Using the Kubernetes Java SDK to Access CCI
This section describes how to use cci-iam-authenticator (the CCI authentication tool) and kubernetes-client/java to call the APIs.
Installing cci-iam-authenticator
Download, install, and set cci-iam-authenticator by referring to Using Native kubectl (Recommended).
Installing kubernetes-client/java
For details, see Installation.
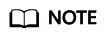
The Kubernetes version corresponding to the CCI open APIs is 1.19. According to Versioning and Compatibility, the recommended SDK version is 11.0.2 or later.
If you want to use GenericKubernetesClient (see Code Examples), the version must be 9.0.0 or later.
Using the Java SDK
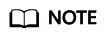
The examples have been tested for the following versions:
- 11.0.2
Add the following dependencies to the project's POM file:
<dependency> <groupId>io.kubernetes</groupId> <artifactId>client-java</artifactId> <version>11.0.2</version> </dependency>
The following example shows how to use the kubeconfig file to create ApiClient. For details, see the generate-kubeconfig subcommand in cci-iam-authenticator Usage Reference.
public class CommonCases { // ... public static void main(String[] args) throws IOException, ApiException, InterruptedException { // file path to your KubeConfig String kubeConfigPath = "<path to kubeconfig>"; // loading the out-of-cluster config, a kubeconfig from file-system File file = new File(kubeConfigPath); KubeConfig config = KubeConfig.loadKubeConfig(new FileReader(file)); config.setFile(file); ApiClient client = buildClient(config).build(); // ... } public static ClientBuilder buildClient(KubeConfig config) throws IOException { final ClientBuilder builder = new ClientBuilder(); String server = config.getServer(); if (!server.contains("://")) { if (server.contains(":443")) { server = "https://" + server; } else { server = "http://" + server; } } builder.setVerifyingSsl(config.verifySSL()); builder.setBasePath(server); builder.setAuthentication(new CCIKubeconfigAuthentication(config)); return builder; } }
CCIKubeconfigAuthentication.java
package com.huawei.demos; import io.kubernetes.client.openapi.ApiClient; import io.kubernetes.client.util.KubeConfig; import io.kubernetes.client.util.credentials.Authentication; import okhttp3.Interceptor; import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.Response; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import java.io.IOException; import java.time.Instant; /** * Uses a {@link KubeConfig} to configure {@link ApiClient} authentication to the CCI Kubernetes API. * * <p> Only try to use AccessTokenAuthentication mechanisms, which is enough for CCI. */ public class CCIKubeconfigAuthentication implements Authentication, Interceptor { private static final Logger log = LoggerFactory.getLogger(CCIKubeconfigAuthentication.class); private final KubeConfig config; private String token; private Instant expiry; public CCIKubeconfigAuthentication(final KubeConfig config) throws IOException { this.config = config; this.expiry = Instant.MIN; } private String getToken() { // get access token every 600 seconds if (Instant.now().isAfter(this.expiry)) { log.debug("Token expired, get new one from kubeconfig"); this.token = config.getAccessToken(); if (this.token != null) { this.expiry = Instant.now().plusSeconds(600); } } return this.token; } @Override public void provide(ApiClient client) { OkHttpClient httpClient = client.getHttpClient().newBuilder().addInterceptor(this).build(); client.setHttpClient(httpClient); } @Override public Response intercept(Interceptor.Chain chain) throws IOException { Request request = chain.request(); Request authRequest; authRequest = request.newBuilder().header("Authorization", "Bearer " + getToken()).build(); return chain.proceed(authRequest); } }
Accessing CCI:
public class CommonCases { // ... private static void createNamespace(CoreV1Api api) throws ApiException { String enableK8sRbac = "false"; String flavor = "general-computing"; String warmPoolSize = "10"; Map<String, String> labels = new HashMap<>(); labels.put("rbac.authorization.cci.io/enable-k8s-rbac", enableK8sRbac); Map<String, String> annotations = new HashMap<>(); annotations.put("namespace.kubernetes.io/flavor", flavor); annotations.put("network.cci.io/warm-pool-size", warmPoolSize); V1Namespace namespace = new V1Namespace() .metadata(new V1ObjectMeta().name(NAMESPACE).labels(labels).annotations(annotations)); LOGGER.info("start to create namespace {}", NAMESPACE); api.createNamespace(namespace, null, null, null); LOGGER.info("namespace created"); } private static void createNetwork(GenericKubernetesApi<Network, NetworkList> networkApi) throws ApiException { String name = NETWORK; String projectID = "<Account ID, which can be obtained from My Credentials>"; String domainID = "<Project ID, which can be obtained from My Credentials>"; String securityGroupID = "<Security group ID, which can be obtained from the Security Groups page of the Network Console>"; String availableZone = "<AZ name, for example, cn-north-1a, cn-north-4a, or cn-east-3a>"; String vpcID = "VPC ID, which can be obtained from the VPC console"; String cidr = "<Subnet CIDR block, for example, 192.168.128.0/18>"; String networkID = "<Subnet network ID, which can be obtained from the Subnets page of the VPC console>"; String subnetID = "<Subnet ID, which can be obtained from the Subnets page of the VPC console>"; String networkType = "underlay_neutron"; Map<String, String> annotations = new HashMap<>(); annotations.put("network.alpha.kubernetes.io/default-security-group", securityGroupID); annotations.put("network.alpha.kubernetes.io/domain-id", domainID); annotations.put("network.alpha.kubernetes.io/project-id", projectID); Network network = new Network() .metadata(new V1ObjectMeta().name(name).namespace(NAMESPACE).annotations(annotations)) .spec(new NetworkSpec() .availableZone(availableZone) .cidr(cidr) .attachedVPC(vpcID) .networkID(networkID) .networkType(networkType) .subnetID(subnetID)); LOGGER.info("start to create network {}/{}", NAMESPACE, name); networkApi.create(network).throwsApiException(); LOGGER.info("network created"); } private static void waitNamespaceActive(CoreV1Api api) throws ApiException, InterruptedException { for (int i = 0; i < 5; i++) { V1Namespace ns = api.readNamespace(NAMESPACE, null, null, null); if (ns.getStatus() != null && NAMESPACE_ACTIVE.equals(ns.getStatus().getPhase())) { return; } Thread.sleep(WAIT_ACTIVE_MILLIS); } throw new IllegalStateException("namespace not active"); } private static void waitNetworkActive(GenericKubernetesApi<Network, NetworkList> networkApi) throws ApiException, InterruptedException { for (int i = 0; i < 5; i++) { Network network = networkApi.get(NAMESPACE, NETWORK).throwsApiException().getObject(); if (network.getStatus() != null && NETWORK_ACTIVE.equals(network.getStatus().getState())) { return; } Thread.sleep(WAIT_ACTIVE_MILLIS); } throw new IllegalStateException("network not active"); } private static void createDeployment(AppsV1Api api) throws ApiException { String app = APP; String cpu = "500m"; String memory = "1024Mi"; String containerName = "container-0"; String image = "library/nginx:stable-alpine-perl"; Map<String, Quantity> limits = new HashMap<>(); limits.put("cpu", Quantity.fromString(cpu)); limits.put("memory", Quantity.fromString(memory)); V1Container container = new V1Container() .name(containerName) .image(image) .resources(new V1ResourceRequirements().limits(limits).requests(limits)); Map<String, String> labels = new HashMap<>(); labels.put("app", app); V1PodTemplateSpec podTemplateSpec = new V1PodTemplateSpec() .spec(new V1PodSpec() .priority(0) .imagePullSecrets(Collections.singletonList(new V1LocalObjectReference().name("imagepull-secret"))) .containers(Collections.singletonList(container))) .metadata(new V1ObjectMeta().labels(labels)); V1Deployment deployment = new V1Deployment() .metadata(new V1ObjectMeta().name(app)) .spec(new V1DeploymentSpec() .replicas(2) .selector(new V1LabelSelector().matchLabels(labels)) .template(podTemplateSpec)); LOGGER.info("start to create deployment {}/{}", NAMESPACE, APP); api.createNamespacedDeployment(NAMESPACE, deployment, null, null, null); LOGGER.info("deployment created"); } private static void getDeployment(AppsV1Api api) throws ApiException { V1Deployment deployment = api.readNamespacedDeployment(APP, NAMESPACE, null, null, null); LOGGER.info("deployment metadata: {}", deployment.getMetadata()); } private static void deleteDeployment(AppsV1Api api) throws ApiException { LOGGER.info("start to delete deployment"); api.deleteNamespacedDeployment(APP, NAMESPACE, null, null, null, null, null, null); LOGGER.info("deployment deleted"); } private static void deleteNamespace(CoreV1Api api) throws ApiException { LOGGER.info("start to delete namespace: {}", NAMESPACE); api.deleteNamespace(NAMESPACE, null, null, null, null, null, null); LOGGER.info("namespace deleted"); } }
FAQ
Question: Is the preceding example applicable to other versions of kubernetes-client/java?
Answer: The preceding example is not applicable to SDKs earlier than 9.0.0, because it uses GenericKubernetesClient, which requires version 9.0.0 or later (see Code Examples).
You need to slightly adjust the preceding example code to apply it to the SDKs of different versions.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot