Help Center/
Object Storage Service/
SDK Reference/
C/
Server-Side Encryption/
Example of Encryption
Updated on 2024-12-03 GMT+08:00
Example of Encryption
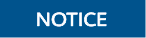
If you have any questions during development, post them on the Issues page of GitHub.
Encrypting an Object to Be Uploaded
Sample code:
static void test_put_object_by_aes_encrypt() { // Buffer to be uploaded char *buffer = "11111111"; // Length of the buffer to be uploaded int buffer_size = strlen(buffer); // Name of an object to be uploaded char *key = "put_buffer_aes"; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); option.bucket_options.protocol = OBS_PROTOCOL_HTTPS; // Initialize the properties of an object to be uploaded. obs_put_properties put_properties; init_put_properties(&put_properties); // Initialize the structure for storing uploaded data. put_buffer_object_callback_data data; memset(&data, 0, sizeof(put_buffer_object_callback_data)); data.put_buffer = buffer; data.buffer_size = buffer_size; // Server-side encryption server_side_encryption_params encryption_params; memset(&encryption_params, 0, sizeof(server_side_encryption_params)); encryption_params.ssec_customer_algorithm = "AES256"; encryption_params.ssec_customer_key = "K7QkYpBkM5+hcs27fsNkUnNVaobncnLht/rCB2o/9Cw="; // Set callback function. obs_put_object_handler putobjectHandler = { { &response_properties_callback, &put_buffer_complete_callback }, &put_buffer_data_callback }; put_object(&option, key, buffer_size, &put_properties, &encryption_params,&putobjectHandler,&data); if (OBS_STATUS_OK == data.ret_status) { printf("put object by_aes_encrypt successfully. \n"); } else { printf("put object by_aes_encrypt encryption failed(%s).\n", obs_get_status_name(data.ret_status)); } }
Decrypting a To-Be-Download Object
Sample code:
static void test_get_object_by_aes_encrypt() { char *file_name = "./test_by_aes"; char *key = "put_buffer_aes"; obs_object_info object_info; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); option.bucket_options.protocol = OBS_PROTOCOL_HTTPS; // The SSE key must be transferred during the download of SSE encrypted object. server_side_encryption_params encryption_params; memset(&encryption_params, 0, sizeof(server_side_encryption_params)); encryption_params.use_ssec = '1'; encryption_params.ssec_customer_algorithm = "AES256"; encryption_params.ssec_customer_key = "K7QkYpBkM5+hcs27fsNkUnNVaobncnLht/rCB2o/9Cw="; memset(&object_info, 0, sizeof(obs_object_info)); object_info.key =key; get_object_callback_data data; data.ret_status = OBS_STATUS_BUTT; data.outfile = write_to_file(file_name); obs_get_conditions getcondition; memset(&getcondition, 0, sizeof(obs_get_conditions)); init_get_properties(&getcondition); obs_get_object_handler get_object_handler = { { NULL, &get_object_complete_callback}, &get_object_data_callback }; get_object(&option, &object_info, &getcondition, &encryption_params, &get_object_handler, &data); if (OBS_STATUS_OK == data.ret_status) { printf("get object by_aes successfully . \n"); } else { printf("get object by_aes faied(%s).\n", obs_get_status_name(data.ret_status)); } fclose(data.outfile); }
Parent topic: Server-Side Encryption
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
The system is busy. Please try again later.
For any further questions, feel free to contact us through the chatbot.
Chatbot