更新时间:2023-03-23 GMT+08:00
场景10:发起呼叫
描述
在使用华为云会议帐号登录后,可以调用发起呼叫接口发起一路呼叫。
业务流程
使用SDK发起时,先调用StartCall接口,然后处理回调函数OnStartCallResult和消息通知OnCallState、OnCallInfo、OnCallRecordInfo。
- 接口调用
- 组装数据结构HwmStartCallInfo。
- 调用StartCall开始创建,第1步中的数据作为参数。
- 处理回调函数
处理回调函数OnStartCallResult。
- 处理消息通知
处理消息通知OnCallState。
- 处理消息通知
处理消息通知OnCallInfo。
示例代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
/** * 发起呼叫 */ int demoStartCallDlg::clickStartCall() { hwmsdkagent::HwmStartCallInfo data; memset(&data, 0, sizeof(hwmsdkagent::HwmStartCallInfo)); //被叫人姓名 CString calleeNameCstr; m_calleeNameEdit.GetWindowText(calleeNameCstr); string calleeName = CTools::UNICODE2UTF(calleeNameCstr); errno_t nRet = strcpy_s(data.callee.name, sizeof(data.callee.name), calleeName.c_str()); //被叫人号码 CString calleeNumberCstr; m_calleeNumberEdit.GetWindowText(calleeNumberCstr); string calleeNumber = CTools::UNICODE2UTF(calleeNumberCstr); nRet += strcpy_s(data.callee.number, sizeof(data.callee.number), calleeNumber.c_str()); //被叫人三方账号 CString calleeThirdUserIdCstr; m_calleeThirdUserIdEdit.GetWindowText(calleeThirdUserIdCstr); string calleeThirdUserId = CTools::UNICODE2UTF(calleeThirdUserIdCstr); nRet += strcpy_s(data.callee.thirdUserId, sizeof(data.callee.thirdUserId), calleeThirdUserId.c_str()); //主叫人姓名 CString callerNameCstr; m_callerNameEdit.GetWindowText(callerNameCstr); string callerName = CTools::UNICODE2UTF(callerNameCstr); nRet += strcpy_s(data.name, sizeof(data.name), callerName.c_str()); if (nRet != 0) { AfxMessageBox(_T("String Copy Error!")); } //视频或音频 CString callTypeCString; m_callTypeCombo.GetWindowText(callTypeCString); hwmsdkagent::HwmCallType type = hwmsdkagent::CALL_TYPE_VIDEO; if (_T("Video") == callTypeCString) { data.callType = hwmsdkagent::CALL_TYPE_VIDEO; } else if (_T("Audio") == callTypeCString) { data.callType = hwmsdkagent::CALL_TYPE_AUDIO; } int ret = hwmsdkagent::StartCall(&data); return ret; } |
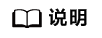
Windows SDK接口中的字符串参数,比如被叫方姓名、呼叫方姓名等,需要编码成UTF8,否则接口会报错。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
/** * 发起呼叫接口回调 */ void demoCallbackProc::OnStartCallResult(hwmsdk::HwmErrCode ret, const char * reason) { Cdemo* app = (Cdemo*)AfxGetApp(); if (!app || reason == NULL) { //窗口已经关闭 return; } CString codeStr; codeStr.Format(_T("%d"), ret); string msgStr = CTools::UTF82MultiByte(reason); CString tips = _T("OnStartCallResult code:") + codeStr + _T(", msg:") + CString(msgStr.c_str()); AfxMessageBox(tips); } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
/** * 通话状态消息通知 */ void demoNotifyProc::OnCallState(const HwmCallStateInfo *callStateInfo) { Cdemo* app = (Cdemo*)AfxGetApp(); if (!app || callStateInfo == NULL) { //窗口已经关闭 return; } CString state; state.Format(_T("%d"), callStateInfo->state); CString callId; callId.Format(_T("%d"), callStateInfo->callId); CString tips = _T("OnCallState state:") + state + _T(", callId:" + callId); AfxMessageBox(tips); } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
/** * 通话信息消息通知 */ void demoNotifyProc::OnCallInfo(const HwmCallInfo *callInfo) { Cdemo* app = (Cdemo*)AfxGetApp(); if (!app || callInfo == NULL) { //窗口已经关闭 return; } CString callType; callType.Format(_T("%d"), callInfo->callType); string number = CTools::UTF82MultiByte(callInfo->number); string name = CTools::UTF82MultiByte(callInfo->name); string startTime = CTools::UTF82MultiByte(callInfo->startTime); string endTime = CTools::UTF82MultiByte(callInfo->endTime); CString isCallOut; isCallOut.Format(_T("%d"), callInfo->isCallOut); CString callId; callId.Format(_T("%d"), callInfo->callId); CString tips = _T("OnCallInfo callType:") + callType + _T(", number:") + CString(number.c_str()) + _T(", name:") + CString(name.c_str()) + _T(", startTime:") + CString(startTime.c_str()) + _T(", endTime:") + CString(endTime.c_str()) + _T(", isCallOut:") + isCallOut + _T(", callId:") + callId; AfxMessageBox(tips); } |
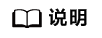
Windows SDK回调函数或者消息通知中的字符串都是UTF8编码的。
父主题: 典型场景