更新时间:2024-09-03 GMT+08:00
使用trident-sdk发送gRPC请求
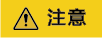
trident sdk截止至版本0.7.0,尚未支持tls认证的方式进行gRPC请求,因此需要按照示例代码,通过java反射的方式,打开trident的tls认证进行节点对接。
在节点页面下载证书,将压缩包内的ca.crt证书放在项目内的目录。
在sdk中配置gRPC endpoint,示例代码如下:
import io.gRPC.*; import io.gRPC.stub.MetadataUtils; import org.tron.trident.api.WalletgRPC; import org.tron.trident.api.WalletSoliditygRPC; import org.tron.trident.core.ApiWrapper; import org.tron.trident.core.exceptions.IllegalException; import org.tron.trident.core.key.KeyPair; import java.io.File; import java.io.IOException; import java.lang.reflect.Field; public class Main { public static void main(String[] args) throws IllegalException, IOException, NoSuchFieldException, IllegalAccessException { String gRPCEndpoint = "your-gRPC-endpoint"; String gRPCSolidityEndpoint = "your-gRPCsolidity-endpoint"; String credential = "your-credential"; String hexPrivateKey = "your-hex-private-key"; ApiWrapper wrapper = new ApiWrapper(gRPCEndpoint, gRPCSolidityEndpoint, hexPrivateKey, credential); // modify wallet channel through reflex ChannelCredentials creds = TlsChannelCredentials.newBuilder() .trustManager(new File("your-ca.crt-file-path")) .build(); ManagedChannel channel = gRPC.newChannelBuilder(gRPCEndpoint, creds) .build(); Metadata header = new Metadata(); Metadata.Key<String> key = Metadata.Key.of("TRON-PRO-API-KEY", Metadata.ASCII_STRING_MARSHALLER); header.put(key, credential); setField(ApiWrapper.class, wrapper, "channel", channel); setField(ApiWrapper.class, wrapper, "blockingStub", MetadataUtils.attachHeaders(WalletgRPC.newBlockingStub(channel), header)); // modify walletsolidity channel through reflex ManagedChannel channelSolidity = gRPC.newChannelBuilder(gRPCSolidityEndpoint, creds) .build(); setField(ApiWrapper.class, wrapper, "channelSolidity", channelSolidity); setField(ApiWrapper.class, wrapper, "blockingStubSolidity", MetadataUtils.attachHeaders(WalletSoliditygRPC.newBlockingStub(channelSolidity), header)); // getNowBlock & getNowBlockSolidity test System.out.println(wrapper.getNowBlock()); System.out.println(wrapper.getNowBlockSolidity()); System.out.println("finish"); } private static void setField(Class clazz, Object obj, String fieldName, Object value) throws NoSuchFieldException, IllegalAccessException { Field field = clazz.getDeclaredField(fieldName); field.setAccessible(true); field.set(obj, value); } }
Response example:
父主题: gRPC请求示例