Development Example
This section uses the C language as an example to describe how to calculate and add UDP watermarks on the client. Developers can adjust the code based on the development platform.
Example Code for Calculating the CRC Hash Value
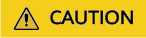
The CRC algorithm in this section uses CRC-32-IEEE 802.3.
- Initialize the CRC table:
unsigned int g_szCRCTable[256]; void CRC32TableInit(void) { unsigned int c; int n, k; for (n = 0; n < 256; n++) { c = (unsigned int)n; for (k = 0; k < 8; k++) { if (c & 1) { c = 0xedb88320 ^ (c >> 1); } else { c = c >> 1; } } g_szCRCTable[n] = c; } }
- Interface for calculating the CRC hash value. The first parameter crc is set to 0 by default.
unsigned int CRC32Hash(unsigned int crc, unsigned char* buf, int len) { unsigned int c = crc ^ 0xFFFFFFFF; int n; for (n = 0; n < len; n++) { c = g_szCRCTable[(c ^ buf[n]) & 0xFF] ^ (c >> 8); } return c ^ 0xFFFFFFFF; }
Example Code for Calculating the Watermark Value of a Packet
Figure 1 shows the watermark structure for compute
- The watermark data structure is defined as follows:
typedef struct { unsigned int userId; /*User ID*/ unsigned int payload; /*Service payload*/ unsigned short destPort; /*Service destination port*/ unsigned short rsv; /*Reserved field, 2-byte filling*/ unsigned int destIp; /*Service destination IP address*/ unsigned int key; /*Watermark keyword*/ } UdpWatermarkInfo;
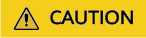
- The byte order needs to use the network byte order.
- If the service payload is less than four bytes, you can use 0s to fill it up.
- The CPU hardware acceleration interface can be used to calculate the CRC hash value to improve the processing performance.
unsigned int UdpFloodWatermarkHashGet(unsigned int userId, unsigned int payload, unsigned short destPort, unsigned int destIp, unsigned int key) { UdpWatermarkInfo stWaterInfo; stWaterInfo.destIp = destIp; stWaterInfo.destPort = destPort; stWaterInfo.userId = userId; stWaterInfo.payload = payload; stWaterInfo.key = key; stWaterInfo.rsv = 0; return CRC32Hash(0, (UCHAR *)&stWaterInfo, sizeof(stWaterInfo)); }
Filling UDP Watermarks
The packet is filled with the calculated CRC hash value according to the structure in Figure 2 and then sent out.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot