API authorization operations (authorization/authorization cancellation/application/renewal)
Function
-
Proactive API authorization: An API reviewer can initiate an API authorization request. After the API authorization is successful, the app can access the API within the validity period. API authorization includes authorization and renewal.
-
Authorization: Apps are granted the right to access APIs within the validity period.
-
Renewal: The validity period of the authorization is updated during renewal. The validity period can be extended but cannot be reduced.
-
API authorization cancellation: An API reviewer can cancel the authorization relationship between an API and an app. After the API authorization is canceled, the app can no longer call the API. Before performing this operation, reserve at least two days for the app to make preparations.
-
App authorization cancellation: The app owner can initiate a request to cancel the authorization relationship between an API and an app. After the API authorization is canceled, the app can no longer call the API. This operation requires no preparation time.
-
App authorization application: An app owner can initiate an application for an API. After the application is approved by the API reviewer, the app can access the API. The authorization grants the app the right to access the API within the validity period. The API review is required.
-
App renewal: The app owner can initiate a renewal request. The renewal will update the authorization validity period. The validity period can be extended but cannot be reduced. The renewal needs to be reviewed by the API.
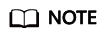
-
It is recommended that you apply for your own API authorization or renewal without approval.
-
It is recommended that the app be used to cancel the authorization. No preparation time needs to be reserved.
Calling Method
For details, see Calling APIs.
URI
POST /v1/{project_id}/service/apis/authorize/action
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
project_id |
Yes |
String |
Project ID. For details about how to obtain the project ID, see Project ID and Account ID. |
Request Parameters
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
X-Auth-Token |
Yes |
String |
User token. This parameter is mandatory when token authentication is used. You can obtain it from the value of X-Subject-Token in the response message header returned by the "Obtaining a User Token" API of the IAM service. |
workspace |
Yes |
String |
Workspace ID. For details about how to obtain the workspace ID, see Instance ID and Workspace ID. |
Dlm-Type |
No |
String |
Specifies the version type of the data service. The value can be SHARED or EXCLUSIVE. |
Content-Type |
Yes |
String |
Type (format) of the message body. This parameter is mandatory if the message body exists. If the message body does not exist, leave this parameter blank. If the request body contains Chinese characters, use charset=utf8 to specify the Chinese character set, for example, application/json;charset=utf8. |
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
api_id |
No |
String |
API ID |
instance_id |
No |
String |
Cluster ID |
app_id |
No |
String |
App ID |
apply_type |
No |
String |
Application type Enumerated values:
|
time |
No |
String |
End time. |
Response Parameters
Status code: 400
Parameter |
Type |
Description |
---|---|---|
error_code |
String |
Error code. |
error_msg |
String |
Error message. |
Example Requests
Authorize an API to an app./Cancel API authorization (by the API reviewer)./Cancel API authorization (by the app owner)./Apply for authorization./Renew the validity period.
/v1/0833a5737480d53b2f250010d01a7b88/service/apis/authorize/action { "api_id" : "47046fe7830c1be77cb0dc23bd86afa5", "instance_id" : "21398ikjdsjd9087122d4e", "app_id" : "908489209a320df61607355c57c82882", "apply_type" : "APPLY_TYPE_AUTHORIZE", "time" : "2021-01-01T10:00:00.000Z" }
Example Responses
None
SDK Sample Code
The SDK sample code is as follows.
Authorize an API to an app./Cancel API authorization (by the API reviewer)./Cancel API authorization (by the app owner)./Apply for authorization./Renew the validity period.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
package com.huaweicloud.sdk.test; import com.huaweicloud.sdk.core.auth.ICredential; import com.huaweicloud.sdk.core.auth.BasicCredentials; import com.huaweicloud.sdk.core.exception.ConnectionException; import com.huaweicloud.sdk.core.exception.RequestTimeoutException; import com.huaweicloud.sdk.core.exception.ServiceResponseException; import com.huaweicloud.sdk.dataartsstudio.v1.region.DataArtsStudioRegion; import com.huaweicloud.sdk.dataartsstudio.v1.*; import com.huaweicloud.sdk.dataartsstudio.v1.model.*; public class AuthorizeActionApiToInstanceSolution { public static void main(String[] args) { // The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. // In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment String ak = System.getenv("CLOUD_SDK_AK"); String sk = System.getenv("CLOUD_SDK_SK"); String projectId = "{project_id}"; ICredential auth = new BasicCredentials() .withProjectId(projectId) .withAk(ak) .withSk(sk); DataArtsStudioClient client = DataArtsStudioClient.newBuilder() .withCredential(auth) .withRegion(DataArtsStudioRegion.valueOf("<YOUR REGION>")) .build(); AuthorizeActionApiToInstanceRequest request = new AuthorizeActionApiToInstanceRequest(); ApiParaForAuthToInstance body = new ApiParaForAuthToInstance(); body.withTime("2021-01-01T10:00:00.000Z"); body.withApplyType(ApiParaForAuthToInstance.ApplyTypeEnum.fromValue("APPLY_TYPE_AUTHORIZE")); body.withAppId("908489209a320df61607355c57c82882"); body.withInstanceId("21398ikjdsjd9087122d4e"); body.withApiId("47046fe7830c1be77cb0dc23bd86afa5"); request.withBody(body); try { AuthorizeActionApiToInstanceResponse response = client.authorizeActionApiToInstance(request); System.out.println(response.toString()); } catch (ConnectionException e) { e.printStackTrace(); } catch (RequestTimeoutException e) { e.printStackTrace(); } catch (ServiceResponseException e) { e.printStackTrace(); System.out.println(e.getHttpStatusCode()); System.out.println(e.getRequestId()); System.out.println(e.getErrorCode()); System.out.println(e.getErrorMsg()); } } } |
Authorize an API to an app./Cancel API authorization (by the API reviewer)./Cancel API authorization (by the app owner)./Apply for authorization./Renew the validity period.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
# coding: utf-8 import os from huaweicloudsdkcore.auth.credentials import BasicCredentials from huaweicloudsdkdataartsstudio.v1.region.dataartsstudio_region import DataArtsStudioRegion from huaweicloudsdkcore.exceptions import exceptions from huaweicloudsdkdataartsstudio.v1 import * if __name__ == "__main__": # The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. # In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment ak = os.environ["CLOUD_SDK_AK"] sk = os.environ["CLOUD_SDK_SK"] projectId = "{project_id}" credentials = BasicCredentials(ak, sk, projectId) client = DataArtsStudioClient.new_builder() \ .with_credentials(credentials) \ .with_region(DataArtsStudioRegion.value_of("<YOUR REGION>")) \ .build() try: request = AuthorizeActionApiToInstanceRequest() request.body = ApiParaForAuthToInstance( time="2021-01-01T10:00:00.000Z", apply_type="APPLY_TYPE_AUTHORIZE", app_id="908489209a320df61607355c57c82882", instance_id="21398ikjdsjd9087122d4e", api_id="47046fe7830c1be77cb0dc23bd86afa5" ) response = client.authorize_action_api_to_instance(request) print(response) except exceptions.ClientRequestException as e: print(e.status_code) print(e.request_id) print(e.error_code) print(e.error_msg) |
Authorize an API to an app./Cancel API authorization (by the API reviewer)./Cancel API authorization (by the app owner)./Apply for authorization./Renew the validity period.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
package main import ( "fmt" "github.com/huaweicloud/huaweicloud-sdk-go-v3/core/auth/basic" dataartsstudio "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/dataartsstudio/v1" "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/dataartsstudio/v1/model" region "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/dataartsstudio/v1/region" ) func main() { // The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. // In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment ak := os.Getenv("CLOUD_SDK_AK") sk := os.Getenv("CLOUD_SDK_SK") projectId := "{project_id}" auth := basic.NewCredentialsBuilder(). WithAk(ak). WithSk(sk). WithProjectId(projectId). Build() client := dataartsstudio.NewDataArtsStudioClient( dataartsstudio.DataArtsStudioClientBuilder(). WithRegion(region.ValueOf("<YOUR REGION>")). WithCredential(auth). Build()) request := &model.AuthorizeActionApiToInstanceRequest{} timeApiParaForAuthToInstance:= "2021-01-01T10:00:00.000Z" applyTypeApiParaForAuthToInstance:= model.GetApiParaForAuthToInstanceApplyTypeEnum().APPLY_TYPE_AUTHORIZE appIdApiParaForAuthToInstance:= "908489209a320df61607355c57c82882" instanceIdApiParaForAuthToInstance:= "21398ikjdsjd9087122d4e" apiIdApiParaForAuthToInstance:= "47046fe7830c1be77cb0dc23bd86afa5" request.Body = &model.ApiParaForAuthToInstance{ Time: &timeApiParaForAuthToInstance, ApplyType: &applyTypeApiParaForAuthToInstance, AppId: &appIdApiParaForAuthToInstance, InstanceId: &instanceIdApiParaForAuthToInstance, ApiId: &apiIdApiParaForAuthToInstance, } response, err := client.AuthorizeActionApiToInstance(request) if err == nil { fmt.Printf("%+v\n", response) } else { fmt.Println(err) } } |
For SDK sample code of more programming languages, see the Sample Code tab in API Explorer. SDK sample code can be automatically generated.
Status Codes
Status Code |
Description |
---|---|
204 |
The API operation is successful. |
400 |
Bad request |
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot