KafkaProducer
Path
com.roma.apic.livedata.client.v1.KafkaProducer
Description
This class is used to produce Kafka messages.
Example
importClass(com.roma.apic.livedata.client.v1.KafkaProducer); importClass(com.roma.apic.livedata.config.v1.KafkaConfig); var kafka_brokers = '1.1.1.1:26330,2.2.2.2:26330' var topic = 'YourKafkaTopic' function execute(data) { var config = KafkaConfig.getConfig(kafka_brokers, null) var producer = new KafkaProducer(config) var record = producer.produce(topic, "hello, kafka.") return { offset: record.offset(), partition: record.partition(), code: 0, message: "OK" } }
Constructor Details
public KafkaProducer(Map configs)
Constructs a Kafka message producer.
Parameter: configs indicates configuration information of the Kafka.
Method List
Returned Type |
Method and Description |
---|---|
org.apache.kafka.clients.producer.RecordMetadata |
produce(String topic, String message) Produce messages. |
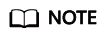
The produce(String topic, String message) method cannot be directly returned. Otherwise, the returned information is empty. For example, do not use the return record statement directly in the preceding example. Otherwise, the returned information is empty.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot