Resource-Related SDKs
Prerequisites
- You have configured the Java SDK environment by following the instructions provided Instructions.
- You have initialized the DLI Client by following the instructions provided in Initializing the DLI Client.
Uploading a Resource Package
You can use the interface provided by the DLI to upload resource packages. The code example is as follows:
1 2 3 4 5 6 7 8 9 |
private static void uploadResources(DLIClient client) throws DLIException { String kind = "jar"; String[] paths = new String[1]; paths[0] = "https://bucketname.obs.com/jarname.jar"; String description = "test for sdk"; //Call the uploadResources method of the DLIClient object to upload resources. List<PackageResource> packageResources = client.uploadResources(kind, paths, description); System.out.println("---------- uploadResources success ---------"); } |
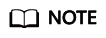
The following describes the request parameters. For details, see Instructions.
- kind: resource package type. The options are as follows:
- jar: JAR file
- Pyfile: User Python file
- file: User file
- modelfile: User AI model file
- paths: OBS path of the resource package. The parameter format is {bucketName}.{obs domain name}/{jarPath}/{jarName}.
- description: description of the resource package
Querying All Resource Packages
You can use the API provided by DLI to query the list of uploaded resources. Sample code is as follows:
1 2 3 4 5 6 7 8 9 10 11 |
private static void listAllResources(DLIClient client) throws DLIException { System.out.println("list all resources..."); //Call the listAllResources method of the DLIClient object to query the queue resource list. Resources resources = client.listAllResources(); for (PackageResource packageResource : resources.getPackageResources()) { System.out.println("Package resource name:" + packageResource.getResourceName()); } for (ModuleResource moduleResource : resources.getModuleResources()) { System.out.println("Module resource name:" + moduleResource.getModuleName()); } } |
Querying a Specified Resource Package
private static void getResource(DLIClient client) throws DLIException { String resourceName = "xxxxx"; // group: If the resource package is not in the group, leave this parameter empty. String group= "xxxxxx"; // Call getResource on the DLIClient object to query a specified resource package. PackageResource packageResource=client.getResource(resourceName,group); System.out.println(packageResource); }
Deleting a Resource Package
private static void deleteResource(DLIClient client) throws DLIException { String resourceName = "xxxxx"; // group: If the resource package is not in the group, leave this parameter empty. String group= "xxxxxx"; //Call deleteResource on the DLIClient object to upload resources. client.deleteResource(resourceName,group); System.out.println("---------- deleteResource success ---------"); }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.