How Can I Connect to a PostgreSQL Database Through JDBC?
If you are connecting to a PostgreSQL database through Java database connectivity (JDBC), the SSL certificate is optional. For security reasons, you are advised to download the SSL certificate to encrypt the connection.
Prerequisites
You must be familiar with:
- Computer basics
- Java programming language
- JDBC basic knowledge
Connection with the SSL Certificate
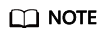
The JDBC connection is an SSL connection. The SSL certificate needs to be downloaded and verified for connecting to databases.
In the DB Information area on the Basic Information page, click in the SSL field to download the root certificate or certificate bundle.
- Connect to the RDS PostgreSQL DB instance through JDBC.
jdbc:postgresql://<instance_ip>:<instance_port>/<database_name>?sslmode=verify-full&sslrootcert=<ca.pem>
Table 1 Parameter description Parameter
Description
<instance_ip>
If you are accessing the RDS DB instance through an ECS, instance_ip indicates the floating IP address displayed on the Basic Information page of the DB instance to which you intend to connect.
If you are accessing the RDS DB instance through an EIP, instance_ip indicates the EIP that has been bound to the DB instance.
<instance_port>
Indicates the database port number displayed on the Basic Information page. The default port number is 5432.
<database_name>
Indicates the name of the database to which you intend to connect. The default database name is postgres.
sslmode
Indicates the SSL connection mode. The default mode is verify-full.
sslrootcert
Indicates the directory of the CA certificate for the SSL connection. The certificate should be stored in the directory where the command is executed.
Example script in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.Statement; public class MyConnTest { final public static void main(String[] args) { Connection conn = null; // set sslmode here. // with ssl certificate and path. String url = "jdbc:postgresql://192.168.0.225:5432/my_db_test?sslmode=verify-full&sslrootcert=/home/Ruby/ca.pem"; try { Class.forName("org.postgresql.Driver"); conn = DriverManager.getConnection(url, "root", "password"); System.out.println("Database connected"); Statement stmt = conn.createStatement(); ResultSet rs = stmt.executeQuery("SELECT * FROM mytable WHERE columnfoo = 500"); while (rs.next()) { System.out.println(rs.getString(1)); } rs.close(); stmt.close(); conn.close(); } catch (Exception e) { e.printStackTrace(); System.out.println("Test failed"); } finally { // release resource .... } } }
Connection Without the SSL Certificate
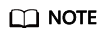
The JDBC connection is an SSL connection, but you do not need to download the SSL certificate because the certificate verification on the server is not required.
- Connect to the RDS PostgreSQL DB instance through JDBC.
jdbc:postgresql://<instance_ip>:<instance_port>/<database_name>?sslmode=disable
Table 2 Parameter description Parameter
Description
<instance_ip>
If you are accessing the RDS DB instance through an ECS, instance_ip indicates the floating IP address displayed on the Basic Information page of the DB instance to which you intend to connect.
If you are accessing the RDS DB instance through an EIP, instance_ip indicates the EIP that has been bound to the DB instance.
<instance_port>
Indicates the database port number displayed on the Basic Information page. The default port number is 5432.
<database_name>
Indicates the name of the database to which you intend to connect. The default database name is postgres.
sslmode
Indicates the SSL connection mode. disable indicates that data is not encrypted.
Example script in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.Statement; public class MyConnTest { final public static void main(String[] args) { Connection conn = null; // set sslmode here. // no ssl certificate, so do not specify path. String url = "jdbc:postgresql://192.168.0.225:5432/my_db_test?sslmode=disable"; try { Class.forName("org.postgresql.Driver"); conn = DriverManager.getConnection(url, "root", "password"); System.out.println("Database connected"); Statement stmt = conn.createStatement(); ResultSet rs = stmt.executeQuery("SELECT * FROM mytable WHERE columnfoo = 500"); while (rs.next()) { System.out.println(rs.getString(1)); } rs.close(); stmt.close(); conn.close(); } catch (Exception e) { e.printStackTrace(); System.out.println("Test failed"); } finally { // release resource .... } } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot