Deleting Objects
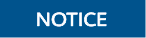
If you have any questions during development, post them on the Issues page of GitHub.

Exercise caution when performing this operation. If the versioning function is disabled for the bucket where the object is located, the object cannot be restored after being deleted.
Deleting a Single Object
You can use delete_object to delete a single object. The parameters are described as follows:
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
option |
The context of the bucket. For details, see Configuring option. |
Mandatory |
Bucket parameter |
object_info |
obs_object_info * |
Mandatory |
Object name and version number. For non-multi-version objects, set version to 0. |
handler |
obs_response_handler * |
Mandatory |
Callback function |
callback_data |
void * |
Optional |
Callback data |
Sample code:
static void test_delete_object(char *key, char *version_id, char *bucket_name) { obs_status ret_status = OBS_STATUS_BUTT; // Create and initialize object information. obs_object_info object_info; memset(&object_info, 0, sizeof(obs_object_info)); object_info.key = key; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler resqonseHandler = { &response_properties_callback, &response_complete_callback }; // Delete an object. delete_object(&option,&object_info,&resqonseHandler, &ret_status); if (OBS_STATUS_OK == ret_status) { printf("delete object successfully. \n"); } else { printf("delete object failed(%s).\n", obs_get_status_name(ret_status)); } }
Batch Deleting Objects
You can call batch_delete_objects to delete multiple objects in batches.
A maximum of 1000 objects can be deleted each time. Two response modes are supported: verbose (detailed) and quiet (brief).
- In verbose mode (default mode), the returned response includes the deletion result of each requested object.
- In quiet mode, the returned response includes only results of objects failed to be deleted.
The parameters are as follows:
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
option |
The context of the bucket. For details, see Configuring option. |
Mandatory |
Bucket parameter |
object_info |
obs_object_info * |
Mandatory |
Name and version number of the object to be deleted. For non-multi-version objects, set version to 0. |
delobj |
obs_delete_object_info* |
Mandatory |
The number of objects to be deleted. Specifies the quiet mode. |
put_properties |
obs_put_properties * |
Optional |
Sets the verification properties of the object to be deleted. |
handler |
obs_delete_object_handler* |
Mandatory |
Callback function |
callback_data |
void * |
Optional |
Callback data |
Sample code:
static void test_batch_delete_objects() { obs_status ret_status = OBS_STATUS_BUTT; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Initialize information of the objects to be deleted. obs_object_info objectinfo[100]; objectinfo[0].key = "obj1"; objectinfo[0].version_id = "versionid1"; objectinfo[1].key = "obj2"; objectinfo[1].version_id = "versionid2"; obs_delete_object_info delobj; memset_s(&delobj,sizeof(obs_delete_object_info),0,sizeof(obs_delete_object_info)); delobj.keys_number = 2; // Set response callback function. obs_delete_object_handler handler = { {&response_properties_callback, &response_complete_callback}, &delete_objects_data_callback }; // Delete objects in batches. batch_delete_objects(&option, objectinfo, &delobj, 0, &handler, &ret_status); if (OBS_STATUS_OK == ret_status) { printf("test batch_delete_objects successfully. \n"); } else { printf("test batch_delete_objects faied(%s).\n", obs_get_status_name(ret_status)); } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot