Development Specifications
Preventing Chaincode Container from Failing After a Panic
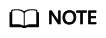
This section applies only to the Go chaincode development.
When a panic exception occurs, the chaincode container may be suspended and restarted, logs cannot be found, and the problem cannot be located immediately. To prevent this case, add the defer statement at the entry point of the Invoke function. When a panic occurs, the error is returned to the client.
// Name the return value in the defer statement to ensure that the client can receive the value if a panic occurs. //Use debug.PrintStack() to print the stack trace to the standard output for fault locating. func (t *SimpleChaincode) Invoke(stub shim.ChaincodeStubInterface) (pr pb.Response) { defer func() { if err:=recover(); err != nil { fmt.Println("recover from invoke:", err) debug.PrintStack() pr = shim.Error(fmt.Sprintf("invoke painc. err: %v",err)) } }() fmt.Println("ex02 Invoke") function, args := stub.GetFunctionAndParameters() if function == "invoke" { // Make payment of X units from A to B return t.invoke(stub, args) } else if function == "delete" { // Deletes an entity from its state return t.delete(stub, args) } else if function == "query" { // the old "Query" is now implemented in invoke return t.query(stub, args) } pr = shim.Error("Invalid invoke function name. Expecting \"invoke\" \"delete\" \"query\"") return pr }
Querying Data in Batches
If too many records are returned in one query, too many resources will be occupied and the interface delay will be long. For example, if the interface delay exceeds 30s, the peer task will be interrupted. Therefore, estimate the data volume in advance, and query data in batches if the data volume is large.
To modify or delete the ledger data chaincode, consider performing operations in batches based on the data volume.
Using Indexes with CouchDB
Using indexes with CouchDB accelerates data querying, but slows data writing. Therefore, create indexes only for certain fields based on service requirements.
Verifying Permissions
Verify the permissions of the smart contract executor to prevent unauthorized users from executing chaincodes.
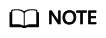
If no specified organization is required for the endorsement, select at least two endorsing organizations to ensure that the chaincode data is not maliciously modified (such as installing invalid chaincode or processing data) by any other organizations.
Verifying Parameters
Before parameters (including input parameters and parameters defined in code) are used, the quantity, type, length, and value range of the parameters must be verified to prevent array out-of-range.
Processing Logs
During the development of services that have a complex logic and are prone to error, use fmt to print logs to facilitate debugging. fmt consumes a lot of time and resources. Delete the logs after the debugging is complete.
Configuring Dependencies
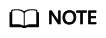
This section applies only to the Java chaincode development.
Use Gradle or Maven to manage chaincode projects. If the chaincode project contains non-local dependencies, ensure that all nodes of the BCS instance are bound with EIPs. If the chaincode container runs in a restricted network environment, ensure that all dependencies in the project are configured as local dependencies. To obtain the chaincode used in this section, go to the BCS console and click Use Cases. Download Chaincode_Java_Local_Demo in the Java SDK Demo area.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot