Help Center/
GaussDB/
Developer Guide(Centralized_3.x)/
Application Development Guide/
Psycopg-based Development/
Examples: Common Operations
Updated on 2024-05-07 GMT+08:00
Examples: Common Operations
import psycopg2 import os # Obtain the username and password from environment variables. user = os.getenv('user') password = os.getenv('password') # Create a connection object. conn=psycopg2.connect(database="database", user=user, password=password, host="localhost", port=port) cur=conn.cursor() # Create a pointer object. # Create a connection object (using SSL). conn = psycopg2.connect(dbname="database", user=user, password=password, host="localhost", port=port, sslmode="verify-ca", sslcert="client.crt",sslkey="client.key",sslrootcert="cacert.pem") Note: If sslcert, sslkey, and sslrootcert are not set, the following files in the .postgresql directory of the current user are used by default: client.crt, client.key, and root.crt. # Create a table. cur.execute("CREATE TABLE student(id integer,name varchar,sex varchar);") # Insert data. cur.execute("INSERT INTO student(id,name,sex) VALUES(%s,%s,%s)",(1,'Aspirin','M')) cur.execute("INSERT INTO student(id,name,sex) VALUES(%s,%s,%s)",(2,'Taxol','F')) cur.execute("INSERT INTO student(id,name,sex) VALUES(%s,%s,%s)",(3,'Dixheral','M')) # Insert data in batches. stus = ((4,'John','M'),(5,'Alice','F'),(6,'Peter','M')) cur.executemany("INSERT INTO student(id,name,sex) VALUES(%s,%s,%s)",stus) # Obtain the result. cur.execute('SELECT * FROM student') results=cur.fetchall() print (results) # Perform a commit. conn.commit() # Insert a data record. cur.execute("INSERT INTO student(id,name,sex) VALUES(%s,%s,%s)",(7,'Lucy','F')) # Perform a rollback. conn.rollback() # Close the connection. cur.close() conn.close() # Common connection modes of psycopg2. conn = psycopg2.connect(dbname="dbname", user=user, password=password, host="localhost", port=port) conn = psycopg2.connect(f"dbname=dbname user={user} password={password} host=localhost port=port") # Use logs. import logging import psycopg2 from psycopg2.extras import LoggingConnection import os # Obtain the username and password from environment variables. user = os.getenv('user') password = os.getenv('password') logging.basicConfig(level=logging.DEBUG) # Log level logger = logging.getLogger(__name__) db_settings = { "user": user, "password": password, "host": "localhost", "database": "dbname", "port": port } # LoggingConnection records all SQL statements by default. You can filter unnecessary or sensitive SQL statements. Example of filtering password-related SQL statements: class SelfLoggingConnection(LoggingConnection): def filter(self, msg, curs): if db_settings['password'] in msg.decode(): return b'queries containing the password will not be recorded' return msg conn = psycopg2.connect(connection_factory=SelfLoggingConnection, **db_settings) conn.initialize(logger)
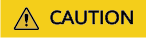
- By default, LoggingConnection records all SQL information and does not anonymize sensitive information. You can use the filter function to define the output SQL content.
- The log function is an additional function provided by psycopg2 for developers to explicitly debug full SQL statements. By default, the log function is not used. This function prints SQL statements before pyscopg2 executes SQL statements. However, the SQL statements can be printed only when the log level is DEBUG. This function is not a default function. It is used only when there are special requirements. You are advised not to use this function unless there are special requirements. For details, visit https://www.psycopg.org/docs/extras.html?highlight=loggingconnection.
Parent topic: Psycopg-based Development
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
The system is busy. Please try again later.
For any further questions, feel free to contact us through the chatbot.
Chatbot