Supported Expressions, Functions, and Procedures
Expression
Cypher queries support multiple expressions and can be used in combination to form various filter criteria. Currently, the following expressions are supported.
Operation Type |
Expression |
Example |
---|---|---|
Logical operations |
and |
match (n:user) where n.age='Under 18' and n.gender='F' return n |
or |
match(n:user) where n.`Zip-code`='22181' or n.userid=6 return n |
|
not |
match(n:movie) where not n.genres contains 'Drama' return n |
|
Null value judgment |
is null |
match (n) where n.userid is null return n |
is not null |
match (n) where n.userid is not null return n |
|
Comparison calculation |
>,>=,<,<=,=,<> |
match(n:user) where n.userid>=5 return n |
Arithmetic operators (2.3.10) |
+,-,*,/,%,^ |
return (1+3)%3 |
String comparisons |
starts with |
match(n:movie) where n.genres starts with 'Comedy' return n |
ends with |
match(n:movie) where n.genres ends with 'Drama' return n |
|
contains |
match(n:movie) where n.genres contains 'Drama' return n |
|
List-related operation |
in |
match(n:student) where 'math' in n.courses return n |
[] |
match(n:user) return n[' userid'] with [1, 2, 3, 4] as list return list[0] with [1, 2, 3, 4] as list return list[0..1] match p=(n)-->(m) return [x in nodes(p) where x.gender='F'|id(x)] |
|
Date expressions (2.3.10) |
.year, .month, .day, .hour, .minute, .second, .dayOfWeek |
Year, month, and day of a specific date: with '2000-12-27 23:44:41' as strVal with datetime(strVal) as d2 return d2.year, d2.month, d2.day, d2.hour, d2.minute, d2.second,d2.dayOfWeek, d2.ordinalDay |
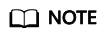
The where clause in Cypher queries does not support regular expressions.
Function
Cypher supports the following functions for grouping, aggregation, and vertex and edge operations:
- Aggregate
Aggregate functions count and collect are supported.
Function
Earliest Version Supported
Description
Example
count
2.2.17
Returns the total number of results.
match (n) return count(*)
match (n) return count(n.userid)
collect
2.2.17
Collects results into a list.
match (n:movie) return n.genres, collect(n) as movieList
sum
2.3.3
Returns the sum of values.
unwind [1, 2.0, 3] as p return sum(p)
avg
2.3.3
Returns the average of values.
unwind [1, 2.0, 3] as p return avg(p)
min
2.3.3
Returns the minimum value.
unwind [1, 2.0, 3] as p return min(p)
max
2.3.3
Returns the maximum value.
unwind [1, 2.0, 3] as p return max(p)
- Regular functions
Based on the types of input parameters, regular functions are classified into vertex and edge functions, path functions, list functions, and value functions.
Table 1 Vertex and edge functions Function
Earliest Version Supported
Description
Example
id
2.2.16
Obtains the ID of a vertex.
match (n) return id(n)
labels
2.2.16
Obtains labels of a vertex.
match (n) return labels(n)
type
2.2.16
Obtains the label of an edge.
match(n)-[r]->(m) return type(r)
degree
2.2.26
Obtains the degree of a vertex.
match (n) where id='Vivian' return degree(n)
inDegree
2.2.26
Obtains the indegree of a vertex.
match (n) where id='Vivian' return inDegree(n)
outDegree
2.2.26
Obtains the outdegree of a vertex.
match (n) where id='Vivian' return outDegree(n)
startNode
2.3.10
Obtains the start vertex of an edge.
match (n)-[r]->(m) return startNode(r)
endNode
2.3.10
Obtains the end vertex of an edge.
match (n)-[r]->(m) return endNode(r)
Table 2 Path functions Function
Earliest Version Supported
Description
Example
nodes
2.2.19
Obtains the list of vertices on a path.
match p=(n)-[:friends*1..2]->(m) return nodes(p)
relationships
2.2.19
Obtains the list of edges on a path.
match p=(n)-[:friends*1..2]->(m) return relationships(p)
length
2.2.19
Obtains the path length.
match p=(n)-[:friends*1..2]->(m) return length(p)
Table 3 List functions Function
Earliest Version Supported
Description
Example
head
2.3.10
Obtains the first element of a list.
with [1,2,3,4] as list return head(list)
last
2.3.10
Obtains the last element of a list.
with [1,2,3,4] as list return last(list)
size
2.3.10
Obtains the list length.
with [1,2,3,4] as list return size(list)
range
2.3.10
Generates a list.
return range(1,5), range(1,5,2)
Table 4 Value functions Function
Earliest Version Supported
Description
Example
toString
2.2.21
Converts a value to a string.
match (n) where toString(labels(n)) contains 'movi' return n
toUpper
2.2.26
Converts a string into uppercase letters.
match (n:movie) return toUpper(n.title)
toLower
2.2.26
Converts a string into lowercase letters.
match (n:movie) return toLower(n.title)
toInteger
2.2.29
Converts a string to an int number.
with '123' as p return toInteger(p)
toLong
2.2.29
Converts a string to a long number.
with '123' as p return toLong(p)
toFloat
2.2.29
Converts a string to a float number.
with '123.4' as p return toFloat(p)
toDouble
2.2.29
Converts a string to a double number.
with '123.4' as p return toDouble(p)
toBoolean
2.2.29
Converts a string to a bool value.
with 'true' as p return toBoolean(p)
size
2.2.29
Obtains the string length.
with 'GES' as p return size(p)
subString
2.3.10
Truncates a part of a string.
return subString('abc', 1), subString('abcde', 1,2)
coalesce
2.3.10
Obtains the first non-null value of the parameters.
return coalesce(null, '123')
Table 5 Mathematical functions Function
Earliest Version Supported
Description
Example
floor
2.3.10
Rounds a number down to the nearest integer.
return floor(4.1)
ceil
2.3.10
Rounds a number up to the nearest integer.
return ceil(4.1)
round
2.3.14
Round
return round(3.4), round(3.5)
abs
2.3.14
Absolute value function
return abs(-3),abs(-3.5)
sin
2.3.14
Sine function
return sin(pi()/2)
cos
2.3.14
Cosine function
return cos(0),cos(pi()/2)
tan
2.3.14
Tangent function
return tan(pi()/4)
acos
2.3.14
Inverse cosine function
return acos(1)
asin
2.3.14
Inverse sine function
return asin(0)
atan
2.3.14
Inverse tangent function
return atan(1)
cot
2.3.14
Cotangent function
return cot(pi()/4)
radians
2.3.14
Converts degree to radian
return radians(180)
degrees
2.3.14
Converts radian to degree
return degrees(pi())
pi
2.3.14
Returns the approximate value of Pi (π).
return pi()
Table 6 Date and time functions Function
Earliest Version Supported
Description
Example
datetime(val)
2.3.10
Returns the time based on the timestamp.
return datetime(1688696395)
datetime()
2.3.14
Obtains the current time (valid only for read statements).
return datetime()
timestamp(val)
2.3.10
Returns the timestamp based on the time string.
return timestamp('2023-07-07 02:20:42')
timestamp()
2.3.14
Obtains the current timestamp (valid only for read statements).
return timestamp()
localDatetime
2.3.14
Converts a time or timestamp to a local time string.
return localDatetime(timestamp())
Table 7 Predicate functions Function
Earliest Version Supported
Description
Example
all
2.2.19
If all elements meet the expression, true is returned.
all (x in p where x>1)
any
2.2.19
If any element meets the expression, true is returned.
any (x in p where x>1)
none
2.2.19
If all elements cannot meet the expression, true is returned.
none (x in p where x>1)
single
2.2.19
If only one element meets the expression, true is returned.
single (x in p where x>1)
Table 8 Algorithm expressions Function
Earliest Version Supported
Description
Example
shortestPath
2.3.2
Returns the shortest path between two vertices.
The following statement returns the shortest path between the given vertices n and m. The direction is m to n, and the edge label is rate:
with n,m, shortestPath((n)<-[:rate*]-(m)) as p return p
allShortestPaths
2.3.2
Returns all shortest paths between two vertices.
The following statement returns all shortest paths between the given vertices n and m:
with n,m, allShortestPaths((n)-[*]-(m)) as p return p
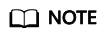
Procedure
Currently, GES supports the following procedures.
Procedure |
Statement |
---|---|
Obtaining graph pattern information |
call db.schema() |
Obtaining vertex labels |
call db.labels() |
Obtaining edge labels |
call db.relationshipTypes() |
Querying the Cypher statements that are being executed |
call dbms.listQueries() |
Terminating a Cypher statement based on queryId |
call dbms.killQuery('queryId') |
Querying indexes |
call db.indexes() |
Full-text indexing for querying vertices that meet the search conditions |
call db.index.fulltext.queryNodes() |
Full-text indexing for querying edges that meet the conditions |
call db.index.fulltext.queryRelationships() |
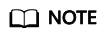
Full-text indexes support six types of queries: prefix, wildcard, regexp, fuzzy, match, and combine. To use full-text indexes, you need to call the API for creating a full-text index.
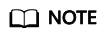
Function and procedure names are case sensitive and must be in lower camel case.
- Example of a full-text index query request
POST http://{SERVER_URL}/ges/v1.0/{project_id}/graphs/{graph_name}/action?action_id=execute-cypher-query { "statements": [ { "statement": "call db.index.fulltext.queryNodes('combine', {title:'1977'}) yield node, score return node, score skip 1 limit 10", "resultDataContents": [ "row" ], "parameters": {} } ] }
- Parallel edge processing policy
When using Cypher to add edges, you can add duplicate edges. Duplicate edges are two edges with the same source vertex and target vertex.
- How to add an edge without a label
When you use a Cypher statement to add an edge, set the label of the edge to the default value __DEFAULT__. For example, create ()-[r:__DEFAULT__]->() return r.
Querying the Schema Structure Using Cypher
- Function
You can call the db.schema () function using Cypher to query the structure of a generated schema (obtained from OBS).
- Query statement
- Name: Schema structure query
- Statement: call db.schema()
- Note:
If you did not call the API for generating the schema structure, the returned schema file contains all labels.
If you have called the API for generating the schema structure, this API returns the labels as the vertices and the relationships between the labels as edges.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.