Using client-go to Access CCI
This section describes how to use cci-iam-authenticator (the CCI authentication tool) and Kubernetes client-go to call the APIs.
Installing cci-iam-authenticator
Download, install, and set cci-iam-authenticator by referring to (Recommended) Using Native kubectl.
Installing Kubernetes client-go
For details, see Installing client-go.
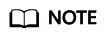
- The Kubernetes version corresponding to CCI open APIs is 1.19. According to Compatibility: client-go <-> Kubernetes clusters, the recommended SDK version is k8s.io/client-go@kubernetes-1.19.0.
- The clusters used in CCI are shared clusters. All namespaces for the clusters and resources in these namespaces cannot be watched. Only resources in the specified namespace can be watched.
Using the Go SDK
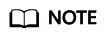
The examples have been tested for the following versions:
- k8s.io/client-go@kubernetes-1.15.0
- k8s.io/client-go@kubernetes-1.16.0
- k8s.io/client-go@kubernetes-1.17.0
- k8s.io/client-go@kubernetes-1.18.0
- k8s.io/client-go@kubernetes-1.19.0
- k8s.io/client-go@kubernetes-1.20.0
import ( "fmt" "k8s.io/client-go/kubernetes" "k8s.io/client-go/tools/clientcmd" "k8s.io/client-go/tools/clientcmd/api" ) const ( apiVersion = "client.authentication.k8s.io/v1beta1" // CCI endpoint: https://developer.huaweicloud.com/intl/en-us/endpoint cciEndpoint = "<For example, https://cci.cn-north-4.myhuaweicloud.com indicates CN North-Beijing4.>" // IAM endpoint: https://developer.huaweicloud.com/intl/en-us/endpoint iamEndpoint = "<For example, https://iam.cn-north-4.myhuaweicloud.com for CN North-Beijing4>" // Regions and endpoint: https://developer.huaweicloud.com/intl/en-us/endpoint projectName = "<Example: cn-north-4 indicates CN North-Beijing4.>" ) // userName, domainName, and password indicate the username, account name, and password used for authentication. Keep the username, account name, and password secure. var userName, domainName, password string // NewClient indicates creating a Clientset using the username/password. func NewClient() (*kubernetes.Clientset, error) { config, err := clientcmd.BuildConfigFromFlags(cciEndpoint, "") if err != nil { return nil, err } var optionArgs []string optionArgs = append(optionArgs, fmt.Sprintf("--iam-endpoint=%s", iamEndpoint)) optionArgs = append(optionArgs, fmt.Sprintf("--project-name=%s", projectName)) optionArgs = append(optionArgs, fmt.Sprintf("--token-only=false")) optionArgs = append(optionArgs, fmt.Sprintf("--domain-name=%s", domainName)) optionArgs = append(optionArgs, fmt.Sprintf("--user-name=%s", userName)) optionArgs = append(optionArgs, fmt.Sprintf("--password=%s", password)) config.ExecProvider = &api.ExecConfig{ Command: "cci-iam-authenticator", APIVersion: apiVersion, Args: append([]string{"token"}, optionArgs...), Env: make([]api.ExecEnvVar, 0), } return kubernetes.NewForConfig(config) }
Using an AK/SK for authentication:
import ( "fmt" "k8s.io/client-go/kubernetes" "k8s.io/client-go/tools/clientcmd" "k8s.io/client-go/tools/clientcmd/api" ) const ( apiVersion = "client.authentication.k8s.io/v1beta1" // CCI endpoint: https://developer.huaweicloud.com/intl/en-us/endpoint cciEndpoint = "<For example, https://cci.cn-north-4.myhuaweicloud.com indicates CN North-Beijing4.>" // IAM endpoint: https://developer.huaweicloud.com/intl/en-us/endpoint iamEndpoint = "<For example, https://iam.cn-north-4.myhuaweicloud.com for CN North-Beijing4>" // Regions and endpoint: https://developer.huaweicloud.com/intl/en-us/endpoint projectName = "<Example: cn-north-4 indicates CN North-Beijing4.>" ) // Obtaining an AK/SK. Refer to https://support.huaweicloud.com/intl/en-us/devg-cci/cci_kubectl_01.html#cci_kubectl_01__section17023744719. // ak and sk indicate the user's AK and SK used for authentication. The AK and SK need to be transferred by the user. Exercise caution when using them. var ak, sk string // NewClient indicates creating a Clientset through AK/SK authentication. func NewClient() (*kubernetes.Clientset, error) { config, err := clientcmd.BuildConfigFromFlags(cciEndpoint, "") if err != nil { return nil, err } var optionArgs []string optionArgs = append(optionArgs, fmt.Sprintf("--iam-endpoint=%s", iamEndpoint)) optionArgs = append(optionArgs, fmt.Sprintf("--project-name=%s", projectName)) optionArgs = append(optionArgs, fmt.Sprintf("--token-only=false")) optionArgs = append(optionArgs, fmt.Sprintf("--ak=%s", ak)) optionArgs = append(optionArgs, fmt.Sprintf("--sk=%s", sk)) config.ExecProvider = &api.ExecConfig{ Command: "cci-iam-authenticator", APIVersion: apiVersion, Args: append([]string{"token"}, optionArgs...), Env: make([]api.ExecEnvVar, 0), } return kubernetes.NewForConfig(config) }
The following example shows how to generate the kubeconfig file for authentication configuration. For details, see the generate-kubeconfig subcommand in cci-iam-authenticator Usage Reference.
import ( "k8s.io/client-go/kubernetes" "k8s.io/client-go/tools/clientcmd" ) // NewClient indicates creating a Clientset using the kubeconfig file. // The kubeconfig file must contain authentication information. For details, see "cci-iam-authenticator Usage Reference" at https://support.huaweicloud.com/intl/en-us/devg-cci/cci_kubectl_03.html. func NewClient() (*kubernetes.Clientset, error) { config, err := clientcmd.BuildConfigFromFlags("", "/path/to/kubeconfig") if err != nil { return nil, err } return kubernetes.NewForConfig(config) }
FAQ
Question: In the preceding examples, is the request result code 401 returned?
Answer: Generally, if the password or AK/SK is correctly configured, a mechanism provided by client-go for periodically calling cci-iam-authenticator to update the token can ensure that the token does not expire (the validity period of the token is 24 hours) and avoid returning code 401. For details, see https://github.com/kubernetes/client-go/blob/master/plugin/pkg/client/auth/exec/exec.go.
However, if the account permissions are changed, the token may also become invalid and the code 401 may be returned.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot