Using TridentSDK to Send gRPC Requests
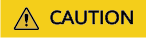
TridentSDK of the version 0.7.0 and earlier is supported. Currently, you cannot send gRPC requests using TLS. Therefore, perform node interconnection with Java reflection according to the sample code.
Download the certificate on the node details page and place the ca.crt certificate in the package under the project directory.
import io.gRPC.*; import io.gRPC.stub.MetadataUtils; import org.tron.trident.api.WalletgRPC; import org.tron.trident.api.WalletSoliditygRPC; import org.tron.trident.core.ApiWrapper; import org.tron.trident.core.exceptions.IllegalException; import org.tron.trident.core.key.KeyPair; import java.io.File; import java.io.IOException; import java.lang.reflect.Field; public class Main { public static void main(String[] args) throws IllegalException, IOException, NoSuchFieldException, IllegalAccessException { String gRPCEndpoint = "your-gRPC-endpoint"; String gRPCSolidityEndpoint = "your-gRPCsolidity-endpoint"; String credential = "your-credential"; String hexPrivateKey = "your-hex-private-key"; ApiWrapper wrapper = new ApiWrapper(gRPCEndpoint, gRPCSolidityEndpoint, hexPrivateKey, credential); // modify wallet channel through reflex ChannelCredentials creds = TlsChannelCredentials.newBuilder() .trustManager(new File("your-ca.crt-file-path")) .build(); ManagedChannel channel = gRPC.newChannelBuilder(gRPCEndpoint, creds) .build(); Metadata header = new Metadata(); Metadata.Key<String> key = Metadata.Key.of("TRON-PRO-API-KEY", Metadata.ASCII_STRING_MARSHALLER); header.put(key, credential); setField(ApiWrapper.class, wrapper, "channel", channel); setField(ApiWrapper.class, wrapper, "blockingStub", MetadataUtils.attachHeaders(WalletgRPC.newBlockingStub(channel), header)); // modify walletsolidity channel through reflex ManagedChannel channelSolidity = gRPC.newChannelBuilder(gRPCSolidityEndpoint, creds) .build(); setField(ApiWrapper.class, wrapper, "channelSolidity", channelSolidity); setField(ApiWrapper.class, wrapper, "blockingStubSolidity", MetadataUtils.attachHeaders(WalletSoliditygRPC.newBlockingStub(channelSolidity), header)); // getNowBlock & getNowBlockSolidity test System.out.println(wrapper.getNowBlock()); System.out.println(wrapper.getNowBlockSolidity()); System.out.println("finish"); } private static void setField(Class clazz, Object obj, String fieldName, Object value) throws NoSuchFieldException, IllegalAccessException { Field field = clazz.getDeclaredField(fieldName); field.setAccessible(true); field.set(obj, value); } }
Response example
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot