Connecting to a Database
If you are connecting to an instance using Python, an SSL certificate is optional, but downloading an SSL certificate and encrypting the connection will improve the security of your instance.
SSL is disabled by default for a new GeminiDB Mongo instance. To enable SSL, see Enabling SSL.
Prerequisites
- To connect an ECS to an instance, the ECS must be able to communicate with the GeminiDB Mongo instance. You can run the following command to connect to the IP address and port of the instance server to test the network connectivity.
curl ip:port
If the message It looks like you are trying to access MongoDB over HTTP on the native driver port is displayed, the ECS and DDS instance can communicate with each other.
- Install Python and third-party installation package pymongo on the ECS. PyMongo 3.10 is recommended.
- If SSL is enabled, download the root certificate and upload it to the ECS.
Connection Code
- Enabling SSL
import ssl import os from pymongo import MongoClient # There will be security risks if the username and password used for authentication are directly written into code. Store the username and password in ciphertext in the configuration file or environment variables. # In this example, the username and password are stored in the environment variables. Before running this example, set environment variables EXAMPLE_USERNAME_ENV and EXAMPLE_PASSWORD_ENV as needed. rwuser = os.getenv('EXAMPLE_USERNAME_ENV') password = os.getenv('EXAMPLE_PASSWORD_ENV') conn_urls="mongodb://%s:%s@ip:port/{mydb}?authSource=admin" connection = MongoClient(conn_urls % (rwuser, password),connectTimeoutMS=5000,ssl=True, ssl_cert_reqs=ssl.CERT_REQUIRED,ssl_match_hostname=False,ssl_ca_certs=${path to certificate authority file}) dbs = connection.database_names() print "connect database success! database names is %s" % dbs
- Disabling SSL
import ssl import os from pymongo import MongoClient # There will be security risks if the username and password used for authentication are directly written into code. Store the username and password in ciphertext in the configuration file or environment variables. # In this example, the username and password are stored in the environment variables. Before running this example, set environment variables EXAMPLE_USERNAME_ENV and EXAMPLE_PASSWORD_ENV as needed. rwuser = os.getenv('EXAMPLE_USERNAME_ENV') password = os.getenv('EXAMPLE_PASSWORD_ENV') conn_urls="mongodb://%s:%s@ip:port/{mydb}?authSource=admin" connection = MongoClient(conn_urls % (rwuser, password),connectTimeoutMS=5000) dbs = connection.database_names() print "connect database success! database names is %s" % dbs
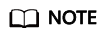
The authentication database in the URL must be admin. Set authSource to admin.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot