Connecting to a Database (Using UDS)
The Unix domain socket is used for data exchange between different processes on the same host. You can add junixsocket to obtain the socket factory.
The junixsocket-core-XXX.jar, junixsocket-common-XXX.jar, and junixsocket-native-common-XXX.jar JAR packages need to be referenced. In addition, you need to add socketFactory=org.newsclub.net.unix.AFUNIXSocketFactory$FactoryArg&socketFactoryArg=[path-to-the-unix-socket] to the URL connection string.
Example:
// There will be security risks if the username and password used for authentication are directly written into code. It is recommended that the username and password be stored in the configuration file or environment variables (the password must be stored in ciphertext and decrypted when being used) to ensure security. // In this example, the username and password are stored in environment variables. Before running this example, set environment variables EXAMPLE_USERNAME_ENV and EXAMPLE_PASSWORD_ENV in the local environment (set the environment variable names based on the actual situation). import java.sql.Connection; import java.sql.DriverManager; import java.sql.Statement; import java.util.Properties; public class Test { public static void main(String[] args) { String driver = "org.postgresql.Driver"; String userName = System.getenv("EXAMPLE_USERNAME_ENV"); String password = System.getenv("EXAMPLE_PASSWORD_ENV"); Connection conn; try { Class.forName(driver).newInstance(); Properties properties = new Properties(); properties.setProperty("user", userName); properties.setProperty("password", password); conn = DriverManager.getConnection("jdbc:postgresql://$ip:$port/postgres?socketFactory=org.newsclub" + ".net.unix" + ".AFUNIXSocketFactory$FactoryArg&socketFactoryArg=/data/tmp/.s.PGSQL.8000", properties); System.out.println("Connection Successful!"); Statement statement = conn.createStatement(); statement.executeQuery("select 1"); } catch (Exception e) { e.printStackTrace(); } } }
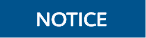
- Set the socketFactoryArg parameter based on the actual path. The value must be the same as that of the GUC parameter unix_socket_directory.
- The connection host name must be set to localhost.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot