Java Example Code
Description
Run the IoTDB SQL statement in Session connection mode.
Sample Code
The following code snippets are used as an example. For complete code, see com.huawei.bigdata.SessionExample.
Session object parameters include the IP address, port number, username, and password of the node where the IoTDBServer is located.
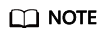
- On FusionInsight Manager, choose Cluster > Services > IoTDB > Instance to view the IP address of the node where the IoTDBServer to be connected is located.
- To obtain the RPC port number, log in to FusionInsight Manager, choose Cluster > Services > IoTDB. Click Configuration, click All Configurations, and search for IOTDB_SERVER_RPC_PORT.
- In normal mode, IoTDB has a default user root after initial installation, and the password is root. This user is an administrator and has all permissions, which cannot be assigned, revoked, or deleted.
private static final String IOTDB_SSL_ENABLE = "true";//To obtain the value, log in to FusionInsight Manager, choose Cluster > Services > IoTDB, click Configurations, search for SSL in the search box, and view the value of SSL_ENABLE. public static void main(String[] args) throws IoTDBConnectionException, StatementExecutionException { // set iotdb_ssl_enable System.setProperty("iotdb_ssl_enable", IOTDB_SSL_ENABLE); if ("true".equals(IOTDB_SSL_ENABLE)) { // set truststore.jks path System.setProperty("iotdb_ssl_truststore", "truststore file path"); } session = new Session(nodeUrls, "root", "Password"); session.open(false); // set session fetchSize session.setFetchSize(10000); try { session.setStorageGroup("root.sg1"); } catch (StatementExecutionException e) { if (e.getStatusCode() != TSStatusCode.PATH_ALREADY_EXIST_ERROR.getStatusCode()) { throw e; } } createTimeseries(); createMultiTimeseries(); insertRecord(); insertTablet(); insertTablets(); insertRecords(); nonQuery(); query(); queryWithTimeout(); rawDataQuery(); queryByIterator(); deleteData(); deleteTimeseries(); setTimeout(); sessionEnableRedirect = new Session(nodeUrls, "root", "Password"); sessionEnableRedirect.setEnableQueryRedirection(true); sessionEnableRedirect.open(false); // set session fetchSize sessionEnableRedirect.setFetchSize(10000); insertRecord4Redirect(); query4Redirect(); sessionEnableRedirect.close(); session.close(); }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot