Example
Scenarios
This section describes the sequence of calling APIs for establishing a WebSocket connection, subscribing to information, pushing information, and maintaining connection.
Service Process
The following figure shows the WebSocket connection establishment, subscription, and information push process.
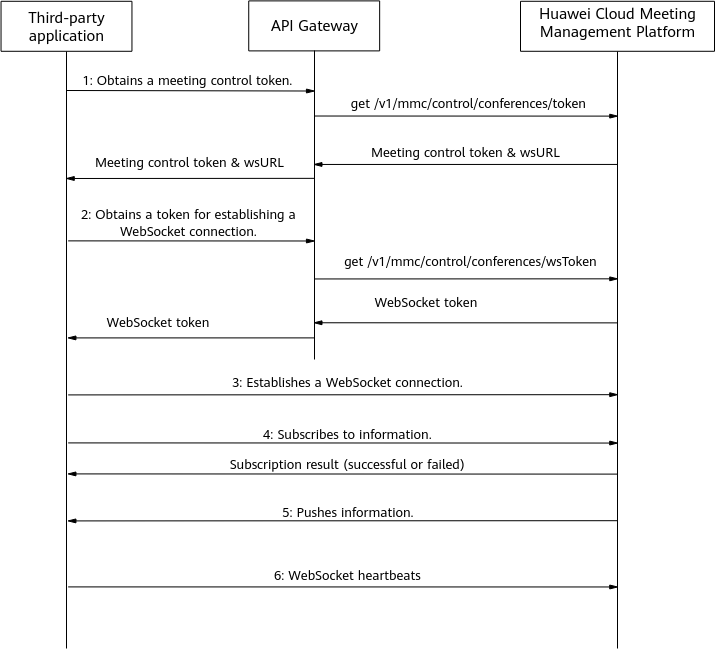
- A third-party application obtains a meeting control token and the server URL required for establishing a WebSocket connection using the meeting ID and host password. For details, see Obtaining a Meeting Control Token.
- The third-party application uses the meeting control token to obtain a token for establishing a WebSocket connection. For details, see Obtaining a Token for Establishing a WebSocket Connection.
- The third-party application establishes a WebSocket connection with the server.
- The third-party application subscribes to information that needs to be pushed by the Huawei Cloud Meeting server.
- The Huawei Cloud Meeting server pushes the subscribed-to information.
- The third-party application sends a heartbeat message at least once within 180 seconds.
Code Example 1
Code for WebSocket connection establishment, subscription, and message delivery and reception
<!DOCTYPE HTML> <html> <head> <meta charset="utf-8"> <title>WebSocket Connection Establishment and Message Delivery and Receiption</title> <script type="text/javascript"> function WebSocketTest() { if ("WebSocket" in window) { // Establish a WebSocket connection. var confID = "900964776"; var tempToken = "&tmpToken=" + "Obtaining a Token for Establishing a WebSocket Connection"; var uri = "wss://100.94.23.40:443/cms/open/websocket/confctl/increment/conn?confID=" + confID + tempToken; var ws = new WebSocket(uri); ws.onopen = function() { // WebSocket connection established. Send a subscription message. var senddata = JSON.stringify({ sequence: "000000000000000002611382273415", action: "Subscribe", data: JSON.stringify({ subscribeType: [ "ConfBasicInfoNotify", "ConfDynamicInfoNotify", "ParticipantsNotify", "AttendeesNotify", "SpeakerChangeNotify", "NetConditionNotify", "CustomMultiPicNotify", "InviteResultNotify", "InterpreterGroupNotify", "NetworkQualityNotify" ], confToken: "Obtaining a Meeting Control Token", }), }); ws.send(senddata); alert("Sending subscription message..." + senddata); }; ws.onmessage = function (evt) { var received_msg = evt.data; // alert("Data received." + received_msg); }; } else { // The browser does not support WebSocket. alert("Your browser does not support WebSocket."); } } </script> </head> <body> <div id="meeting"> <a href="javascript:WebSocketTest()">WebSocket connection establishment and subscription</a> </div> </body> </html>
Code Example 2
Code for connection establishment on a Java WebSocket client and message delivery and reception
import java.io.IOException; import java.net.URI; import java.net.URISyntaxException; import java.security.KeyManagementException; import java.security.NoSuchAlgorithmException; import java.security.cert.CertificateException; import java.security.cert.X509Certificate; import java.util.ArrayList; import java.util.Iterator; import java.util.List; import javax.net.ssl.SSLContext; import javax.net.ssl.SSLSocketFactory; import javax.net.ssl.TrustManager; import javax.net.ssl.X509TrustManager; import org.java_websocket.WebSocket; import org.java_websocket.client.WebSocketClient; import org.java_websocket.handshake.ServerHandshake; import com.google.gson.Gson; public class WebSocketClientDemo extends WebSocketClient{ public WebSocketClientDemo(String url) throws URISyntaxException { super(new URI(url)); } @Override public void onOpen(ServerHandshake shake) { System.out.println("Hand shake!"); for(Iterator<String> it = shake.iterateHttpFields(); it.hasNext();) { String key = it.next(); System.out.println(key + ":" + shake.getFieldValue(key)); } } @Override public void onMessage(String paramString) { System.out.println("receive msg: " + paramString); } @Override public void onClose(int paramInt, String paramString, Boolean paramBoolean) { System.out.println("Channel close!"); } @Override public void onError(Exception e) { System.out.println("error: " + e); } public static void main(String[] args) throws Exception{ try { // Establish a WebSocket connection. String confID = "900964776"; String tempToken = "&tmpToken=" + "Obtaining a Token for Establishing a WebSocket Connection"; String url = "wss://100.94.23.40:443/cms/open/websocket/confctl/increment/conn?confID=" + confID + tempToken; WebSocketClientDemo client = new WebSocketClientDemo(url); client.connect(); while (!client.getReadyState().equals(WebSocket.READYSTATE.OPEN)) { System.out.println("Not open yet"); Thread.sleep(100); } System.out.println("WebSocket channel connected!"); // WebSocket connection established. Send a subscription message. Gson gson = new Gson(); List<SubscribeType> subscribeTypes = new ArrayList<>(); subscribeTypes.add(SubscribeType.ConfBasicInfoNotify); subscribeTypes.add(SubscribeType.ConfDynamicInfoNotify); subscribeTypes.add(SubscribeType.ParticipantsNotify); subscribeTypes.add(SubscribeType.AttendeesNotify); subscribeTypes.add(SubscribeType.SpeakerChangeNotify); subscribeTypes.add(SubscribeType.NetConditionNotify); subscribeTypes.add(SubscribeType.InviteResultNotify); SubscribeReq subscribeReq = SubscribeReq.builder() .subscribeType(subscribeTypes) .confToken(Obtaining a Meeting Control Token) .build(); SubscribeMsgFrame subscribeMsgFrame = SubscribeMsgFrame.builder() .action("Subscribe") .sequence("000000000000000002611382271289") .data(gson.toJson(subscribeReq)) .build(); String jsonStr = gson.toJson(subscribeMsgFrame); client.send(jsonStr); } catch (URISyntaxException e) { e.printStackTrace(); } } } Connection establishment and message delivery and reception on a Java WebSocket client SubscribeReq.java ------ import java.util.List; import lombok.Builder; import lombok.Getter; import lombok.Setter; @Getter @Setter @Builder public class SubscribeReq { /** * Subscription type */ List<SubscribeType> subscribeType; /** * Meeting token */ String confToken; } SubscribeMsgFrame .java ------ import lombok.Builder; import lombok.Getter; import lombok.Setter; @Getter @Setter @Builder public class SubscribeMsgFrame { /** * Message type */ String action; /** * Random sequence number of a message */ String sequence; /** * Message body */ String data; }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot