Example: JavaScript Page Integration Code
Environment Requirement |
- |
---|---|
Reference Library |
jquery.js |
Download Link |
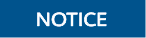
-
The demo described in this document may involve the use of personal data. You are advised to comply with relevant laws and regulations and take sufficient measures to ensure that personal data is fully protected.
-
The demo described in this document is for demonstration only. Commercial use of the demo is prohibited.
-
Information in this document is for reference only and does not constitute any offer or commitment.
index.html
<!DOCTYPE html> <html> <head> <!-- If the co-browsing function is required, introduce the cobrowse.js and cobrowseCommon.js components. Replace ip:port with the IP address and port number of the CEC, or directly with the domain name of the CEC. --> <script type="text/javascript" src="https://ip:port/service-cloud/resource.root/cobrowse/sdk/cobrowse.js"></script> <script type="text/javascript" src="https://ip:port//service-cloud/webclient/chat_client/js/cobrowseCommon.js"></script> <!-- 0. Introduce the jQuery component. --> <script type="text/javascript" src="jquery.js"></script> </head> <body> <script> // 1. Define variables, which will be used on the chat box page. For details about the parameters, see Table 1. const userId = "XXXXXXXX"; // user ID (value of thirdUserId in the table) const userName = "XXXXXXXXXXX"; // user nickname (value of thirdUserName in the table) const tenantSpaceId ="XXXXXXXXXX"; // tenant space ID (tenant id) const configId = "XXXXXXXXXXXXXXXXXX"; // channel ID (wWEBchannelConfig id). const locale = "zh"; // language (locale, which can be zh or en) const $ContextPath = "https://ip:port/service-cloud"; // request URL // 2. Construct request parameters. let serviceUrl = $ContextPath + "/webclient/chat_client/js/newThirdPartyClient.js?&t="+new Date().getTime(); let thirdUserData = {}; thirdUserData['thirdUserName'] = userName; thirdUserData['thirdUserId'] = userId; thirdUserData['tenantSpaceId'] = tenantSpaceId; thirdUserData['channelConfigId'] = configId; thirdUserData['locale'] = locale; thirdUserData['timestamp'] = new Date().getTime(); // timestamp thirdUserData['secretKey'] = 'XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX'; // authentication key getAuthorization(thirdUserData); // authorization signature // 3. Request newThirdPartyClient.js. var request = $.ajax({ url: serviceUrl, method: "POST", data: JSON.stringify(thirdUserData), xhrFields: { withCredentials: true }, success: function (data) { // 4. Create a script tag and run the newThirdPartyClient.js script. importScript(data); }, error: function (XMLHttpRequest, textStatus) { alert("unauthorized,validate failed") } }); // 5. Load and run the JavaScript on the integration page. var importScript = (function (oHead) { function loadError(oError) { throw new URIError("The script " + oError.target.src + " is not accessible."); } return function (sSrc, fOnload) { var oScript = document.createElement("script"); oScript.type = "text/javascript"; oScript.onerror = loadError; if (fOnload) { oScript.onload = fOnload; } oHead.appendChild(oScript); oScript.innerHTML = sSrc; } })(document.head || document.getElementsByTagName("body")[0]); // Obtain the authorization signature based on parameters. function getAuthorization(thirdUserData){ $.ajax({ url:"/webchat/authorizationService", method:"post", data:JSON.stringify(thirdUserData), async:false, success: function (data) { thirdUserData['thirdPartAuthorization'] = data; } }); } </script> </body> </html>
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot