Operate Namespaces Using REST
Function
Use the REST service to import the URL consisting of the host and port and the specified namespace, Use HTTPS to create, query, and delete namespaces, and obtain tables in the specified namespace.
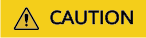
HBase tables are stored in Namespace:Table name format. If no namespace is specified when a table is created, the table is stored in default. The hbase namespace is the system table namespace. Do not create service tables or read or write data in the system table namespace.
Example Code
- Invoking methods
// Namespace operations. createNamespace(url, "testNs"); getAllNamespace(url); deleteNamespace(url, "testNs"); getAllNamespaceTables(url, "default");
- Creating a namespace
The following code snippets are in the createNamespace method in the HBaseRestTest class of the hbase-rest-example\src\main\java\com\huawei\hadoop\hbase\examples packet.
private void createNamespace(String url, String namespace) { String endpoint = "/namespaces/" + namespace; Optional<ResultModel> result = sendAction(url + endpoint, MethodType.POST, null); if (result.orElse(new ResultModel()).getStatusCode() == HttpStatus.SC_CREATED) { LOG.info("Create namespace '{}' success.", namespace); } else { LOG.error("Create namespace '{}' failed.", namespace); } }
- Querying all namespaces
The following code snippets are in the getAllNamespace method in the HBaseRestTest class of the hbase-rest-example\src\main\java\com\huawei\hadoop\hbase\examples packet.
private void getAllNamespace(String url) { String endpoint = "/namespaces"; Optional<ResultModel> result = sendAction(url + endpoint, MethodType.GET, null); handleNormalResult(result); }
- Deleting a specified namespace
The following code snippets are in the deleteNamespace method in the HBaseRestTest class of the hbase-rest-example\src\main\java\com\huawei\hadoop\hbase\examples packet.
private void deleteNamespace(String url, String namespace) { String endpoint = "/namespaces/" + namespace; Optional<ResultModel> result = sendAction(url + endpoint, MethodType.DELETE, null); if (result.orElse(new ResultModel()).getStatusCode() == HttpStatus.SC_OK) { LOG.info("Delete namespace '{}' success.", namespace); } else { LOG.error("Delete namespace '{}' failed.", namespace); } }
- Obtain tables in a specified namespace.
The following code snippets are in the getAllNamespaceTables method in the HBaseRestTest class of the hbase-rest-example\src\main\java\com\huawei\hadoop\hbase\examples packet.
private void getAllNamespaceTables(String url, String namespace) { String endpoint = "/namespaces/" + namespace + "/tables"; Optional<ResultModel> result = sendAction(url + endpoint, MethodType.GET, null); handleNormalResult(result); }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot