Table-Related SDKs
Creating a DLI Table
DLI provides an API for creating DLI tables. You can use it to create a table for storing DLI data. Sample code is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
private static Table createDLITable(Database database) throws DLIException { // Construct a table column set and instantiate the Column object to construct columns. List<Column> columns = new ArrayList<Column>(); Column c1 = new Column("c1", DataType.STRING, "desc for c1"); Column c2 = new Column("c2", DataType.INT, "desc for c2"); Column c3 = new Column("c3", DataType.DOUBLE, "desc for c3"); Column c4 = new Column("c4", DataType.BIGINT, "desc for c4"); Column c5 = new Column("c5", DataType.SHORT, "desc for c5"); Column c6 = new Column("c6", DataType.LONG, "desc for c6"); Column c7 = new Column("c7", DataType.SMALLINT, "desc for c7"); Column c8 = new Column("c8", DataType.BOOLEAN, "desc for c8"); Column c9 = new Column("c9", DataType.DATE, "desc for c9"); Column c10 = new Column("c10", DataType.TIMESTAMP, "desc for c10"); Column c11 = new Column("c11", DataType.DECIMAL, "desc for c11"); columns.add(c1); columns.add(c2); columns.add(c3); columns.add(c4); columns.add(c5); columns.add(c6); columns.add(c7); columns.add(c8); columns.add(c9); columns.add(c10); columns.add(c11); List<String> sortColumns = new ArrayList<String>(); sortColumns.add("c1"); String DLITblName = "tablename"; String desc = "desc for table"; // Call the createDLITable method of the Database object to create a DLI table. Table table = database.createDLITable(DLITblName, desc, columns, sortColumns); System.out.println(table); return table; } |
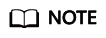
The default precision of DataType.DECIMAL is (10,0). To set the precision of data of the decimal type, perform the following operation:
1
|
Column c11 = new Column("c11", new DecimalTypeInfo(25,5), "test for c11"); |
Creating an OBS Table
DLI provides an API for creating OBS tables. You can use it to create a table for storing OBS data. The example code is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
private static Table createObsTable(Database database) throws DLIException { // Construct a table column set and instantiate the Column object to construct columns. List < Column > columns = new ArrayList < Column > (); Column c1 = new Column("c1", DataType.STRING, "desc for c1"); Column c2 = new Column("c2", DataType.INT, "desc for c2"); Column c3 = new Column("c3", DataType.DOUBLE, "desc for c3"); Column c4 = new Column("c4", DataType.BIGINT, "desc for c4"); Column c5 = new Column("c5", DataType.SHORT, "desc for c5"); Column c6 = new Column("c6", DataType.LONG, "desc for c6"); Column c7 = new Column("c7", DataType.SMALLINT, "desc for c7"); Column c8 = new Column("c8", DataType.BOOLEAN, "desc for c8"); Column c9 = new Column("c9", DataType.DATE, "desc for c9"); Column c10 = new Column("c10", DataType.TIMESTAMP, "desc for c10"); Column c11 = new Column("c11", DataType.DECIMAL, "desc for c11"); columns.add(c1); columns.add(c2); columns.add(c3); columns.add(c4); columns.add(c5); columns.add(c6); columns.add(c7); columns.add(c8); columns.add(c9); columns.add(c10); columns.add(c11); CsvFormatInfo formatInfo = new CsvFormatInfo(); formatInfo.setWithColumnHeader(true); formatInfo.setDelimiter(","); formatInfo.setQuoteChar("\""); formatInfo.setEscapeChar("\\"); formatInfo.setDateFormat("yyyy/MM/dd"); formatInfo.setTimestampFormat("yyyy-MM-dd HH:mm:ss"); String obsTblName = "tablename"; String desc = "desc for table"; String dataPath = "OBS path"; // Call the createObsTable method of the Database object to create an OBS table. Table table = database.createObsTable(obsTblName, desc, columns,StorageType.CSV , dataPath, formatInfo); System.out.println(table); return table; } |
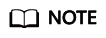
The default precision of DataType.DECIMAL is (10,0). To set the precision of data of the decimal type, perform the following operation:
1
|
Column c11 = new Column("c11", new DecimalTypeInfo(25,5), "test for c11"); |
Deleting a Table
DLI provides an API for deleting tables. You can use it to delete all the tables in a database. The example code is as follows:
1 2 3 4 5 6 7 8 9 |
private static void deleteTables(Database database) throws DLIException { // Call the listAllTables interface of the Database object to query all tables. List<Table> tables = database.listAllTables(); for (Table table : tables) { // Traverse tables and call the deleteTable interface of the Table object to delete tables. table.deleteTable(); System.out.println("delete table " + table.getTableName()); } } |
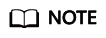
A deleted table cannot be restored. Therefore, exercise caution when deleting a table.
Querying All Tables
DLI provides an API for querying tables. You can use it to query all tables in a database. The example code is as follows:
1 2 3 4 5 6 7 |
private static void listTables(Database database) throws DLIException { // Call the listAllTables method of the Database object to query all tables in a database. List<Table> tables = database.listAllTables(true); for (Table table : tables) { System.out.println(table); } } |
Querying the Partition Information of a Table (Including the Creation and Modification Time of the Partition).
1 2 3 4 5 6 7 8 9 10 11 |
private static void showPartitionsInfo(DLIClient client) throws DLIException { String databaseName = "databasename"; String tableName = "tablename"; // Call the showPartitions method of the DLIClient object to query the partition information (including the partition creation and modification time) in the database table. PartitionResult partitionResult = client.showPartitions(databaseName, tableName); PartitionListInfo partitonInfos = partitionResult.getPartitions(); // Obtain the creation and modification time of the partition. Long createTime = partitonInfos.getPartitionInfos().get(0).getCreateTime().longValue(); Long lastAccessTime = partitonInfos.getPartitionInfos().get(0).getLastAccessTime().longValue(); System.out.println("createTime:"+createTime+"\nlastAccessTime:"+lastAccessTime); } |
Describing Table Information
private static void getTableDetail(Table table) throws DLIException { // Call getTableDetail on the Table object to obtain table information. // TableSchema tableSchema=table.getTableDetail(); // Output System.out.println(List<Column> columns = tableSchema.getColumns()); System.out.println(List<String> sortColumns = tableSchema.getSortColumns()); System.out.println(String createTableSql = tableSchema.getCreateTableSql()); System.out.println(String tableComment = tableSchema.getTableComment()); }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.