SDK Usage Instructions
The data subscription function caches data changes of key services in the database and provides a unified SDK API for downstream services to subscribe to, obtain, and consume.
Before using the SDK, create a data subscription task on the DRS console. For details, see Creating a Data Subscription Task.
After a subscription task is created, you can use an SDK to subscribe to incremental data in the subscription task in real time. The following lists the constraints:
- DRS only provides SDK JAVA version. For details, see SDK Download Address.
- One subscription channel can be consumed by only one SDK. If multiple SDKs are connected to one data subscription channel, only one SDK process can obtain the data change information. If multiple downstream SDKs must subscribe to the same RDS instance, you need to create a data subscription task for each SDK.
- A downstream SDK cannot subscribe to and consume multiple subscription tasks.
- After data subscription is successful, new data can be retained for a maximum of three days if the data is not consumed in time.
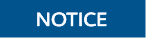
The SDK JAVA version provided by DRS supports the development environment of JDK 1.6 or later. JDK 1.8 is recommended.
Network Type
Currently, only VPCs are supported.
SDK Working Principles
Data consumption and message confirmation are two asynchronous threads started based on the transaction sequence. The two threads are independent of each other and comply with the causal sequence. The received messages invoke the notify function registered by users in sequence. The SDK ensures that each message is pushed only once.
Importing SDK JAR Package
The SDK JAR package can be directly imported only through the library. Currently, the Maven central repository is not supported.
<dependency> <groupId>com.huawei.hwclouds</groupId> <artifactId>drs-subscribe-sdk</artifactId> <version>1.0</version> </dependency>
Configuring Parameters
During data subscription, some parameters affect the speed and interval for obtaining data.
Before starting the SDK, you can set the following parameters in the subscribe.properties configuration file as required:
#Initial delay for obtaining the subscription data MESSAGE_DELAY_TIME = 1500000 #Interval for obtaining the subscription data MESSAGE_PERIOD_TIME = 2000000 #Notify the user that the subscription data initial delay NOTIFY_DELAY_TIME = 2000 #Notify the user of the subscription data interval NOTIFY_PERIOD_TIME = 1000 #Initial delay of the ACK messages from the server ACK_DELAY_TIME = 3000 #Interval between the ACK messages from the server ACK_PERIOD_TIME = 5000
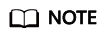
- The preceding parameter values are set by default. You can also customize parameter values based on the site requirements.
- The unit of the time mentioned above is microsecond.
Confirmation Mechanism
The SDK uses the automatic batch confirmation mechanism. The client program does not need to invoke the confirmation function and can be confirmed repeatedly.
For example: the client receives five batches of messages, and the server only confirms the first, the second, and the fifth batch, respectively. Messages are confirmed in sequence. Therefore, it indicates that the client has consumed all messages in the first to fifth batch. If the client program is suspended, the consumption checkpoint starts from the fifth batch.
SDK Template
import com.huawei.hwclouds.drs.context.SubscribeContext; import com.huawei.hwclouds.drs.message.ClusterMessage; import com.huawei.hwclouds.drs.subscribe.ClusterListener; import com.huawei.hwclouds.drs.subscribe.DefaultSubscribeClient; import com.huawei.hwclouds.drs.subscribe.SubscribeClient; import java.util.List; public class MainClass { public static void main(String[] args) throws Exception { SubscribeContext context = new SubscribeContext(); //There will be security risks if the username and password used for authentication are directly written into code. Store the username and password in ciphertext in the configuration file or environment variables. //In this example, the username and password are stored in the environment variables. Before running this example, set environment variables EXAMPLE_USERNAME_ENV and EXAMPLE_PASSWORD_ENV based on site requirements. //Set a Username of the current cloud account. String username = System.getenv("EXAMPLE_USERNAME_ENV"); context.setDomainName(username); //Set the Password of the current cloud account. String userpassword = System.getenv("EXAMPLE_PASSWORD_ENV"); context.setPassword(userpassword); //Set the IP address of the subscription instance on the Data Subscription Management page. context.setIp("SubscribeChannelIp"); context.setUserId("userId"); SubscribeClient client = DefaultSubscribeClient.getSubscribeClient(context); ClusterListener clusterListener = new ClusterListener() { @Override //Client consumption behavior defined in notify public void notify(List<ClusterMessage> var1) throws Exception { for (ClusterMessage message : var1) { System.out.println("Message is " + message.toString()); } } }; client.addClusterListener(clusterListener); client.start(); } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot