Using Watermarks to Defend Against CC Attacks
CNAD supports the sharing of watermark algorithms and keys with the service end. All packets sent by the client are embedded with watermarks, which can effectively defend against layer-4 CC attacks.
There are generally two modes of defending against UDP floods: dynamic fingerprint learning and UDP traffic limiting. The former may mistakenly learn normal service payloads as attack fingerprints, leading to false positives. The latter may block both normal and attack traffic, affecting your service.
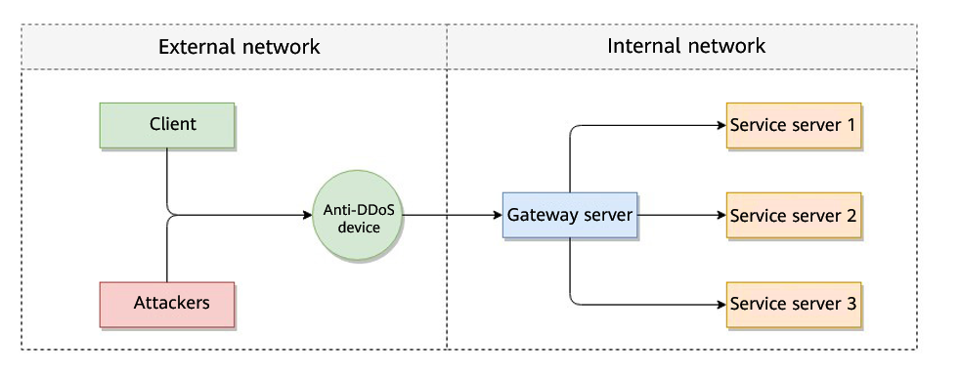
As shown in Figure 2, the Huawei cloud solution adds watermark header information to UDP packets to identify normal service packets. After receiving a UDP packet, the offline Anti-DDoS service device checks whether the UDP watermark is correct to efficiently and accurately permit normal service packets and block attack packets.
The client and Anti-DDoS device need to use the same information structure and calculation rule. The calculation rule refers to the hash factor and hash algorithm for calculating the watermark value. In this solution, the hash factor uses: the destination IP address, destination port, user identifier, and the watermark keyword; and the hash algorithm uses the CRC32.
Limitations and Constraints
- This function needs to be developed on the client. To use this function, submit a service ticket.
- Up to two keys can be configured for a watermark.
Enabling Watermark Protection
You can set a watermark protection policy on the console and configure watermarks on the client.
- Log in to the management console.
- Select a region in the upper part of the page, click
in the upper left corner of the page, and choose . The Anti-DDoS Service Center page is displayed.
- In the navigation pane on the left, choose Protection Policies page is displayed. . The
- Click Create Protection Policy.
- In the displayed dialog box, set the policy name, select an instance, and click OK.
Figure 3 Creating a policy
- In the row containing the target policy, click Set Protection Policy in the Operation column.
- In the Watermark configuration area, click Set.
Figure 4 Watermarking
- On the displayed Watermark Configuration page, click Create.
- In the Create Watermark dialog box, set watermark parameters.
Figure 5 Create Watermark
Table 1 Watermark parameters Parameter
Description
Watermark Name
Watermark name
Protocol
Currently, only UDP is supported.
Key
Keyword. Up to two keywords are supported.
Port Range
The supported port number ranges from 1 to 65535.
- Click OK.
This section uses the C language as an example to describe how to calculate and add UDP watermarks on the client. Developers can adjust the code based on the development platform.
- Initialize the CRC table:
unsigned int g_szCRCTable[256]; void CRC32TableInit(void) { unsigned int c; int n, k; for (n = 0; n < 256; n++) { c = (unsigned int)n; for (k = 0; k < 8; k++) { if (c & 1) { c = 0xedb88320 ^ (c >> 1); } else { c = c >> 1; } } g_szCRCTable[n] = c; } }
- Interface for calculating the CRC hash value. The first parameter crc is set to 0 by default.
unsigned int CRC32Hash(unsigned int crc, unsigned char* buf, int len) { unsigned int c = crc ^ 0xFFFFFFFF; int n; for (n = 0; n < len; n++) { c = g_szCRCTable[(c ^ buf[n]) & 0xFF] ^ (c >> 8); } return c ^ 0xFFFFFFFF; }
- Example Code for Calculating the Watermark Value of a Packet Figure 6 shows the watermark structure for compute
- The watermark data structure is defined as follows:
- The byte order needs to use the network byte order.
- If the service payload is less than four bytes, you can use 0s to fill it up.
typedef struct { unsigned int userId; /*User ID*/ unsigned int payload; /*Service payload*/ unsigned short destPort; /*Service destination port*/ unsigned short rsv; /*Reserved field, 2-byte filling*/ unsigned int destIp; /*Service destination IP address*/ unsigned int key; /*Watermark keyword*/ } UdpWatermarkInfo;
- The CPU hardware acceleration interface can be used to calculate the CRC hash value to improve the processing performance.
unsigned int UdpFloodWatermarkHashGet(unsigned int userId, unsigned int payload, unsigned short destPort, unsigned int destIp, unsigned int key) { UdpWatermarkInfo stWaterInfo; stWaterInfo.destIp = destIp; stWaterInfo.destPort = destPort; stWaterInfo.userId = userId; stWaterInfo.payload = payload; stWaterInfo.key = key; stWaterInfo.rsv = 0; return CRC32Hash(0, (UCHAR *)&stWaterInfo, sizeof(stWaterInfo)); }
- The watermark data structure is defined as follows:
- The packet is filled with the calculated CRC hash value according to the structure in Figure 7 and then sent out.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot