Using gotron-sdk to Send gRPC Requests
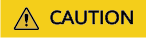
gotron-sdk of the version 2.3.0 and earlier is supported. The gRPC client created in default mode does not support the connection to the gRPC solidity node.
Download the certificate on the node details page and place the ca.crt certificate in the package under the project directory.
Configure the gRPC endpoint in the SDK. The sample code is as follows:
import ( "fmt" "google.golang.org/gRPC" "google.golang.org/gRPC/credentials" "github.com/fbsobreira/gotron-sdk/pkg/client" ) func main() { gRPCWalletEndpoint := "your-gRPC-endpoint" credential := "your-credential" caPath := "your-ca.crt-file-path" creds, err := credentials.NewClientTLSFromFile(caPath, "") if err != nil { fmt.Printf("failed to load credentials: %v\n", err) } gRPCWalletClient := client.NewgRPCClient(gRPCWalletEndpoint) gRPCWalletClient.SetAPIKey(credential) gRPCWalletClient.Start(gRPC.WithTransportCredentials(creds)) resp, err := gRPCWalletClient.GetNowBlock() if err != nil { fmt.Printf("failed to get now block: %v\n", err) } fmt.Println("wallet resp: ", resp) }
Response example
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot