Conditions
A condition can be a single judgment statement or a nested logical operator.
The judgment statement is used to determine whether a specific value meets a specific requirement. It returns a Boolean value and its format is as follows:
{ "value": "...", "comparator": "...", "pattern": "..." }
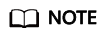
- value can be a constant or an expression. Its value type depends on the selected comparison operator. Example: true, 1, "hello", or "${resource().properties.metadata}"
- comparator: specifies the comparison operator.
- pattern can be a constant or an expression.
The following comparators are supported:
- equals compares whether value is equal to pattern. value can be a string, an integer, or a Boolean, so is pattern.
- notEquals: Its result is opposite to the equals result.
- equalsIgnoreCase compares whether value is equal to pattern in case-insensitive mode. value must be a string, so is pattern.
- like performs fuzzy match of value and pattern. You can add an asterisk (*) to pattern to match zero or multiple random characters, or add a question mark (?) to pattern to match any random character. value must be a string, so is pattern.
- notLike: Its result is opposite to the like result.
- likeIgnoreCase performs fuzzy match of value and pattern in case-insensitive mode. value must be a string, so is pattern.
- contains determines whether pattern is a substring of value. value must be a string, so is pattern.
- notContains: Its result is opposite to the contains result.
- in determines whether value is in pattern. Pattern must be an array. value can be a string or an integer.
- notIn: Its result is opposite to the in result.
- containsKey determines whether value contains the key-value pattern. value must be an object. pattern must be a string.
- notContainsKey: Its result is opposite to the containsKey result.
- less determines whether value is smaller than pattern. value can be a string or an integer, so is pattern.
- lessOrEquals determines whether value is smaller than or equal to pattern. value can be a string or an integer, so is pattern.
- greater determines whether value is greater than pattern. value can be a string or an integer, so is pattern.
- greaterOrEquals determines whether value is greater than or equal to pattern. value can be a string or an integer, so is pattern.
{ "not": { "anyOf": [ { "value": "${resource().properties.metadata}", "comparator": "notContainsKey", "pattern": "systemEncrypted" }, { "value": "${resource().properties.metadata.systemEncrypted}", "comparator": "equals", "pattern": "0" } ] } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot