Java
Scenarios
To use Java to sign backend requests, obtain the Java SDK, import the project, and verify the backend signature by referring to the example provided in this section.
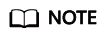
The Java SDK supports only basic and HMAC backend service signatures.
Prerequisites
- A signature key has been created on the console and bound to an API. For details, see Configuring Signature Verification for Backend Services.
- You have obtained the signature key and secret. For details, see Preparations.
- You have installed the development tool and Java development environment. For details, see Preparations.
Obtaining the SDK
Old version: Log in to the ROMA Connect console, choose API Connect > API Management > Signature Keys, and download the SDK.
New version: Log in to the ROMA Connect console, choose API Connect > Credentials > SDKs, and download the SDK.
Importing a Project
- Open IntelliJ IDEA, choose File > New > Project from Existing Sources, select the apigateway-backend-signature-demo\pom.xml file, and click OK.
Figure 1 Select File or Directory to Import
- Retain the default settings, click Next for the following four steps, and then click Finish.
- On the Maven tab page on the right, double-click compile to compile the file.
Figure 2 Compiling the project
If the message "BUILD SUCCESS" is displayed, the compilation is successful.
- Right-click BackendSignatureApplication and choose Run.
Figure 3 Running the BackendSignatureApplication service
Modify the parameters in sample code ApigatewaySignatureFilter.java as required. For details about the sample code, see Example of Verifying the Backend Signature of hmac Type.
Example of Verifying the Backend Signature of hmac Type
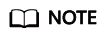
- This example demonstrates how to write a Spring boot–based server as the backend of an API and implement a filter to verify the signature of requests sent from APIC.
- Signature information is added to requests sent to access the backend of an API after a signature key of hmac type is bound to the API.
- Compile a controller in the /hmac directory.
// HelloController.java @RestController @EnableAutoConfiguration public class HelloController { @RequestMapping("/hmac") private String hmac() { return "Hmac authorization success"; } }
- Compile a filter that matches all request paths and methods, and put the signature key and secret in a Map.
public class ApigatewaySignatureFilter implements Filter { private static Map<String, String> secrets = new HashMap<>(); static { // Directly writing AK/SK in code is risky. For security, encrypt your AK/SK and store them in the configuration file or environment variables. // In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, set environment variables HUAWEICLOUD_SDK_AK1, HUAWEICLOUD_SDK_SK1, and HUAWEICLOUD_SDK_AK2, HUAWEICLOUD_SDK_SK2. secrets.put(System.getenv("HUAWEICLOUD_SDK_AK1"), System.getenv("HUAWEICLOUD_SDK_SK1")); secrets.put(System.getenv("HUAWEICLOUD_SDK_AK2"), System.getenv("HUAWEICLOUD_SDK_SK2")); } @Override public void init(FilterConfig filterConfig) throws ServletException { } @Override public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain chain) { //Signature verification code ... } }
- The doFilter function is the signature verification code. To ensure that the body can be read in the filter and controller, wrap the request and send it to the filter and controller. For the implementation of wrapper classes, see RequestWrapper.java.
RequestWrapper request = new RequestWrapper((HttpServletRequest) servletRequest);
- Use a regular expression to parse the Authorization header to obtain signingKey and signedHeaders.
private static final Pattern authorizationPattern = Pattern.compile("SDK-HMAC-SHA256\\s+Access=([^,]+),\\s?SignedHeaders=([^,]+),\\s?Signature=(\\w+)"); ... String authorization = request.getHeader("Authorization"); if (authorization == null || authorization.length() == 0) { response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Authorization not found."); return; } Matcher m = authorizationPattern.matcher(authorization); if (!m.find()) { response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Authorization format incorrect."); return; } String signingKey = m.group(1); String signingSecret = secrets.get(signingKey); if (signingSecret == null) { response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Signing key not found."); return; } String[] signedHeaders = m.group(2).split(";");
For example, for Authorization header:
SDK-HMAC-SHA256 Access=signature_key1, SignedHeaders=host;x-sdk-date, Signature=e11adf65a20d1b82c25419b5********8d0ba12fed1ceb13ed00
The parsing result is as follows:
signingKey=signature_key1 signedHeaders=host;x-sdk-date
- Find signingSecret based on signingKey. If signingKey does not exist, the authentication failed.
String signingSecret = secrets.get(signingKey); if (signingSecret == null) { response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Signing key not found."); return; }
- Create a request, and add the method, URL, query, and signedHeaders headers to the request. Determine whether the body needs to be set.
The body is read if there is no x-sdk-content-sha256 header with value UNSIGNED-PAYLOAD.
Request apiRequest = new DefaultRequest(); apiRequest.setHttpMethod(HttpMethodName.valueOf(request.getMethod())); String url = request.getRequestURL().toString(); String queryString = request.getQueryString(); try { apiRequest.setEndpoint((new URL(url)).toURI()); Map<String, String> parametersmap = new HashMap<>(); if (null != queryString && !"".equals(queryString)) { String[] parameterarray = queryString.split("&"); for (String p : parameterarray) { String[] p_split = p.split("=", 2); String key = p_split[0]; String value = ""; if (p_split.length >= 2) { value = p_split[1]; } parametersmap.put(URLDecoder.decode(key, "UTF-8"), URLDecoder.decode(value, "UTF-8")); } apiRequest.setParameters(parametersmap); //set query } } catch (URISyntaxException e) { e.printStackTrace(); } boolean needbody = true; String dateHeader = null; for (int i = 0; i < signedHeaders.length; i++) { String headerValue = request.getHeader(signedHeaders[i]); if (headerValue == null || headerValue.length() == 0) { ((HttpServletResponse) response).sendError(HttpServletResponse.SC_UNAUTHORIZED, "signed header" + signedHeaders[i] + " not found."); } else { apiRequest.addHeader(signedHeaders[i], headerValue);//set header if (signedHeaders[i].toLowerCase().equals("x-sdk-content-sha256") && headerValue.equals("UNSIGNED-PAYLOAD")) { needbody = false; } if (signedHeaders[i].toLowerCase().equals("x-sdk-date")) { dateHeader = headerValue; } } } if (needbody) { apiRequest.setContent(new ByteArrayInputStream(request.getBody())); //set body }
- Check whether the signature has expired. Obtain the time from the X-Sdk-Date header, and check whether the difference between this time and the server time is within 15 minutes. If signedHeaders does not contain X-Sdk-Date, the authentication failed.
private static final DateTimeFormatter timeFormatter = DateTimeFormat.forPattern("yyyyMMdd'T'HHmmss'Z'").withZoneUTC(); ... if (dateHeader == null) { response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Header x-sdk-date not found."); return; } long date = timeFormatter.parseMillis(dateHeader); long duration = Math.abs(DateTime.now().getMillis() - date); if (duration > 15 * 60 * 1000) { response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Signature expired."); return; }
- Add the Authorization header to the request, and invoke the verify method to verify the request signature. If the verification is successful, the next filter is executed. Otherwise, the authentication failed.
DefaultSigner signer = (DefaultSigner) SignerFactory.getSigner(); boolean verify = signer.verify(apiRequest, new BasicCredentials(signingKey, signingSecret)); if (verify) { chain.doFilter(request, response); } else { response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "verify authroization failed."); }
- Register the mapping between filters and paths.
@Configuration public class FilterConfig { @Bean public FilterRegistrationBean registApigatewaySignatureFilter() { FilterRegistrationBean registration = new FilterRegistrationBean(); registration.setFilter(new ApigatewaySignatureFilter()); registration.addUrlPatterns("/hmac"); registration.setName("ApigatewaySignatureFilter"); return registration; } }
- Run the server to verify the code. The following example uses the HTML signature tool in the JavaScript SDK to generate a signature.
Set the parameters according to the following figure, and click Send request. Copy the generated curl command, execute it in the CLI, and check whether the server returns Hello World!
If an incorrect key or secret is used, the server returns 401, which means authentication failure.
Example of Verifying the Backend Signature of basic Type
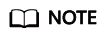
- This example demonstrates how to write a Spring boot–based server as the backend of an API and implement a filter to verify the signature of requests sent from APIC.
- Basic authentication information is added to requests sent to the backend of an API after a basic signature key is bound to an API. The username for basic authentication is the key of the signature key, and the password is the secret of the signature key.
- Compile a controller in the /basic directory.
// HelloController.java @RestController @EnableAutoConfiguration public class HelloController { @RequestMapping("/basic") private String basic() { return "Basic authorization success"; } }
- Compile a filter. According to the basic authentication rule, the Authorization header is in the format of "Basic "+base64encode(username+":"+password). The following is the verification code compiled according to the rule:
// BasicAuthFilter.java public class BasicAuthFilter implements Filter { private static final String CREDENTIALS_PREFIX = "Basic "; private static Map<String, String> secrets = new HashMap<>(); static { secrets.put("signature_key1", "signature_secret1"); secrets.put("signature_key2", "signature_secret2"); } @Override public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain chain) { HttpServletRequest request = (HttpServletRequest) servletRequest; HttpServletResponse response = (HttpServletResponse) servletResponse; try { String credentials = request.getHeader("Authorization"); if (credentials == null || credentials.length() == 0) { response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Authorization not found."); return; } if (!credentials.startsWith(CREDENTIALS_PREFIX)) { response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Authorization format incorrect."); return; } String authInfo = credentials.substring(CREDENTIALS_PREFIX.length()); String decoded; try { decoded = new String(Base64.getDecoder().decode(authInfo)); } catch (IllegalArgumentException e) { response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Authorization format incorrect."); return; } String[] spl = decoded.split(":", 2); if (spl.length < 2) { response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Authorization format incorrect."); return; } String signingSecret = secrets.get(spl[0]); if (signingSecret == null) { response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Username not found."); return; } if (signingSecret.equals(spl[1])) { chain.doFilter(request, response); } else { response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Incorrect username or password"); } } catch (Exception e) { e.printStackTrace(); try { response.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR); } catch (IOException e1) { } } } }
- Register the mapping between filters and paths.
@Configuration public class FilterConfig { @Bean public FilterRegistrationBean registBasicAuthFilter() { FilterRegistrationBean registration = new FilterRegistrationBean(); registration.setFilter(new BasicAuthFilter()); registration.addUrlPatterns("/basic"); registration.setName("BasicAuthFilter"); return registration; } }
- Run the server to verify the code. Generate the Authorization header field of the basic authentication based on the username and password and send the header field to the request interface. If an incorrect username or password is used, the server returns 401, which means authentication failure.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot