Changing Lite Server OS
Scenario
A Lite Server is an elastic BMS. You can use BMS to change the OS and Lite Server resources. There are three methods of changing the OS:
- Change the OS on the BMS console.
- Change the OS using BMS Go SDK.
- Change the OS by encapsulating APIs using Python.
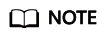
To change the OS, the following conditions must be met:
- The BMS is stopped.
- The target OS must be an IMS public image or private shared image in the region.
Changing the OS on the BMS Console
- Obtain the OS image.
The OS image is provided by Huawei Cloud. You can receive the image on the shared image page of IMS, as shown in the following figure.Figure 1 Shared image
- Change OS.
Stop the BMS corresponding to the Lite Server resource. The OS must be stopped.
Locate the target BMS in the list and choose More > Change OS in the Operation column.
Figure 2 Changing OSOn the Change OS page, select the shared image received in the previous step.
Changing the OS Using BMS Go SDK
The following is the sample code for changing the OS of a BMS using the Go language through SDK.
package main import ( "fmt" "os" "github.com/huaweicloud/huaweicloud-sdk-go-v3/core/auth/basic" bms "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/bms/v1" "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/bms/v1/model" region "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/bms/v1/region" ) func main() { // Hardcoded or plaintext AK/SK is risky. For security, encrypt your AK/SK and store them in the configuration file or environment variables. // In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, set environment variables HUAWEICLOUD_SDK_AK and HUAWEICLOUD_SDK_SK. ak := os.Getenv("HUAWEICLOUD_SDK_AK") sk := os.Getenv("HUAWEICLOUD_SDK_SK") auth := basic.NewCredentialsBuilder(). WithAk(ak). WithSk(sk). Build() client := bms.NewBmsClient( bms.BmsClientBuilder(). WithRegion(region.ValueOf("cn-north-4")). WithCredential(auth). Build()) keyname := "KeyPair-name" userdata := "aGVsbG8gd29ybGQsIHdlbGNvbWUgdG8gam9pbiB0aGUgY29uZmVyZW5jZQ==" request := &model.ChangeBaremetalServerOsRequest{ ServerId: "****input your bms instance id****", Body: &model.OsChangeReq{ OsChange: &model.OsChange{ Keyname: &keyname, Imageid: "****input your ims image id****", Metadata: &model.MetadataInstall{ UserData: &userdata, }, }, }, } response, err := client.ChangeBaremetalServerOs(request) if err == nil { fmt.Printf("%+v\n", response) } else { fmt.Println(err) } }
Changing the OS by Encapsulating APIs Using Python
The following is the sample code for changing the BMS OS using Python through APIs:
# -*- coding: UTF-8 -*- import requests import json import time import requests.packages.urllib3.exceptions from urllib3.exceptions import InsecureRequestWarning requests.packages.urllib3.disable_warnings(InsecureRequestWarning) class ServerOperation(object): ################################ IAM authentication API################################################# def __init__(self, account, password, region_name, username=None, project_id=None): """ :param username: if IAM user,here is small user, else big user :param account: account big big user :param password: account :param region_name: """ self.account = account self.username = username self.password = password self.region_name = region_name self.project_id = project_id self.ma_endpoint = "https://modelarts.{}.myhuaweicloud.com".format(region_name) self.service_endpoint = "https://bms.{}.myhuaweicloud.com".format(region_name) self.iam_endpoint = "https://iam.{}.myhuaweicloud.com".format(region_name) self.headers = {"Content-Type": "application/json", "X-Auth-Token": self.get_project_token_by_account(self.iam_endpoint)} def get_project_token_by_account(self, iam_endpoint): body = { "auth": { "identity": { "methods": [ "password" ], "password": { "user": { "name": self.username if self.username else self.account, "password": self.password, "domain": { "name": self.account } } } }, "scope": { "project": { "name": self.region_name } } } } headers = { "Content-Type": "application/json" } import json url = iam_endpoint + "/v3/auth/tokens" response = requests.post(url, headers=headers, data=json.dumps(body), verify=True) token = (response.headers['X-Subject-Token']) return token def change_os(self, server_id): url = "{}/v1/{}/baremetalservers/{}/changeos".format(self.service_endpoint, self.project_id, server_id) print(url) body = { "os-change": { "adminpass": "@Server", "imageid": "40d88eea-6e41-418a-ad6c-c177fe1876b8" } } response = requests.post(url, headers=self.headers, data=json.dumps(body), verify=False) print(json.dumps(response.json(), indent=1)) return response.json() if __name__ == '__main__': # Prepare for calling the API and initialize the authentication. server = ServerOperation(username="xxx", account="xxx", password="xxx", project_id="xxx", region_name="cn-north-4") server.change_os(server_id="0c84bb62-35bd-4e1c-ba08-a3a686bc5097")
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot