CONNECT
Description
Establishes a connection between the client and the SQL server.
Syntax
CONNECT TO connection_target [ AS connection_name ] [ USER connection_user ]
Parameters
- connection_target
Specifies the target server to be connected in one of the following formats:
- [ database_name ] [@ host ] [: port ]: connection over TCP/IP.
- unix: postgresql://host [: port ] / [ database_name ] [? connection_option ]: connection over Unix domain sockets.
- tcp: postgresql://host [: port ] / [ database_name ] [? connection_option ]: connection over TCP/IP.
- SQL string constant: one of the preceding forms.
- connection_name
An optional identifier used for the connection, which can be referenced in other commands. It can be an SQL identifier or a host variable.
- connection_user
Username for database connection.
You can use user_name/password, user_name SQLIDENTIFIED BY password, or user_name USING password to specify the username and password.
The username and password can be SQL identifiers, string constants, or host variables.
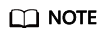
In the preceding parameters, the information in italics refers to variables. Replace them based on the actual situation.
Examples
EXEC SQL CONNECT TO "connectdb" AS main; EXEC SQL CONNECT TO "connectdb" AS second; EXEC SQL CONNECT TO 'connectdb' AS main; EXEC SQL CONNECT TO REGRESSDB1 as main; EXEC SQL CONNECT TO connectdb AS :id; EXEC SQL CONNECT TO connectdb AS main USER connectuser/connectdb; EXEC SQL CONNECT TO connectdb AS main USER connectuser USING "connectdb"; EXEC SQL CONNECT TO connectdb AS main; EXEC SQL CONNECT TO tcp:postgresql://localhost/connectdb USER connectuser IDENTIFIED BY connectpw; EXEC SQL CONNECT TO tcp:postgresql://localhost:$PORT/connectdb USER connectuser SQLIDENTIFIED BY connectpw; EXEC SQL CONNECT TO unix:postgresql://localhost/connectdb USER connectuser SQLIDENTIFIED BY "connectpw"; EXEC SQL CONNECT TO unix:postgresql://localhost/connectdb USER connectuser USING "connectpw";
Example of using the connection syntax:
#include <stdlib.h> #include <string.h> #include <stdlib.h> #include <stdio.h> int main(void) { // Define the host by defining columns such as database and password required by the connection string. The actual values are read from environment variables or configuration files. Environment variables need to be configured as required. If no environment variable is used, a character string can be directly assigned. exec sql begin declare section; const int max_str_len = 200; char db[max_str_len] = getenv("EXAMPLE_DATABASENAME_ENV"); char pw[max_str_len] = getenv("EXAMPLE_PASSWD_ENV"); char new_pw[max_str_len] = getenv("EXAMPLE_NEW_PASSWD_ENV"); exec sql end declare section; // Print debug logs. ECPGdebug(1, stderr); // The connection statement involves the database, username, and password. The user must be created in advance and have related operation permissions. // Connection mode: EXEC SQL CONNECT TO [ database_name ][ @host ][ :port ] [ USER connection_user ] // Case 1: Use the default local connection mode to connect to the postgres database. exec sql connect to postgres; // Case 2: Use the default local connection mode to connect to the postgres database. The connection alias is conn1. exec sql connect to postgres as conn1; // Case 3: Use the ip+port mode (localhost indicates the local IP address listened by the database, and $PORT indicates the listening port of the database) to connect to the connectdb database, specify the database alias, and specify the user password. exec sql connect to connectdb@localhost:$PORT as conn2 user connectuser using :pw; // Case 4: Use the ip+port mode (127.0.0.1 indicates the local address listened by the database, and $PORT indicates the listening port of the database) to connect to the connectdb database, specify the database alias, and specify the user password. exec sql connect to connectdb@127.0.0.1:$PORT as conn3 user connectuser sqlidentified by :pw; // Case 5: Close the connection to the database. exec sql disconnect postgres; exec sql disconnect conn1; exec sql disconnect conn2; exec sql disconnect conn3; // Connection mode: EXEC SQL CONNECT TO <tcp|unix>:<gaussdb|postgresql>://host [ :port ]/[ database_name ][ ?connection_option ] // Case 1: Replace the URL variables with the host variables pw and db. strcpy(pw, new_pw); strcpy(db, "tcp:postgresql://localhost/connectdb"); exec sql connect to :db user connectuser using :pw; // Case 2: 127.0.0.1 indicates the IP address listened by the database, and connectdb indicates the database. exec sql connect to tcp:postgresql://127.0.0.1/connectdb as conn4 user connectuser using :pw; // Case 3: 127.0.0.1 indicates the IP address listened by the database, connectdb indicates the database, and connect_timeout=14 indicates the connection string configuration parameter. exec sql connect to tcp:gaussdb://localhost/connectdb?connect_timeout=14 as conn5 user connectuser sqlidentified by :pw; // Case 4: Close all connections. exec sql close all; // Connect to the database and execute the service. exec sql connect to tcp:postgresql://127.0.0.1/connectdb as conn4 user connectuser using :pw; exec sql set autocommit = on; exec sql create table t1(a int); exec sql insert into t1 values(1),(2); exec sql select a from t1 where a > 1; exec sql drop table t1; exec sql disconnect current; return 0; }
#include <stdio.h> #include <stdlib.h> #include <string.h> int main(void) { EXEC SQL BEGIN DECLARE SECTION; /* The values of dbname, user, and pwd must be read from environment variables or configuration files. Environment variables need to be configured as required. If no environment variable is used, a character string can be directly assigned. */ char *dbname = getenv("EXAMPLE_DBNAME_ENV"); /* Database name */ char *user = getenv("EXAMPLE_USERNAME_ENV"); /* Username for connection */ char *pwd = getenv("EXAMPLE_PASSWD_ENV"); /* Password */ char *connection = "tcp:postgresql://localhost:$PORT/testdb"; /* Connection string */ char ver[256]; /* Buffer for storing version strings */ EXEC SQL END DECLARE SECTION; ECPGdebug(1, stderr); EXEC SQL CONNECT TO :dbname; EXEC SQL SELECT pg_catalog.set_config('search_path', '', false); EXEC SQL COMMIT; EXEC SQL SELECT version() INTO :ver; EXEC SQL DISCONNECT; printf("version: %s\n", ver); EXEC SQL CONNECT TO :connection USER :user USING :pwd; EXEC SQL SELECT pg_catalog.set_config('search_path', '', false); EXEC SQL COMMIT; EXEC SQL SELECT version() INTO :ver; EXEC SQL DISCONNECT; printf("version: %s\n", ver); return 0; }
Helpful Links
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot