更新时间:2024-01-11 GMT+08:00
链代码调测
链代码调测主要是使用shim包中的MockStub进行单元测试,所以您需要在测试代码中引入shim包。您可参考下文提供的示例测试代码,也可参考Fabric官方示例中的其它测试代码。
编写测试代码
账户转账链代码示例的测试代码内容如下:
package main import ( "fmt" "testing" "github.com/hyperledger/fabric/core/chaincode/shim" ) func checkInit(t *testing.T, stub *shim.MockStub, args [][]byte) { res := stub.MockInit("1", args) if res.Status != shim.OK { fmt.Println("Init failed", string(res.Message)) t.FailNow() } } func checkState(t *testing.T, stub *shim.MockStub, name string, value string) { bytes := stub.State[name] if bytes == nil { fmt.Println("State", name, "failed to get value") t.FailNow() } if string(bytes) != value { fmt.Println("State value", name, "was not", value, "as expected") t.FailNow() } } func checkQuery(t *testing.T, stub *shim.MockStub, name string, value string) { res := stub.MockInvoke("1", [][]byte{[]byte("query"), []byte(name)}) if res.Status != shim.OK { fmt.Println("Query", name, "failed", string(res.Message)) t.FailNow() } if res.Payload == nil { fmt.Println("Query", name, "failed to get value") t.FailNow() } if string(res.Payload) != value { fmt.Println("Query value", name, "was not", value, "as expected") t.FailNow() } } func checkInvoke(t *testing.T, stub *shim.MockStub, args [][]byte) { res := stub.MockInvoke("1", args) if res.Status != shim.OK { fmt.Println("Invoke", args, "failed", string(res.Message)) t.FailNow() } } func TestExample02_Init(t *testing.T) { scc := new(SimpleChaincode) stub := shim.NewMockStub("ex02", scc) // Init A=123 B=234 checkInit(t, stub, [][]byte{[]byte("init"), []byte("A"), []byte("123"), []byte("B"), []byte("234")}) checkState(t, stub, "A", "123") checkState(t, stub, "B", "234") } func TestExample02_Query(t *testing.T) { scc := new(SimpleChaincode) stub := shim.NewMockStub("ex02", scc) // Init A=345 B=456 checkInit(t, stub, [][]byte{[]byte("init"), []byte("A"), []byte("345"), []byte("B"), []byte("456")}) // Query A checkQuery(t, stub, "A", "345") // Query B checkQuery(t, stub, "B", "456") } func TestExample02_Invoke(t *testing.T) { scc := new(SimpleChaincode) stub := shim.NewMockStub("ex02", scc) // Init A=567 B=678 checkInit(t, stub, [][]byte{[]byte("init"), []byte("A"), []byte("567"), []byte("B"), []byte("678")}) // Invoke A->B for 123 checkInvoke(t, stub, [][]byte{[]byte("invoke"), []byte("A"), []byte("B"), []byte("123")}) checkQuery(t, stub, "A", "444") checkQuery(t, stub, "B", "801") // Invoke B->A for 234 checkInvoke(t, stub, [][]byte{[]byte("invoke"), []byte("B"), []byte("A"), []byte("234")}) checkQuery(t, stub, "A", "678") checkQuery(t, stub, "B", "567") checkQuery(t, stub, "A", "678") checkQuery(t, stub, "B", "567") }
执行调测
调测时在IDE中单击执行对应的测试函数即可。
图1 执行调测
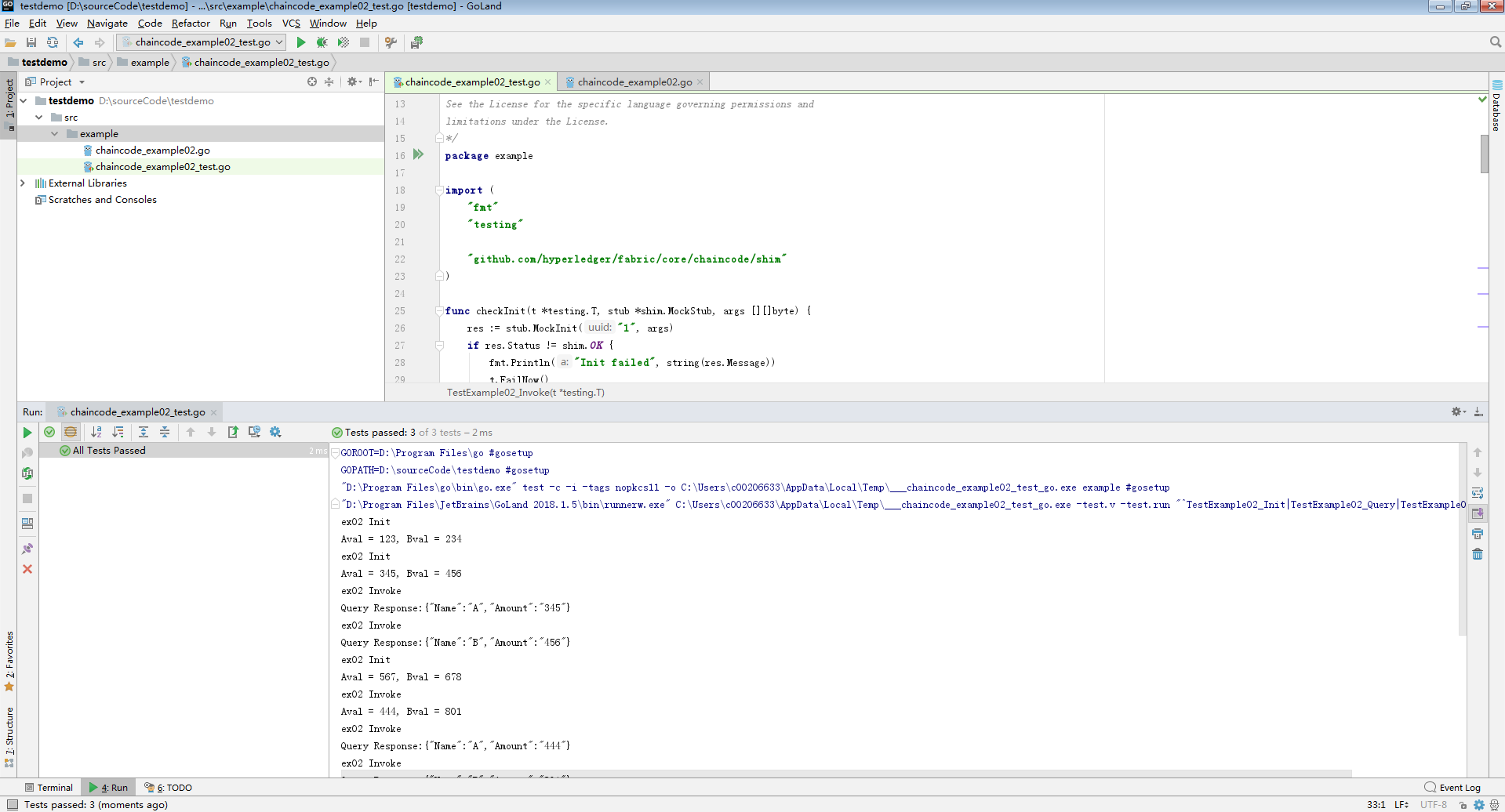
父主题: Go语言链代码开发