Accessing a DCS Redis Instance Through Jedis
Access a DCS Redis instance through Jedis on an ECS in the same VPC. For more information on how to use other Redis clients, visit https://redis.io/clients.
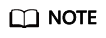
The operations described in this section apply only to single-node, master/standby, and Proxy Cluster instances. To use Jedis to connect to a Redis Cluster instance, see https://github.com/xetorthio/jedis#jedis-cluster.
Prerequisites
- The DCS Redis instance you want to access is in the Running state.
- An ECS has been created. For more information on how to create ECSs, see the Elastic Cloud Server User Guide.
- If the ECS runs the Linux OS, ensure that the GCC compilation environment has been installed on the ECS.
Procedure
- View the IP address/domain name and port number of the DCS Redis instance to be accessed.
For details, see Viewing Details of a DCS Instance.
- Log in to the ECS where you want to install Docker.
- Access the DCS instance by using Jedis.
- Obtain the source code of the Jedis client from https://github.com/xetorthio/jedis.
- Write code.
Use either of the following two methods to access a DCS Redis instance through Jedis:
- Single Jedis connection
- Jedis pool
Example code:
- Example code for a single Jedis connection
// Creating a connection in password mode String host = "192.168.0.150"; int port = 6379; String pwd = "passwd"; Jedis client = new Jedis(host, port); client.auth(pwd); client.connect(); // Run the set command String result = client.set("key-string", "Hello, Redis!"); System.out.println( String.format("set instruction execution result:%s", result) ); // Run the get command String value = client.get("key-string"); System.out.println( String.format("get command result:%s", value) ); // Creating a connection in password-free mode String host = "192.168.0.150"; int port = 6379; Jedis client = new Jedis(host, port); client.connect(); // Run the set command String result = client.set("key-string", "Hello, Redis!"); System.out.println( String.format("set command result:%s", result) ); // Run the get command String value = client.get("key-string"); System.out.println( String.format("get command result:%s", value) );
host indicates the example IP address/domain name of DCS instance and port indicates the port number of DCS instance. For details on how to obtain the IP address/domain name and port, see 1. Change the IP address and port as required. pwd indicates the password used for logging in to the chosen DCS Redis instance. This password is defined during DCS Redis instance creation. Do not hard code the plaintext password.
- Example code for a Jedis pool
// Generate configuration information of a Jedis pool String ip = "192.168.0.150"; int port = 6379; String pwd = "passwd"; GenericObjectPoolConfig config = new GenericObjectPoolConfig(); config.setTestOnBorrow(false); config.setTestOnReturn(false); config.setMaxTotal(100); config.setMaxIdle(100); config.setMaxWaitMillis(2000); JedisPool pool = new JedisPool(config, ip, port, 100000, pwd);//Generate a Jedis pool when the application is being initialized // Get a Jedis connection from the Jedis pool when a service operation occurs Jedis client = pool.getResource(); try { // Run commands String result = client.set("key-string", "Hello, Redis!"); System.out.println( String.format("set command result:%s", result) ); String value = client.get("key-string"); System.out.println( String.format("get command result:%s", value) ); } catch (Exception e) { // TODO: handle exception } finally { // Return the Jedis connection to the Jedis connection pool after the client's request is processed if (null != client) { pool.returnResource(client); } } // end of try block // Destroy the Jedis pool when the application is closed pool.destroy(); // Configure the connection pool in the password-free mode String ip = "192.168.0.150"; int port = 6379; GenericObjectPoolConfig config = new GenericObjectPoolConfig(); config.setTestOnBorrow(false); config.setTestOnReturn(false); config.setMaxTotal(100); config.setMaxIdle(100); config.setMaxWaitMillis(2000); JedisPool pool = new JedisPool(config, ip, port, 100000);//Generate a JedisPool when the application is being initialized // Get a Jedis connection from the Jedis pool when a service operation occurs Jedis client = pool.getResource(); try { // Run commands String result = client.set("key-string", "Hello, Redis!"); System.out.println( String.format("set command result:%s", result) ); String value = client.get("key-string"); System.out.println( String.format("get command result:%s", value) ); } catch (Exception e) { // TODO: handle exception } finally { // Return the Jedis connection to the Jedis connection pool after the client's request is processed if (null != client) { pool.returnResource(client); } } // end of try block // Destroy the Jedis pool when the application is closed pool.destroy();
ip indicates the IP address/domain name of DCS instance and port indicates the port number of DCS instance. For details on how to obtain the IP address/domain name and port, see 1. Change the IP address and port as required. pwd indicates the password used for logging in to the chosen DCS Redis instance. This password is defined during DCS Redis instance creation. Do not hard code the plaintext password.
- Compile code according to the readme file in the source code of the Jedis client. Run the Jedis client to access the chosen DCS Redis instance.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot