Chaincode Structure
This section uses the Go language as an example. A chaincode is a Go file. After creating a chaincode, you can use it to develop functions.
Chaincodes can be compiled in two styles: 1.4 style (using package shim) and 2.2 style (using fabric-contract-api-go).
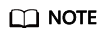
BCS supports two chaincode styles.
Chaincode Interface
- To start a chaincode, you must call the Start function in the shim package (1.4 style). The input parameter is the Chaincode interface type defined in the shim package. During chaincode development, define a structure to implement the Chaincode interface.
type Chaincode interface { Init(stub ChaincodeStubInterface) pb.Response Invoke(stub ChaincodeStubInterface) pb.Response }
- When developing chaincodes of the 2.2 style (using fabric-contract-api-go), define a structure to implement the Chaincode interface.
type Chaincode interface { Init(ctx contractapi.TransactionContextInterface, args…) error Invoke(ctx contractapi.TransactionContextInterface, args…) error }
Chaincode Structure
- The Go chaincode structure (1.4 style) is as follows:
package main //Import the required package. import ( "github.com/hyperledger/fabric/core/chaincode/shim" pb "github.com/hyperledger/fabric/protos/peer" ) //Declare a structure. type SimpleChaincode struct {} //Add the Init method to the structure. func (t *SimpleChaincode) Init(stub shim.ChaincodeStubInterface) pb.Response { //Implement the processing logic for chaincode initialization or update in this method. //stub APIs can be flexibly used during compilation. } //Add the Invoke method to the structure. func (t *SimpleChaincode) Invoke(stub shim.ChaincodeStubInterface) pb.Response { //Implement the processing logic for responding to the call or query in this method. //stub APIs can be flexibly used during compilation. } //Main function. The shim.Start() method needs to be invoked. func main() { err := shim.Start(new(SimpleChaincode)) if err != nil { fmt.Printf("Error starting Simple chaincode: %s", err) } }
- The Go chaincode structure (2.2 style) is as follows:
package main //Import the required package. import ( "github.com/hyperledger/fabric/plugins/fabric-contract-api-go/contractapi") //Declare a structure. type Chaincode struct { contractapi.Contract } //Add the Init method to the structure. func (ch * Chaincode) Init(ctx contractapi.TransactionContextInterface, args…) error { //Implement the processing logic for chaincode initialization or update in this method. } //Add the Invoke method to the structure. func (ch * Chaincode) Invoke(ctx contractapi.TransactionContextInterface, args…) error { //Implement the processing logic for responding to the call or query in this method. } //Main function func main() { cc, err := contractapi.NewChaincode(new(ABstore)) if err != nil { panic(err.Error()) } if err := cc.Start(); err != nil { fmt.Printf("Error starting ABstore chaincode: %s", err) } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot