Reading Data from a File
Function
Read data from a specified file in the Hadoop distributed file system (HDFS). The process is:
- Use the open method in the FileSystem instance to obtain the input stream of writing files.
- Use the input stream to read the content of the specified file in the HDFS.
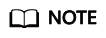
Close all requested resources after reading files.
Example Codes
The following is code snippets. For complete codes, see the HdfsExample class in com.huawei.bigdata.hdfs.examples.
/** * Read s file. * * @throws java.io.IOException */ private void read() throws IOException { String strPath = DEST_PATH + File.separator + FILE_NAME; Path path = new Path(strPath); FSDataInputStream in = null; BufferedReader reader = null; StringBuffer strBuffer = new StringBuffer(); try { in = fSystem.open(path); reader = new BufferedReader(new InputStreamReader(in)); String sTempOneLine; // write file while ((sTempOneLine = reader.readLine()) != null) { strBuffer.append(sTempOneLine); } LOG.info("result is : " + strBuffer.toString()); LOG.info("success to read."); } finally { // make sure the streams are closed finally. IOUtils.closeStream(reader); IOUtils.closeStream(in); } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot