HttpClient
Path
- com.roma.apic.livedata.client.v1.HttpClient
- com.huawei.livedata.lambdaservice.livedataprovider.HttpClient
Description
This class is used to send HTTP requests.
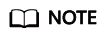
Some common HTTP response headers, such as Location, cannot be returned.
Example
- com.roma.apic.livedata.client.v1.HttpClient
importClass(com.roma.apic.livedata.client.v1.HttpClient); importClass(com.roma.apic.livedata.provider.v1.APIConnectResponse); function execute(data) { var httpClient = new HttpClient(); var resp = httpClient.request('GET', 'http://apigdemo.exampleRegion.com/api/echo', {}, null, 'application/json'); myHeaders = resp.headers(); proxyHeaders = {}; for (var key in myHeaders) { proxyHeaders[key] = myHeaders.get(key); } return new APIConnectResponse(resp.code(), proxyHeaders, resp.body().string(), false); }
- com.huawei.livedata.lambdaservice.livedataprovider.HttpClient
importClass(com.huawei.livedata.lambdaservice.livedataprovider.HttpClient); function excute(data) { var httpExecutor = new HttpClient(); var obj = JSON.parse(data); var host = 'xx.xx.xxx.xx:xxxx'; var headers = { 'clientapp' : 'FunctionStage' }; var params = { 'employ_no' :'00xxxxxx' }; var result = httpExecutor.callGETAPI(host,'/livews/rest/apiservice/iData/personInfo/batch',JSON.stringify(params),JSON.stringify(headers)); return result; }
Constructor Details
- com.roma.apic.livedata.client.v1.HttpClient
Constructs an HttpClient without parameters.
public HttpClient(HttpConfig config)
Constructs an HttpClient that contains the HttpConfig configuration information.
Parameter: config indicates the HttpClient configuration information.
- com.huawei.livedata.lambdaservice.livedataprovider.HttpClient
Constructs an HttpClient without parameters.
Method List
- com.roma.apic.livedata.client.v1.HttpClient
Returned Type
Method and Description
okhttp3.Response
request(HttpConfig config)
Send REST requests.
okhttp3.Response
request(String method, String url)
Send a REST request by specifying the request method and path.
okhttp3.Response
request(String method, String url, Map<String,String> headers)
Send a REST request by specifying the request method, path, and header.
okhttp3.Response
request(String method, String url, Map<String,String> headers, String body)
Send a REST request by specifying the request method, path, header, and body.
okhttp3.Response
request(String method, String url, Map<String,String> headers, String body, String contentType)
Send a REST request by specifying the request method, path, header, body, and content type.
- com.huawei.livedata.lambdaservice.livedataprovider.HttpClient
Returned Type
Method and Description
String
callGETAPI(String url)
Use the get method to invoke the HTTP or HTTPS service.
String
callGETAPI(String host, String service, String params, String header)
Use the get method to invoke the HTTP or HTTPS service.
Response
get(String url, String header)
Use the get method to invoke the HTTP or HTTPS service.
String
callPostAPI(String host, String service, String content, String header, String contentType)
Use the post method to invoke the HTTP or HTTPS service.
String
callPostAPI(String url, String header, String requestBody, String type)
Use the post method to invoke the HTTP or HTTPS service.
Response
post(String url, String header, String content, String type)
Use the post method to invoke the HTTP or HTTPS service.
String
callFormPost(String url, String header, String/Map param)
Invoke the HTTP or HTTPS service in the formdata format.
Response
callFormPost(String url, String header, String param, FormDataMultiPart form)
Invoke the HTTP or HTTPS service in the formdata format.
String
callDelAPI(String url, String header, String content, String type)
Use the delete method to invoke the HTTP or HTTPS service.
String
callPUTAPI(String url, String header, String content, String type)
Use the put method to invoke the HTTP or HTTPS service.
String
callPatchAPI(String url, String header, String content, String type)
Use the patch method to invoke the HTTP or HTTPS service.
Response
put(String url, String header, String content, String type)
Use the put method to invoke the HTTP or HTTPS service.
Method Details
- com.roma.apic.livedata.client.v1.HttpClient
- public okhttp3.Response request(HttpConfig config)
Send REST requests.
Input Parameter
config indicates the HttpConfig configuration information.
Returns
Response body.
- public okhttp3.Response request(String method, String url)
Send a REST request by specifying the request method and path.
Input Parameter
- method indicates a request method.
- url indicates a request URL.
Returns
Response body.
- public okhttp3.Response request(String method, String url, Map<String,String> headers)
Send a REST request by specifying the request method, path, and header.
Input Parameter
- method indicates a request method.
- url indicates a request URL.
- headers indicates the request header information of the map type.
Returns
Response body.
- public okhttp3.Response request(String method, String url, Map<String,String> headers, String body)
Send a REST request by specifying the request method, path, header, and body.
Input Parameter
- method indicates a request method.
- url indicates a request URL.
- headers indicates the request header information of the map type.
- body indicates the request body.
Returns
Response body.
- public okhttp3.Response request(String method, String url, Map<String,String> headers, String body, String contentType)
Send a REST request by specifying the request method, path, header, body, and content type.
Input Parameter
- method indicates a request method.
- url indicates a request URL.
- headers indicates the request header information of the map type.
- body indicates the request body.
- contentType indicates the content type of the request body.
Returns
Response body.
- public okhttp3.Response request(HttpConfig config)
- com.huawei.livedata.lambdaservice.livedataprovider.HttpClient
- public String callGETAPI(String url)
Use the get method to invoke the HTTP or HTTPS service.
Input Parameter
url indicates the service address.
Returns
Response body.
- public String callGETAPI(String host, String service, String params, String header)
Use the get method to invoke the HTTP or HTTPS service.
Input Parameter
- host indicates the service address.
- service indicates the service path.
- params indicates the HTTP parameter information.
- header indicates the HTTP header information.
Returns
Response body.
- public Response get(String url, String header)
Use the get method to invoke the HTTP or HTTPS service.
Input Parameter
- url indicates the service address.
- header indicates the request header information.
Returns
Response body.
- public String callPostAPI(String host, String service, String content, String header, String contentType)
Use the post method to invoke the HTTP or HTTPS service.
Input Parameter
- host indicates the service address.
- service indicates the service path.
- content indicates the message body.
- header indicates the request header information.
- contentType indicates the content type.
Returns
Response body.
- public String callPostAPI(String url, String header, String requestBody, String type)
Use the post method to invoke the HTTP or HTTPS service.
Input Parameter
- url indicates the service address.
- header indicates the request header information.
- requestBody indicates the message body.
- type indicates the MIME type.
Returns
Response body.
- public Response post(String url, String header, String content, String type)
Use the post method to invoke the HTTP or HTTPS service.
Input Parameter
- url indicates the service address.
- header indicates the request header information.
- content indicates the message body.
- type indicates the MIME type.
Returns
Response body.
- public String callFormPost(String url, String header, String/Map param)
Invoke the HTTP or HTTPS service in the formdata format.
Input Parameter
- url indicates the service address.
- header indicates the request header information.
- param indicates the parameter information.
Returns
Response body.
- public Response callFormPost(String url, String header, String param, FormDataMultiPart form)
Invoke the HTTP or HTTPS service in the formdata format.
Input Parameter
- url indicates the service address.
- header indicates the request header information.
- param indicates the parameter information.
- form indicates the body parameter.
Returns
Response body.
- public String callDelAPI(String url, String header, String content, String type)
Use the delete method to invoke the HTTP or HTTPS service.
Input Parameter
- url indicates the service address.
- header indicates the request header information.
- content indicates the message body.
- type indicates the MIME type.
Returns
Response body.
- public String callPUTAPI(String url, String header, String content, String type)
Use the put method to invoke the HTTP or HTTPS service.
Input Parameter
- url indicates the service address.
- header indicates the request header information.
- content indicates the message body.
- type indicates the MIME type.
Returns
Response body.
- public String callPatchAPI(String url, String header, String content, String type)
Use the patch method to invoke the HTTP or HTTPS service.
Input Parameter
- url indicates the service address.
- header indicates the request header information.
- content indicates the message body.
- type indicates the MIME type.
Returns
Response body.
- public Response put(String url, String header, String content, String type)
Use the put method to invoke the HTTP or HTTPS service.
Input Parameter
- url indicates the service address.
- header indicates the request header information.
- content indicates the message body.
- type indicates the MIME type.
Returns
Response body.
- public String callGETAPI(String url)
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot