Connecting to a Database
Connecting to an Instance Using an SSL Certificate
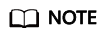
Download the SSL certificate and verify the certificate before connecting to an instance.
In the DB Information area on the Basic Information page, click in the SSL field to download the root certificate or certificate bundle.
- Use Java to connect to the MongoDB database.
- Connecting to a replica set
mongodb://<username>:<password>@<instance_ip>:<instance_port>/<database_name>?authSource=admin&replicaSet=replica&ssl=true
Table 1 Parameters Parameter
Description
<username>
Current username.
<password>
Password for the current username.
<instance_ip>
If you access an instance from an ECS, instance_ip is the private IP address of the instance.
If you access an instance through an EIP, instance_ip is the EIP that has been bound to the instance.
<instance_port>
Database port displayed on the Basic Information page. Default value: 8635
<database_name>
Name of the database to be connected.
authSource
Authentication user database. The value is admin.
ssl
Connection mode. true indicates that SSL will be used.
Example script in Java:
import java.util.ArrayList; import java.util.List; import org.bson.Document; import com.mongodb.MongoClient; import com.mongodb.MongoCredential; import com.mongodb.ServerAddress; import com.mongodb.client.MongoDatabase; import com.mongodb.client.MongoCollection; import com.mongodb.MongoClientURI; import com.mongodb.MongoClientOptions; public class MongoDBJDBC { public static void main(String[] args){ try { System.setProperty("javax.net.ssl.trustStore", "/home/Mike/jdk1.8.0_112/jre/lib/security/mongostore"); System.setProperty("javax.net.ssl.trustStorePassword", "****"); ServerAddress serverAddress = new ServerAddress("ip", port); List addrs = new ArrayList(); addrs.add(serverAddress); // There will be security risks if the username and password used for authentication are directly written into code. Store the username and password in ciphertext in the configuration file or environment variables. // In this example, the username and password are stored in the environment variables. Before running this example, set environment variables EXAMPLE_USERNAME_ENV and EXAMPLE_PASSWORD_ENV as needed. String userName = System.getenv("EXAMPLE_USERNAME_ENV"); String rwuserPassword = System.getenv("EXAMPLE_PASSWORD_ENV"); MongoCredential credential = MongoCredential.createScramSha1Credential("rwuser", "admin", rwuserPassword.toCharArray()); List credentials = new ArrayList(); credentials.add(credential); MongoClientOptions opts= MongoClientOptions.builder() .sslEnabled(true) .sslInvalidHostNameAllowed(true) .build(); MongoClient mongoClient = new MongoClient(addrs,credentials,opts); MongoDatabase mongoDatabase = mongoClient.getDatabase("testdb"); MongoCollection collection = mongoDatabase.getCollection("testCollection"); Document document = new Document("title", "MongoDB"). append("description", "database"). append("likes", 100). append("by", "Fly"); List documents = new ArrayList(); documents.add(document); collection.insertMany(documents); System.out.println("Connect to database successfully"); } catch (Exception e) { System.err.println( e.getClass().getName() + ": " + e.getMessage() ); } } }
Sample code:
javac -cp .:mongo-java-driver-3.2.0.jar MongoDBJDBC.java
java -cp .:mongo-java-driver-3.2.0.jar MongoDBJDBC
- Connecting to a replica set
Connecting to an Instance Without the SSL Certificate
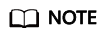
You do not need to download the SSL certificate because certificate verification on the server is not required.
- Use Java to connect to the MongoDB database.
- Connecting to a replica set
mongodb://<username>:<password>@<instance_ip>:<instance_port>/<database_name>?authSource=admin&replicaSet=replica
Table 2 Parameters Parameter
Description
<username>
Current username.
<password>
Password for the current username.
<instance_ip>
If you access an instance from an ECS, instance_ip is the private IP address of the instance.
If you access an instance through an EIP, instance_ip is the EIP that has been bound to the instance.
<instance_port>
Database port displayed on the Basic Information page. Default value: 8635
<database_name>
Name of the database to be connected.
authSource
Authentication user database. The value is admin.
Example script in Java:
import com.mongodb.ConnectionString; import com.mongodb.reactivestreams.client.MongoClients; import com.mongodb.reactivestreams.client.MongoClient; import com.mongodb.reactivestreams.client.MongoDatabase; import com.mongodb.MongoClientSettings; public class MyConnTest { final public static void main(String[] args) { try { // no ssl ConnectionString connString = new ConnectionString("mongodb://rwuser:****@192.*.*.*:8635,192.*.*.*:8635/test? authSource=admin"); MongoClientSettings settings = MongoClientSettings.builder() .applyConnectionString(connString) .retryWrites(true) .build(); MongoClient mongoClient = MongoClients.create(settings); MongoDatabase database = mongoClient.getDatabase("test"); System.out.println("Connect to database successfully"); } catch (Exception e) { e.printStackTrace(); System.out.println("Test failed"); } } }
- Connecting to a replica set
Connecting to an Instance Using YML file
- Use YML file to connect to the MongoDB database.
- Connecting to a replica set
spring: data: mongodb: uri: mongodb://<username>:<password>@<instance_ip>:<instance_port>/<database_name>?authSource=admin&replicaSet=replica
Table 3 Parameters Parameter
Description
<username>
Current username.
<password>
Password for the current username.
<instance_ip>
If you access an instance from an ECS, instance_ip is the private IP address of the instance.
If you access an instance through an EIP, instance_ip is the EIP that has been bound to the instance.
<instance_port>
Database port displayed on the Basic Information page. Default value: 8635
<database_name>
Name of the database to be connected.
authSource
Authentication user database. The value is admin.
Example script using YML file:- application.yml
spring: data: mongodb: uri: mongodb://<username>:<password>@<instance_ip>:<instance_port>/<database_name>?authSource=admin&replicaSet=replica
- ITestService.java
package com.springboot.cli.service; public interface ITestService { long doCount(); }
- TestServiceImpl.java
package com.springboot.cli.service.impl; import com.springboot.cli.service.ITestService; import lombok.RequiredArgsConstructor; import org.springframework.data.mongodb.core.MongoTemplate; import org.springframework.stereotype.Service; @Service @RequiredArgsConstructor public class TestServiceImpl implements ITestService { private final MongoTemplate mongoTemplate; @Override public long doCount() { return mongoTemplate.estimatedCount("coll"); } }
- MongodbApplicationTests.java
package com.springboot.cli; import com.springboot.cli.service.impl.TestServiceImpl; import org.junit.jupiter.api.Test; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.stereotype.Service; @SpringBootTest @Service class MongodbApplicationTests { @Autowired TestServiceImpl testService; @Test void doCount() { System.out.println("coll count is: " + testService.doCount()); } }
- application.yml
- Connecting to a replica set
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot