictionMenu = new RouterMenu(R.id.hwmconf_confsetting_camera_restriction, R.string.hwmconf_camera_restriction, ""); IConfMenu allowShareMuteMenu = new SwitchMenu(R.id.hwmconf_confsetting_allow_sharing, R.string.hwmconf_allow_sharing, false); // Set the sharing interruption permission. IConfMenu preemptShareTypeMenu = new TextMenu(R.id.hwmconf_confsetting_preempt_share_type, R.string.hwmconf_preempt_share_permission, R.string.hwmconf_preempt_share_only_host); // Set the chat permission. IConfMenu chatPermissionMenu = new TextMenu(R.id.hwmconf_confsetting_chat_permission, R.string.hwmconf_chat_settings, R.string.hwmconf_private_chat_free); // Allow renaming. IConfMenu allowRenameMenu = new SwitchMenu(R.id.hwmconf_confsetting_allow_rename, R.string.hwmconf_set_allow_rename, true); IConfMenu allowLocalRecordMenu = new RouterMenu(R.id.hwmconf_confsetting_local_record_permission, R.string.hwmconf_allow_record, ""); attendeePermissionMenuChildren.add(allowReleaseMuteMenu); attendeePermissionMenuChildren.add(cameraRestrictionSwitch); attendeePermissionMenuChildren.add(cameraRestrictionMenu); attendeePermissionMenuChildren.add(allowShareMuteMenu); attendeePermissionMenuChildren.add(preemptShareTypeMenu); attendeePermissionMenuChildren.add(chatPermissionMenu); attendeePermissionMenuChildren.add(allowRenameMenu); attendeePermissionMenuChildren.add(allowLocalRecordMenu); /* * [Demo] Add a setting item to Meeting settings. */ IConfMenu customeSettingMenuTest1 = new SwitchMenu(R.id.conf_setting_menu_test1, R.string.menu_conf_setting_menu_test1, false); attendeePermissionMenuChildren.add(customeSettingMenuTest1); MenuContainer attendeePermissionMenuContainer = new MenuContainer(R.id.hwmconf_setting_menu_paticipant_permission, R.string.hwmconf_setting_participant_permission, attendeePermissionMenuChildren); List<IConfMenu> commonMenuChildren = new ArrayList<>(); // Set the caption translation language. IConfMenu subtitlesLanguageMenu = new TextMenu(R.id.hwmconf_confsetting_subtitles_language, R.string.hwmconf_subtitle_translation_language, R.string.hwmconf_auto_no_translation); commonMenuChildren.add(subtitlesLanguageMenu); // Configure chat message notification. IConfMenu chatMessageRemindMenu = new TextMenu(R.id.hwmconf_confsetting_chat_message_remind, R.string.hwmconf_message_notify_mode, R.string.hwmconf_bubble_message_mode); commonMenuChildren.add(chatMessageRemindMenu); // Check the network quality. IConfMenu networkDetectMenu = new RouterMenu(R.id.hwmconf_confsetting_network_detect, R.string.hwmconf_network_check, "cloudlink://hwmeeting/networkdetection"); commonMenuChildren.add(networkDetectMenu); MenuContainer commonMenuContainer = new MenuContainer(R.id.hwmconf_setting_menu_common, R.string.hwmconf_setting_common, commonMenuChildren); menus.add(audioVideoMenuContainer); menus.add(securityMenuContainer); menus.add(attendeePermissionMenuContainer); menus.add(commonMenuContainer); /* * [Demo] Add a group of setting items to Meeting settings. */ List<IConfMenu> customChildren = new ArrayList<>(); // Check the network quality. IConfMenu customSettingMenuTest2 = new RouterMenu(R.id.conf_setting_menu_test2, R.string.menu_conf_setting_menu_test1, "test"); customChildren.add(customSettingMenuTest2); MenuContainer customContainer = new MenuContainer(R.id.conf_setting_menu_test_group, R.string.menu_conf_setting_menu_test_group, customChildren); menus.add(customContainer); return menus; } } |
Customizing a Menu for Webinars

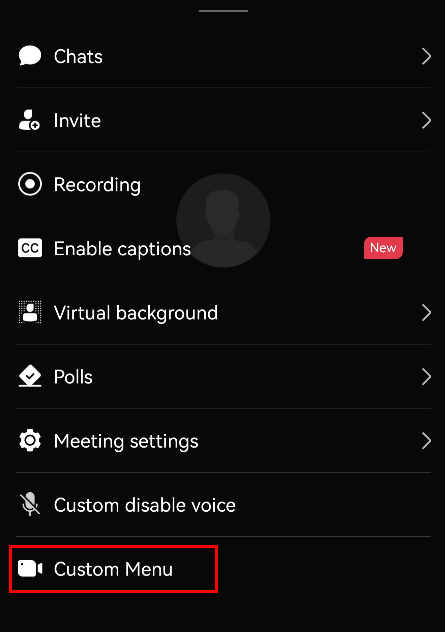
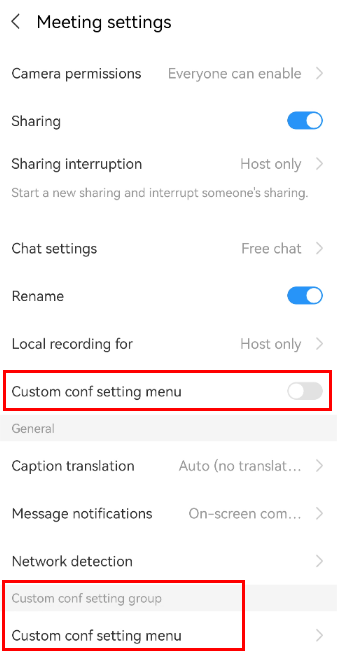
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 |
/** * Demo of customizing a toolbar for webinars */ public class CustomConfWebinarToolbarHandle implements IToolbarMenuStrategy { @Override public List<IConfMenu> buildMenuItems() { if (!NativeSDK.getConfMgrApi().isInConf()) { return buildAllMenuItems(); } if (NativeSDK.getConfStateApi().getSelfRole() == ConfRole.ROLE_AUDIENCE) { return buildAudienceMenuItems(); } if (NativeSDK.getConfStateApi().getSelfRole() == ConfRole.ROLE_HOST) { return buildHostMenuItems(); } return buildAttendeeMenuItems(); } private List<IConfMenu> buildAllMenuItems() { List<IConfMenu> confMenus = new ArrayList<>(); confMenus.add(new MicMenu()); confMenus.add(new CameraMenu()); if (TupConfig.isNeedConfChat()) { confMenus.add(new ChatMenu()); } confMenus.add(new HandsMenu()); if (TupConfig.isNeedScreenShare() && CpuLevelUtil.isHigherThan(CpuLevel.CALL_CPU_LEVEL_SUPER_LOW)) { confMenus.add(new ShareMenu()); } if (ConfUIConfig.getInstance().isAudience()) { confMenus.add(new VoteBtnMenu()); } confMenus.add(new AudienceJoinMenu()); confMenus.add(new ParticipantMenu()); confMenus.add(new MoreMenu()); return confMenus; } private List<IConfMenu> buildAudienceMenuItems() { List<IConfMenu> confMenus = new ArrayList<>(); confMenus.add(new MicMenu()); if (TupConfig.isNeedConfChat()) { confMenus.add(new ChatMenu()); } confMenus.add(new HandsMenu()); if (ConfUIConfig.getInstance().isAudience()) { confMenus.add(new VoteBtnMenu()); } confMenus.add(new MoreMenu()); return confMenus; } private List<IConfMenu> buildAttendeeMenuItems() { List<IConfMenu> confMenus = new ArrayList<>(); confMenus.add(new MicMenu()); confMenus.add(new CameraMenu()); if (TupConfig.isNeedScreenShare() && CpuLevelUtil.isHigherThan(CpuLevel.CALL_CPU_LEVEL_SUPER_LOW)) { confMenus.add(new ShareMenu()); } confMenus.add(new ParticipantMenu()); if (ConfUIConfig.getInstance().isAudience()) { confMenus.add(new VoteBtnMenu()); } confMenus.add(new MoreMenu()); return confMenus; } private List<IConfMenu> buildHostMenuItems() { List<IConfMenu> confMenus = new ArrayList<>(); confMenus.add(new MicMenu()); confMenus.add(new CameraMenu()); confMenus.add(new AudienceJoinMenu()); confMenus.add(new ParticipantMenu()); confMenus.add(new MoreMenu()); return confMenus; } @Override public List<IConfMenu> buildMoreMenuItems() { List<IConfMenu> confMenus = new ArrayList<>(); confMenus.add(new ConnectAudioMenu()); confMenus.add(new DisconnectAudioMenu()); confMenus.add(new RenameMenu()); confMenus.add(new StartOrStopShareMenu()); confMenus.add(new ChatMenu()); confMenus.add(new ShareConfLinkMenu()); confMenus.add(new HandsUpOrDownMenu()); confMenus.add(new InviteMoreMenu()); confMenus.add(new OpenOrCloseRecordMenu()); confMenus.add(new SubtitlesMenu()); confMenus.add(new CloudLiveMenu()); confMenus.add(new SwitchCameraMenu()); confMenus.add(new VirtualBackgroundMenu()); confMenus.add(new VoteMenu()); confMenus.add(new ConfSettingMenu()); // [Demo] Add a custom menu. confMenus.add(new CustomMenu()); return confMenus; } @Override public List<IConfMenu> buildSettingMenuItems() { List<IConfMenu> menus = new ArrayList<>(); List<IConfMenu> audioVideomenuChildren = new ArrayList<>(); IConfMenu howlDetectMenu = new SwitchMenu(R.id.hwmconf_confsetting_whistle_detection, com.huawei.hwmdemo.R.string.hwmconf_mine_calling_setting_howl_auto_mute, true); IConfMenu beautyMenu = new SwitchMenu(R.id.hwmconf_confsetting_beauty, com.huawei.hwmdemo.R.string.hwmconf_setting_beauty, true); IConfMenu mirrorMenu = new SwitchMenu(R.id.hwmconf_confsetting_video_mirror, R.string.hwmconf_video_mirroring, true); IConfMenu pipMenu = new SwitchMenu(R.id.hwmconf_confsetting_hide_self, com.huawei.hwmdemo.R.string.hwmconf_setting_pip, true); IConfMenu directionMenu = new RouterMenu(R.id.hwmconf_confsetting_camera_direction, R.string.hwmconf_auto, "cloudlink://hwmeeting/cameradirection"); IConfMenu noiseReductionMenu = new SwitchMenu(R.id.hwmconf_confsetting_noise_reduction, R.string.hwmconf_audio_noise_reduction, true); IConfMenu highResMenu = new SwitchMenu(R.id.hwmconf_confsetting_high_resolution, R.string.hwmconf_mine_calling_setting_high_resolution, true); IConfMenu enableBrightenMenu = new SwitchMenu(R.id.hwmconf_confsetting_enable_brighten, R.string.hwmconf_enhance_video_quality, false); IConfMenu pictureRatioMenu = new TextMenu(R.id.hwmconf_confsetting_picture_ratio, R.string.hwmconf_picture_ratio, R.string.hwmconf_picture_ratio_original_size); audioVideomenuChildren.add(howlDetectMenu); audioVideomenuChildren.add(beautyMenu); audioVideomenuChildren.add(mirrorMenu); audioVideomenuChildren.add(pipMenu); audioVideomenuChildren.add(directionMenu); audioVideomenuChildren.add(noiseReductionMenu); audioVideomenuChildren.add(highResMenu); audioVideomenuChildren.add(enableBrightenMenu); audioVideomenuChildren.add(pictureRatioMenu); MenuContainer audioVideoMenuContainer = new MenuContainer(R.id.hwmconf_setting_menu_audio_video, com.huawei.hwmdemo.R.string.hwmconf_setting_audio_video, audioVideomenuChildren); List<IConfMenu> securityMenuChildren = new ArrayList<>(); // Lock the meeting. IConfMenu lockConfMenu = new SwitchMenu(R.id.hwmconf_confsetting_lock_meeting, com.huawei.hwmdemo.R.string.hwmconf_lock, false); securityMenuChildren.add(lockConfMenu); // Set users who can join the meeting. IConfMenu allowJoinConfMenu = new TextMenu(R.id.hwmconf_confsetting_allow_join_conf, R.string.hwmconf_allow_incoming_call, R.string.hwmconf_everyone); securityMenuChildren.add(allowJoinConfMenu); // Enable the waiting room. IConfMenu enableWaitingRoomMenu = new SwitchMenu(R.id.hwmconf_confsetting_enable_waiting_room, R.string.hwmconf_enable_waiting_room, false); securityMenuChildren.add(enableWaitingRoomMenu); MenuContainer securityMenuContainer = new MenuContainer(R.id.hwmconf_setting_menu_security, com.huawei.hwmdemo.R.string.hwmconf_setting_safe, securityMenuChildren); List<IConfMenu> attendeePermissionMenuChildren = new ArrayList<>(); // Allow unmuting. IConfMenu allowReleaseMuteMenu = new SwitchMenu(R.id.hwmconf_confsetting_allow_unmute, com.huawei.hwmdemo.R.string.hwmconf_allow_ummute_self, false); // Allow participants to enable the camera. IConfMenu cameraRestrictionSwitch = new SwitchMenu(R.id.hwmconf_confsetting_allow_open_camera, R.string.hwmconf_allow_open_video, false); IConfMenu cameraRestrictionMenu = new RouterMenu(R.id.hwmconf_confsetting_camera_restriction, R.string.hwmconf_camera_restriction, ""); IConfMenu allowShareMuteMenu = new SwitchMenu(R.id.hwmconf_confsetting_allow_sharing, com.huawei.hwmdemo.R.string.hwmconf_allow_sharing, false); // Set the sharing interruption permission. IConfMenu preemptShareTypeMenu = new TextMenu(R.id.hwmconf_confsetting_preempt_share_type, R.string.hwmconf_preempt_share_permission, R.string.hwmconf_preempt_share_only_host); // Set the chat permission. IConfMenu chatPermissionMenu = new TextMenu(R.id.hwmconf_confsetting_chat_permission, R.string.hwmconf_chat_settings, R.string.hwmconf_private_chat_free);// Allow renaming. // Allow renaming. IConfMenu allowRenameMenu = new SwitchMenu(R.id.hwmconf_confsetting_allow_rename, com.huawei.hwmdemo.R.string.hwmconf_set_allow_rename, true); IConfMenu allowLocalRecordMenu = new RouterMenu(R.id.hwmconf_confsetting_local_record_permission, R.string.hwmconf_allow_record, ""); attendeePermissionMenuChildren.add(allowReleaseMuteMenu); attendeePermissionMenuChildren.add(cameraRestrictionSwitch); attendeePermissionMenuChildren.add(cameraRestrictionMenu); attendeePermissionMenuChildren.add(allowShareMuteMenu); attendeePermissionMenuChildren.add(preemptShareTypeMenu); attendeePermissionMenuChildren.add(chatPermissionMenu); attendeePermissionMenuChildren.add(allowRenameMenu); attendeePermissionMenuChildren.add(allowLocalRecordMenu); /* * [Demo] Add a setting item to Meeting settings. */ IConfMenu customeSettingMenuTest1 = new SwitchMenu(com.huawei.hwmdemo.R.id.conf_setting_menu_test1, com.huawei.hwmdemo.R.string.menu_conf_setting_menu_test1, false); attendeePermissionMenuChildren.add(customeSettingMenuTest1); MenuContainer attendeePermissionMenuContainer = new MenuContainer(R.id.hwmconf_setting_menu_paticipant_permission, com.huawei.hwmdemo.R.string.hwmconf_setting_participant_permission, attendeePermissionMenuChildren); List<IConfMenu> commonMenuChildren = new ArrayList<>(); // Set the caption translation language. IConfMenu subtitlesLanguageMenu = new TextMenu(R.id.hwmconf_confsetting_subtitles_language, R.string.hwmconf_subtitle_translation_language, R.string.hwmconf_auto_no_translation); commonMenuChildren.add(subtitlesLanguageMenu); // Configure chat message notification. IConfMenu chatMessageRemindMenu = new TextMenu(R.id.hwmconf_confsetting_chat_message_remind, R.string.hwmconf_message_notify_mode, R.string.hwmconf_bubble_message_mode); commonMenuChildren.add(chatMessageRemindMenu); // Check the network quality. IConfMenu networkDetectMenu = new RouterMenu(R.id.hwmconf_confsetting_network_detect, com.huawei.hwmdemo.R.string.hwmconf_network_check, "cloudlink://hwmeeting/networkdetection"); commonMenuChildren.add(networkDetectMenu); MenuContainer commonMenuContainer = new MenuContainer(R.id.hwmconf_setting_menu_common, com.huawei.hwmdemo.R.string.hwmconf_setting_common, commonMenuChildren); menus.add(audioVideoMenuContainer); menus.add(securityMenuContainer); menus.add(attendeePermissionMenuContainer); menus.add(commonMenuContainer); /* * [Demo] Add a group of setting items to Meeting settings. */ List<IConfMenu> customChildren = new ArrayList<>(); // Check the network quality. IConfMenu customeSettingMenuTest2 = new RouterMenu(com.huawei.hwmdemo.R.id.conf_setting_menu_test2, com.huawei.hwmdemo.R.string.menu_conf_setting_menu_test1, "test"); customChildren.add(customeSettingMenuTest2); MenuContainer customContainer = new MenuContainer(com.huawei.hwmdemo.R.id.conf_setting_menu_test_group, com.huawei.hwmdemo.R.string.menu_conf_setting_menu_test_group, customChildren); menus.add(customContainer); return menus; } } |
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot