SMTP Code Calling Example (Java)
This section uses Java code as an example, for example, Code Calling Sample. If the Java code does not meet the requirements, see Single Connection (Sending Multiple Times) and Example Email with an Attachment.
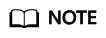
JDK 1.8 or later is recommended.
Prerequisites
You have obtained the sender address from the Email Message console.
You have obtained the SMTP account and password from the KooMessage operations personnel.
Supported SMTP Server and Port
private static final String SMTP_HOST = "sg-smtp.koomessage.7k2k1.cn"; private static final String SMTP_PORT = "465";
Code Calling Sample
import javax.mail.*; import javax.mail.internet.InternetAddress; import javax.mail.internet.MimeMessage; import java.io.UnsupportedEncodingException; import java.nio.charset.StandardCharsets; import java.util.Properties; public class SampleMail { private static final String SMTP_HOST = "sg-smtp.koomessage.7k2k1.cn"; private static final String SMTP_PORT = "465"; public static void main(String[] args) { // Configure the environment attributes for sending emails. final Properties props = new Properties(); // Identity authentication is required for sending emails through SMTP. props.put("mail.smtp.auth", "true"); props.put("mail.smtp.host", SMTP_HOST); // SMTP uses SSL to ensure security. If you do not want to import the KM certificate, add downstream code. props.put("mail.smtp.ssl.trust", SMTP_HOST); // If SSL is used, delete the configuration of port 25 and perform the following configuration: props.put("mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory"); props.put("mail.smtp.socketFactory.port", SMTP_PORT); props.put("mail.smtp.port", SMTP_PORT); // SMTP account of the sender. Replace username with the SMTP account provided by KooMessage. props.put("mail.user", "username"); // Password required for accessing the SMTP service (set when selecting the sender address on the console). Replace password with the SMTP password provided by KooMessage. props.put("mail.password", "password"); props.setProperty("mail.smtp.socketFactory.fallback", "false"); props.put("mail.smtp.ssl.enable", "true"); //props.put("mail.smtp.starttls.enable","true"); // Construct authorization information for SMTP authentication. Authenticator authenticator = new Authenticator() { @Override protected PasswordAuthentication getPasswordAuthentication() { // Username and password. String userName = props.getProperty("mail.user"); String password = props.getProperty("mail.password"); return new PasswordAuthentication(userName, password); } }; // Use the environment attributes and authorization information to create an email session. Session mailSession = Session.getInstance(props, authenticator); // mailSession.setDebug(true); // UUID uuid = UUID.randomUUID(); // final String messageIDValue = "<" + uuid.toString() + ">"; // Create an email message. MimeMessage message = new MimeMessage(mailSession) { //@Override //protected void updateMessageID() throws MessagingException { // Customize Message-ID. //setHeader("Message-ID", messageIDValue); //} }; try { // Set the email address and name of the sender. Enter the sender address configured on the console, for example, test@qq.com. The value must be the same as that of mail.user. The name can be customized. InternetAddress from = new InternetAddress("test@qq.com", "test"); message.setFrom(from); //(Optional) Set the reply address. //Address[] a = new Address[1]; //a[0] = new InternetAddress("***"); //message.setReplyTo(a); // Set the recipient email address, for example, yyy@yyy.com. InternetAddress to = new InternetAddress("xxx@xxx.com"); message.setRecipient(MimeMessage.RecipientType.TO, to); // If the email is sent to multiple recipients at the same time, replace the preceding two lines with the following (because of some restrictions of the receiving system, try to send the email to one recipient at a time. In addition, a maximum of 50 recipients are allowed at a time.): //InternetAddress[] adds = new InternetAddress[2]; //adds[0] = new InternetAddress("xxx@xxx.com"); //adds[1] = new InternetAddress("xxx@xxx.com"); //message.setRecipients(Message.RecipientType.TO, adds); // Set the email title. message.setSubject("Test email"); message.setHeader("Content-Transfer-Encoding", "base64"); // Set the email body type: text/plain or text/html message.setContent("<!DOCTYPE html>\n<html>\n<head>\n<meta charset=\"utf-8\">\n<title>hello world</title>\n</head>\n<body>\n " + "<h1>My first title</h1>\n <p>My first paragraph.</p>\n</body>\n</html>", "text/html;charset=UTF-8"); // Send the email. Transport.send(message); } catch (MessagingException | UnsupportedEncodingException e) { String err = e.getMessage(); err = new String(err.getBytes(StandardCharsets.ISO_8859_1), StandardCharsets.UTF_8); System.out.println(err); } } }
Single Connection (Sending Multiple Times)
If you use a single connection and send emails for multiple times, you can refer to the following example to improve the sending performance.
import jakarta.mail.Authenticator; import jakarta.mail.MessagingException; import jakarta.mail.PasswordAuthentication; import jakarta.mail.Session; import jakarta.mail.Transport; import jakarta.mail.internet.InternetAddress; import jakarta.mail.internet.MimeMessage; import java.io.UnsupportedEncodingException; import java.nio.charset.StandardCharsets; import java.util.Arrays; import java.util.List; import java.util.Properties; public class SampleMail { private static final String SMTP_HOST = "sg-smtp.koomessage.7k2k1.cn"; private static final String SMTP_PORT = "465"; public static void main(String[] args) { // Configure the environment attributes for sending emails. final Properties props = new Properties(); // Identity authentication is required for sending emails through SMTP. props.put("mail.smtp.auth", "true"); props.put("mail.smtp.host", SMTP_HOST); // SMTP uses SSL to ensure security. If you do not want to import the KM certificate, add downstream code. props.put("mail.smtp.ssl.trust", SMTP_HOST); // If SSL is used, delete the configuration of port 25 and perform the following configuration: props.put("mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory"); props.put("mail.smtp.socketFactory.port", SMTP_PORT); props.put("mail.smtp.port", SMTP_PORT); // SMTP account of the sender. Replace username with the SMTP account provided by KooMessage. props.put("mail.user", "username"); // Password required for accessing the SMTP service (set when selecting the sender address on the console). Replace password with the SMTP password provided by KooMessage. props.put("mail.password", "password"); props.setProperty("mail.smtp.socketFactory.fallback", "false"); props.put("mail.smtp.ssl.enable", "true"); //props.put("mail.smtp.starttls.enable","true"); // Construct authorization information for SMTP authentication. Authenticator authenticator = new Authenticator() { @Override protected PasswordAuthentication getPasswordAuthentication() { // Username and password. String userName = props.getProperty("mail.user"); String password = props.getProperty("mail.password"); return new PasswordAuthentication(userName, password); } }; // Use the environment attributes and authorization information to create an email session. Session mailSession = Session.getInstance(props, authenticator); // mailSession.setDebug(true); // UUID uuid = UUID.randomUUID(); // final String messageIDValue = "<" + uuid.toString() + ">"; // Create an email message. MimeMessage message = new MimeMessage(mailSession) { //@Override //protected void updateMessageID() throws MessagingException { // Customize Message-ID. //setHeader("Message-ID", messageIDValue); //} }; Transport transport = null; try { // Set the email address and name of the sender. Enter the sender address configured on the console, for example, test@qq.com. The value must be the same as that of mail.user. The name can be customized. transport = mailSession.getTransport(); transport.connect(props.getProperty("mail.user"), props.getProperty("mail.password")); List<String> toList = Arrays.asList("xxx1@xx.com", "xxx2@xx.com", "xxx3@xx.com"); for (String to : toList) { InternetAddress from = new InternetAddress("jwl6C@kmemailtest.cn", "test"); message.setFrom(from); //(Optional) Set the reply address. //Address[] a = new Address[1]; //a[0] = new InternetAddress("***"); //message.setReplyTo(a); // Set the recipient email address, for example, yyy@yyy.com. InternetAddress toAddress = new InternetAddress(to); message.setRecipient(MimeMessage.RecipientType.TO, toAddress); // If the email is sent to multiple recipients at the same time, replace the preceding two lines with the following (because of some restrictions of the receiving system, try to send the email to one recipient at a time. In addition, a maximum of 50 recipients are allowed at a time.): //InternetAddress[] adds = new InternetAddress[2]; //adds[0] = new InternetAddress("xxx@xxx.com"); //adds[1] = new InternetAddress("xxx@xxx.com"); //message.setRecipients(Message.RecipientType.TO, adds); // Set the email title. message.setSubject ("Test email"); message.setHeader("Content-Transfer-Encoding", "base64"); // Set the email body type: text/plain or text/html message.setContent( "<!DOCTYPE html>\n<html>\n<head>\n<meta charset=\"utf-8\">\n<title>hello world</title>\n</head>\n<body>\n " + "<h1>My first title</h1>\n <p>My first paragraph.</p>\n</body>\n</html>", "text/html;charset=UTF-8"); // Send the email. transport.sendMessage(message, message.getAllRecipients()); } } catch (MessagingException | UnsupportedEncodingException e) { String err = e.getMessage(); err = new String(err.getBytes(StandardCharsets.ISO_8859_1), StandardCharsets.UTF_8); System.out.println(err); } finally { if (transport != null) { try { transport.close(); } catch (MessagingException e) { String err = e.getMessage(); err = new String(err.getBytes(StandardCharsets.ISO_8859_1), StandardCharsets.UTF_8); System.out.println(err); } } } } }
Example Email with an Attachment
import jakarta.activation.DataHandler; import jakarta.activation.DataSource; import jakarta.activation.FileDataSource; import jakarta.mail.Authenticator; import jakarta.mail.Message; import jakarta.mail.MessagingException; import jakarta.mail.Multipart; import jakarta.mail.PasswordAuthentication; import jakarta.mail.Session; import jakarta.mail.Transport; import jakarta.mail.internet.InternetAddress; import jakarta.mail.internet.MimeBodyPart; import jakarta.mail.internet.MimeMessage; import jakarta.mail.internet.MimeMultipart; import java.util.Properties; public class SendEmailWithAttachment { private static final String SMTP_HOST = "sg-smtp.koomessage.7k2k1.cn"; private static final String SMTP_PORT = "465"; public static void main(String[] args) { // Configure the environment attributes for sending emails. final Properties props = new Properties(); // Identity authentication is required for sending emails through SMTP. props.put("mail.smtp.auth", "true"); props.put("mail.smtp.host", SMTP_HOST); // If SSL is used, delete the configuration of port 25 and perform the following configuration: props.put("mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory"); props.put("mail.smtp.socketFactory.port", SMTP_PORT); props.put("mail.smtp.port", SMTP_PORT); // SMTP account of the sender. Replace username with the SMTP account provided by KooMessage. props.put("mail.user", "username"); // Password required for accessing the SMTP service (set when selecting the sender address on the console). Replace password with the SMTP password provided by KooMessage. props.put("mail.password", "password"); props.setProperty("mail.smtp.socketFactory.fallback", "false"); props.put("mail.smtp.ssl.enable", "true"); //props.put("mail.smtp.starttls.enable","true"); // Construct authorization information for SMTP authentication. Authenticator authenticator = new Authenticator() { @Override protected PasswordAuthentication getPasswordAuthentication() { // Username and password. String userName = props.getProperty("mail.user"); String password = props.getProperty("mail.password"); return new PasswordAuthentication(userName, password); } }; // Use the environment attributes and authorization information to create an email session. Session mailSession = Session.getInstance(props, authenticator); // UUID uuid = UUID.randomUUID(); // final String messageIDValue = "<" + uuid.toString() + ">"; // Create an email message. MimeMessage message = new MimeMessage(mailSession) { //@Override //protected void updateMessageID() throws MessagingException { // Customize Message-ID. //setHeader("Message-ID", messageIDValue); //} }; try { // Create a message. message.setFrom(new InternetAddress("xxxx@xx")); message.setRecipients(Message.RecipientType.TO, InternetAddress.parse("xxxx@xx")); message.setSubject("Subject: Test Email with Attachment"); // Create a message body. MimeBodyPart textPart = new MimeBodyPart(); textPart.setText("This is the email body"); // Create an attachment. MimeBodyPart attachmentPart = new MimeBodyPart(); DataSource source = new FileDataSource("path/to/attachment.txt"); // Attachment path. attachmentPart.setDataHandler(new DataHandler(source)); attachmentPart.setFileName("attachment.txt"); // Attachment name. // Combine the message body and attachment. Multipart multipart = new MimeMultipart(); multipart.addBodyPart(textPart); multipart.addBodyPart(attachmentPart); message.setContent(multipart); // Send the email. Transport.send(message); System.out.println("Email sent successfully!"); } catch (MessagingException e) { e.printStackTrace(); } } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot