Configuring the JDBC Connection to Connect to a Cluster Using IAM Authentication
When you use the JDBC application program to connect to a cluster, set the IAM username, credential, and other information as you configure the JDBC URL. After doing this, when you try to access a database, the system will automatically generate a temporary credential and a connection will be set up.
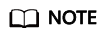
Currently, only clusters whose version is 1.3.1 or later and their corresponding JDBC driver provided by GaussDB(DWS) can access the databases in IAM authentication mode. Download the JDBC driver. For details, see Downloading the JDBC or ODBC Driver.
Configuring JDBC Connection Parameters
Parameter |
Description |
---|---|
url |
gsjdbc4.jar/gsjdbc200.jar database connection descriptor. The JDBC API does not provide the connection retry capability. You need to implement the retry processing in the service code. The URL example is as follows: jdbc:dws:iam://dws-IAM-demo:my-kualalumpur-1/gaussdb?AccessKeyID=XXXXXXXXXXXXXXXXXXXX&SecretAccessKey=XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX&DbUser=user_test&AutoCreate=true
JDBC URL parameters:
|
info |
Database connection properties. Common properties include the following:
|
Example
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
//The following uses gsjdbc4.jar as an example. //The following encapsulates the database connection obtaining operations into an API. You can connect to the database by specifying the region where the cluster is located, cluster name, access key ID, secret access key, and the corresponding IAM username. public static Connection GetConnection(String clustername, String regionname, String AK, String SK, String username) { //Driver class String driver = "org.postgresql.Driver"; // Database connection descriptor. String sourceURL = "jdbc:dws:iam://" + clustername + ":" + regionname + "/gaussdb?" + "AccessKeyID=" + AK + "&SecretAccessKey=" + SK + "&DbUser=" + username + "&autoCreate=true"; Connection conn = null; try { //Load the driver. Class.forName(driver); } catch( Exception e ) { return null; } try { //Create a connection. conn = DriverManager.getConnection(sourceURL); System.out.println("Connection succeed!"); } catch(Exception e) { return null; } return conn; }; |
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot