API Usage
Overview
OneAccess provides a third-party API authorization management function. API providers configure APIs in OneAccess first. To use these APIs, API consumers obtain authentication tokens from OneAccess, and call the APIs with the authentication tokens. The API providers then determine whether to provide services to the API consumers based on the authentication tokens.
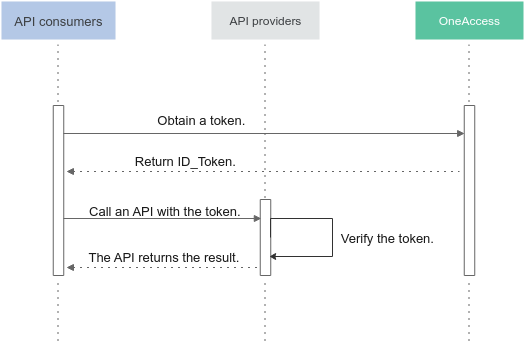
Prerequisite
You have permissions to access the administrator portal.
Adding an Application
Add applications to the administrator portal to provide authentication tokens to API consumers.
- Log in to the administrator portal.
- On the top navigation bar, choose Resources > Applications.
- Click Add Custom Application in the Custom Applications section, set the logo and application name, and click Save.
- Obtain the client ID and client secret.
Click the application logo on the application information page, and obtain the values of Client ID and Client Secret on the application details page. (These values will be provided to API consumers.)
- Click Enable to generate a client secret.
- Client secret is an important credential used to verify the identity of a developer. Do not provide the client secret to other developers nor store it in code.
- If you reset the client secret, the new client secret takes effect immediately, and all APIs that use the old client secret become invalid. Exercise caution when performing this operation.
- OneAccess does not store the client secret. Keep it properly after obtaining it.
Adding an API
Add custom APIs in the administrator portal and authorize access to specific applications.
- Log in to the administrator portal.
- In the top navigation pane, choose Resources > Enterprise APIs.
- On the Enterprise APIs page, click Add Custom APIs.
- On the Add Custom APIs page, upload a product logo, enter the product name and description, and click OK.
- Click the created custom API, click the Application Authorization tab, and click Authorize next to the application added in Adding an Application to authorize the application to use the API.
- Click the Permissions tab and add API permissions.
Granting API Permissions to an Application
Grant permissions for a specific custom API to applications.
- Log in to the administrator portal.
- On the top navigation bar, choose Resources > Applications.
- Click the application added in Adding an Application and then click the logo of it. The General Information page is displayed.
- In the navigation pane, choose API Permissions. In the Operation column of a permission code, click Authorize.
Obtaining the Signature Public Key and Algorithm Key
The authentication tokens issued by OneAccess are encrypted and signed. Prepare the signature public key and algorithm key for the API provider to decrypt the token.
- Log in to the administrator portal.
- In the navigation pane, choose Settings > Service Settings. Click API Authentication to obtain the signature public key and algorithm key, and provide them for the API provider.
OneAccess does not display the algorithm key. After resetting the algorithm key, keep it secure.
(API Consumers) Obtaining an Authentication Token from OneAccess
API consumers obtain the authentication token by calling the OneAccess authentication API.
Access API: https://Access domain name/api/v2/tenant/token?grant_type=client_credentials
This is a Postman calling example.
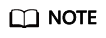
- Replace Access domain name with the real user access domain name. You can obtain it from the OneAccess instance details page.
- Set request method to POST, authentication type to Basic Auth, username and password to the values of Client ID and Client Secret obtained in 4, respectively.
- The returned id_token contains the signature information and the information for third-party API permission authorization. When the API consumers call the third-party API, they can send the value of id_token to the API provider for identity authentication and authorization. They can use header to send the information. The Authorization header is recommended.
- The returned id_token has a validity period, within which the value of id_token can be reused. The validity period is configured in the application.
(API Providers) Verifying a Token
After receiving an API request made by an API consumer with id_token obtained from OneAccess, the API provider needs to verify the token:
- Check whether the signature of the token is correct and whether the token is issued by OneAccess.
- Check whether the permissions declared in the token involve the current API.
Java code example:
import com.alibaba.fastjson.JSON; import lombok.Data; import org.apache.commons.codec.binary.Base64; import org.jose4j.jwa.AlgorithmConstraints; import org.jose4j.jwe.ContentEncryptionAlgorithmIdentifiers; import org.jose4j.jwe.JsonWebEncryption; import org.jose4j.jwe.KeyManagementAlgorithmIdentifiers; import org.jose4j.jwk.JsonWebKey; import org.jose4j.jwt.JwtClaims; import org.jose4j.jwt.consumer.InvalidJwtException; import org.jose4j.jwt.consumer.JwtConsumer; import org.jose4j.jwt.consumer.JwtConsumerBuilder; import org.jose4j.lang.JoseException; import java.io.ByteArrayInputStream; import java.io.InputStream; import java.security.cert.CertificateException; import java.security.cert.CertificateFactory; import java.security.cert.X509Certificate; import java.security.interfaces.RSAPublicKey; import java.util.HashMap; import java.util.List; import java.util.Map; /** * @author : bsong **/ public class JWTTest { public static final String BEGIN_CERT = "-----BEGIN CERTIFICATE-----"; public static final String END_CERT = "-----END CERTIFICATE-----"; public static void main(String[] args) throws CertificateException, InvalidJwtException, JoseException { // The ID token is sent by the API caller String idToken = ""; // Set the algorithm key in OneAccess String aesKey = "0123******************************************789abc"; // Obtain the certificate from the administrator portal String certificate = "-----BEGIN CERTIFICATE-----\n" + "MIIC2jCCAcKgAwI..........................QEBCwUAMC4xLDAqBgNVBAMM\n" + "I2Jzb25nLmlkYWF..........................GUuY29tMB4XDTIyMDExNDA3\n" + "MDY1NVoXDTMyMDE..........................wwjYnNvbmcuaWRhYXMtdGVz\n" + "dC1hbHBoYS5iY2N..........................Ib3DQEBAQUAA4IBDwAwggEK\n" + "AoIBAQCJ7bfMCVX..........................GnE3W9uiSYk3WFkYFK8vh16\n" + "efVuvccAULE+xqi..........................652lsIBNOAC5YPy7J47z4iw\n" + "1GiAVYXxwyehgRe3..........................e0eJDKy6Ew5S+TUq72hqSD7\n" + "zrtQA3szqSK1pgFB..........................J8rMh9WiF2qUqzCdNRqkQRC\n" + "smGGj+PqD86otiif.........................0OPH5UOhR2OEve1cT9dgAlS\n" + "Vt1tKbE0l+iUTQqi..........................oZIhvcNAQELBQADggEBAEP8\n" + "EmkyoaWjngk3Tn5u..........................cJEDGTbuYO55wKap0BTetu6\n" + "cvGFxJYMQYefsx0..........................xn8N4ZgWvwgwDQVQx5WPgAT\n" + "QKunLWz30W4GYUE..........................QJZ7ift2sqoBLmkmjfcyqW0\n" + "jU1+7/e/ea5XAC3..........................DtVHqufwP4R/TALg1muaNyJ\n" + "f7obOcMHAb/OcbP..........................FSAwkVYsxSC9LEEUPhCONvX\n" + "KCWoeQoX/qkZH/nBvXU=\n" + "-----END CERTIFICATE-----"; RSAPublicKey publicKey = getPublicKeyByCertificate(certificate); JsonWebKey jsonWebKey = getJsonWebKey(aesKey); JwtClaims jwtClaims = validateIDToken(publicKey, idToken); String apiPermission = jwtClaims.getClaimValue("api").toString(); String permissionString = decryptionIDToken(jsonWebKey, apiPermission); System.out.println(permissionString); Map<String, List<String>> permissions = getPermissionsFromIdToken(permissionString); System.out.println(permissions); } public static Map<String, List<String>> getPermissionsFromIdToken(String permissionString) throws JoseException { Map<String, List<String>> result = new HashMap<>(); Permission permission = JSON.parseObject(permissionString,Permission.class); permission.getAuz().stream().forEach(p ->{ p.entrySet().forEach(e->{ result.put(e.getKey(),e.getValue()); }); }); return result; } @Data public static class Permission{ List<Map<String, List<String>>> auth_method; List<Map<String, List<String>>> auz; } public static RSAPublicKey getPublicKeyByCertificate(String certificate) throws CertificateException { CertificateFactory fact = CertificateFactory.getInstance("X.509"); byte[] decoded = Base64.decodeBase64(certificate.replace(BEGIN_CERT, "").replace(END_CERT, "")); InputStream input = new ByteArrayInputStream(decoded); X509Certificate cert = (X509Certificate) fact.generateCertificate(input); return (RSAPublicKey) cert.getPublicKey(); } public static JsonWebKey getJsonWebKey(String key) throws JoseException { Map<String,Object> map = new HashMap<>(); map.put("kty","oct"); map.put("k",key); String jwkJson = JSON.toJSONString(map); return JsonWebKey.Factory.newJwk(jwkJson); } public static JwtClaims validateIDToken( RSAPublicKey publicKey,String idToken) throws InvalidJwtException { JwtConsumer jwtConsumer = new JwtConsumerBuilder() .setRequireExpirationTime() // The JWT must have an expiration time .setAllowedClockSkewInSeconds(300) // Allow some leeway in validating time-based claims to account for clock skew .setRequireSubject() // The JWT must have a subject claim .setExpectedIssuer("Issuer") // Whom the JWT needs to have been issued by .setExpectedAudience("Audience") // Whom the JWT is intended for .setVerificationKey(publicKey) .build(); return jwtConsumer.processToClaims(idToken); } public static String decryptionIDToken(JsonWebKey jwk, String idToken) throws JoseException { JsonWebEncryption jsonWebEncryption = new JsonWebEncryption(); jsonWebEncryption.setAlgorithmConstraints(new AlgorithmConstraints(AlgorithmConstraints.ConstraintType.PERMIT, KeyManagementAlgorithmIdentifiers.DIRECT)); jsonWebEncryption.setContentEncryptionAlgorithmConstraints(new AlgorithmConstraints(AlgorithmConstraints.ConstraintType.PERMIT, ContentEncryptionAlgorithmIdentifiers.AES_128_CBC_HMAC_SHA_256)); jsonWebEncryption.setCompactSerialization(idToken); jsonWebEncryption.setKey(jwk.getKey()); return jsonWebEncryption.getPlaintextString(); } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot