Stream Object (Stream)
This section describes the stream APIs of the Web SDK.
API |
Description |
---|---|
Plays audio and video streams. |
|
Stops playing a video stream. |
|
Resumes audio and video playback. |
|
Disables audio and video. |
|
Mutes an audio track. |
|
Disables a video track. |
|
Unmutes an audio track. |
|
Enables a video track. |
|
Obtains the unique ID of a stream. |
|
Obtains the ID of the user to which a stream belongs. |
|
Sets an audio output device. |
|
Sets audio volume. |
|
Obtains the real-time audio volume level. |
|
Indicates whether a stream contains an audio track. |
|
Indicates whether a stream contains a video track. |
|
Obtains the audio track of a stream. |
|
Obtains the video track of a stream. |
|
Obtains the stream type. |
|
Registers the callback for a stream object event. |
|
Deregisters the callback for a stream object event. |
|
Obtains stream information. |
play
async play(elementId: string, options?: Options): Promise<void>
[Function Description]
Plays audio and video streams. This API automatically creates a <audio> or <video> tag and plays audio and video with the specified tag. The tag is added to the div container named elementId on the page.
[Request Parameters]
- elementId: (mandatory) HTML <div> tag ID. The type is string.
- options: (optional) playback option. The type is Options.
Options is defined as:
- objectFit: (optional) string type. The default value is contain for remote sharing streams and cover for other streams. The following enumerated values are supported:
- contain: preferentially ensures that all video content is displayed. The video is scaled proportionally until one side of the video window is aligned with the window border. If the video size is inconsistent with the display window size, when the aspect ratio is locked and the video is zoomed in or out to fill the window, a black bar is displayed around the zoomed-in or zoomed-out video.
- cover: preferentially ensures that the window is filled. The video is scaled proportionally until the entire window is filled with video. If the video size is inconsistent with the display window size, the video stream will be cropped or the image will be stretched to fill the display window.
- fill: The window is filled with video. If the aspect ratio of the video does not match the window, the video will be stretched to fit the window.
- muted: (optional) The type is Boolean. true indicates muted; false indicates unmuted. The default value is false.
- resolutionId: (optional) The type is string. In the dual-stream scenario, specify the resolution ID of the video to be played. If the resolution ID is not specified, the video with the highest resolution is selected by default. This parameter is added in version 1.8.0.
- objectFit: (optional) string type. The default value is contain for remote sharing streams and cover for other streams. The following enumerated values are supported:
[Response Parameters]
Promise<void>: returns a Promise object.
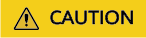
- Due to the restriction of the browser's automatic playback policy, when play() returns an error, the user needs to manually trigger the page control to call the resume API to resume the playback.
- To play local streams, you need to set muted to true (muted) to prevent the played sound from being captured by the microphone.
- On the app, a resolution corresponds to an audio/video playback window. The audio in the stream is common to all resolutions.
stop
stop(option?: StopOption): void
[Function Description]
Stops playing audio and video streams.
[Request Parameters]
option: (optional) option for stopping the playback. The type is StopOption. If this parameter is not passed, the audio of the stream and the videos of all resolutions are stopped.
StopOption is defined as: {
- audio: (optional) whether to stop the audio stream. The type is Boolean. The default value is false.
- video: (optional) whether to stop the video stream. The type is Boolean. The default value is false.
- resolutionIds: (optional) The type is string[]. It is valid when video is set to true. It is used to stop the video with a specific resolution ID. If resolutionIds is not passed, videos of all resolutions are stopped by default.
}
[Response Parameters]
None
resume
async resume(option?: ResumeOption): Promise<void>
[Function Description]
- In some browsers, if the div container transferred to play() is moved, the audio and video player may enter the PAUSED state. Therefore, this API needs to be called to resume the playback.
- Due to the restriction of the browser's automatic playback policy, when play() returns an error, the user needs to manually call this API to resume the playback.
[Request Parameters]
option: (optional) option for resuming the playback. The type is ResumeOption. If this parameter is not passed, the audio of the stream and videos of all resolutions are resumed.
ResumeOption is defined as:
- audio: (optional) whether to resume the audio stream. The type is Boolean. The default value is false.
- video: (optional) whether to resume the video stream. The type is Boolean. The default value is false.
- resolutionIds: (optional) The type is string. It is valid only when video is set to true. It is used to resume the video with a specific resolution ID. If resolutionIds is passed, videos of all resolutions are resumed by default.
}
[Response Parameters]
Promise<void>: returns a Promise object.
close
close(option?: CloseOption): void
[Function Description]
Closes the audio and video playback. For local streams, this method will also disable audio and video capture and release device resources.
[Request Parameters]
option: (optional) option for disabling the audio and video. The type is CloseOption. If option is not set, audio and videos of all resolutions are disabled.
CloseOption is defined as:
- audio: (optional) The type is Boolean. It indicates whether to disable the audio stream. The default value is false.
- video: (optional) The type is Boolean. It indicates whether to disable the video stream. The default value is false.
- resolutionIds: (optional) The type is string[]. It is valid when video is set to true. It is used to disable the video with a specific resolution ID. If resolutionIds is not passed, videos of all resolutions are disabled by default.
}
[Response Parameters]
None
muteAudio
muteAudio(): boolean
[Function Description]
Mutes an audio track.
[Request Parameters]
None
[Response Parameters]
The type is Boolean. true indicates that the audio track is successfully muted; false indicates that the audio track fails to be muted.
muteVideo
muteVideo(): boolean
[Function Description]
Disables a video track.
[Request Parameters]
None
[Response Parameters]
The type is Boolean. true indicates that the video track is successfully disabled; false indicates that the video track fails to be disabled.
unmuteAudio
unmuteAudio(): boolean
[Function Description]
Unmutes an audio track.
[Request Parameters]
None
[Response Parameters]
The type is Boolean. true indicates that the audio track is successfully unmuted; false indicates that the audio track fails to be unmuted.
unmuteVideo
unmuteVideo(): boolean
[Function Description]
Enables a video track.
[Request Parameters]
None
[Response Parameters]
The type is Boolean. true indicates that the video track is successfully enabled; false indicates that the video track fails to be enabled.
getId
getId(): string
[Function Description]
Obtains the unique ID of a stream. If a local stream is used, a valid ID can be obtained only after the stream is published.
[Request Parameters]
None
[Response Parameters]
Unique ID of a stream. The type is string.
getUserId
getUserId(): string
[Function Description]
Obtains the ID of the user to which a stream belongs. For a local stream, if this parameter is not set in the input parameter StreamConfig of createStream, undefined is returned.
[Request Parameters]
None
[Response Parameters]
ID of the user to which the stream belongs. The type is string.
setAudioOutput
setAudioOutput(deviceId: string): Promise<void>
[Function Description]
Sets an audio output device.
[Request Parameters]
deviceId: (mandatory) ID of an audio output device. The type is string.
[Response Parameters]
Promise<void>: returns a Promise object.
setAudioVolume
setAudioVolume(volume: number): void
[Function Description]
Sets audio volume.
[Request Parameters]
volume: (mandatory) volume. The type is number. The value range is [0,100].
[Response Parameters]
None
getAudioLevel
getAudioLevel(): number
[Function Description]
Obtains the real-time volume level.
[Request Parameters]
None
[Response Parameters]
The type is number. The return value range is (0, 1). Generally, a value greater than 0.1 indicates that the user is speaking.
hasAudio
hasAudio(): boolean
[Function Description]
Indicates whether a stream contains an audio track.
[Request Parameters]
None
[Response Parameters]
The type is Boolean. true indicates contained; false indicates not contained.
hasVideo
hasVideo(): boolean
[Function Description]
Indicates whether a stream contains a video track.
[Request Parameters]
None
[Response Parameters]
The type is Boolean. true indicates contained; false indicates not contained.
getAudioTrack
getAudioTrack(): MediaStreamTrack
[Function Description]
Obtains an audio track.
[Request Parameters]
None
[Response Parameters]
The type is MediaStreamTrack. For details about the MediaStreamTrack type, see MediaStreamTrack.
getVideoTrack
getVideoTrack(resolutionId?:string): MediaStreamTrack
[Function Description]
Obtains a video track.
[Request Parameters]
resolutionId: (optional) The type is string. Specify the resolution ID. If the resolution ID is not specified, the video with the highest resolution is selected by default.
[Response Parameters]
The type is MediaStreamTrack. For details about the MediaStreamTrack type, see MediaStreamTrack.
getType
getType(): string
[Function Description]
Obtains the stream type. This API is used to determine whether a stream is video or presentation. Generally, a presentation stream is a screen sharing stream.
[Request Parameters]
None
[Response Parameters]
The type is string. local: local stream; main: remote video stream; auxiliary: remote presentation stream.
on
on(event: string, handler: function): void
[Function Description]
Registers the callback for a stream object event.
- event: (mandatory) event name. The type is string. For details about the event list, see RTCStreamEvent.
- handler: (mandatory) event processing method. The type is function.
[Response Parameters]
None
off
off(event: string, handler: function): void
[Function Description]
Deregisters the callback for a stream object event.
- event: (mandatory) event name. The type is string. For details about the event list, see RTCStreamEvent.
- handler: (mandatory) event processing method. The type is function.
[Response Parameters]
None
getStreamInfo
getStreamInfo(): StreamInfo
[Function Description]
Obtains information about initialized local streams or received remote streams.
[Request Parameters]
None
[Response Parameters]
StreamInfo is defined as:
- videoProfiles: RTCVideoProfileInfo[] type.
- audioProfile: RTCAudioProfile type.
}
RTCVideoProfileInfo is defined as:
- resolutionId: (mandatory) resolution ID. The type is string.
- hasTrack: whether the video of this resolution has a playable track. The type is Boolean.
- width: width of the resolution, in pixels. The type is number.
- height: height of the resolution, in pixels. The type is number.
- frameRate: video frame rate, in fps. The type is number.
- minBitrate: minimum video bit rate, in bit/s. The type is number.
- maxBitrate: maximum video bit rate, in bit/s. The type is number.
}
RTCAudioProfile is defined as: {
- sampleRate: audio sampling ratio. The type is number.
- channelCount: number of audio channels. The type is number.
- bitrate: audio bitrate, in bit/s. The type is number.
}
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot