Client Object (Client)
This section describes the client APIs of the Web SDK.
API |
Description |
---|---|
Joins a specific room for an audio or video call. |
|
Leaves a room after a call ends. |
|
Publishes a local stream after a user joins a room. |
|
Cancels the publication of a local stream. |
|
Subscribes to a remote audio and video media stream. |
|
Unsubscribes from a remote audio and video media stream. |
|
Subscribes to video media streams of remote users in batches. |
|
Switches the role of a user. |
|
Registers the callback for a client object event. |
|
Deregisters the callback for a client object event. |
|
Obtains the client connection state. |
|
Obtains the network transmission statistics. |
|
Obtains local audio statistics. |
|
Obtains local video statistics. |
|
Obtains remote audio statistics. |
|
Obtains remote video statistics. |
|
Sets whether to enable the top-N-audio mode. |
|
Sets the volume in the top-N-audio mode. This API is added in version 1.4.0. |
|
Enables or disables all audio tracks in the top-N-audio mode. This API is added in version 1.4.0. |
|
Enables or disables video stream state detection. This API is added in version 1.4.0. |
|
Changes the nickname of a user. This API is added in version 1.5.0. |
|
Configures the proxy reverse server deployed in the enterprise. This API is added in version 2.0.3. |
|
Configures a proxy server. This API is added in version 2.0.3. |
|
Configures whether to enable the RTC audio and video stream statistics event. This API is added in version 2.0.3. |
|
Configures the maximum media bandwidth. This API is added in version 2.0.5. |
|
Updates a signature. This API is added in version 2.0.8. |
join
async join(roomId: string, options: JoinConfig): Promise<void>
[Function Description]
Joins a room for an audio or video call.
[Request Parameters]
- roomId: unique ID of a room. (Mandatory) string[64] type.
- options: configuration of joining a room. (Mandatory) JoinConfig type.
JoinConfig is defined as: {
- userId: user ID, which must be unique in an application. (Mandatory) string[64] type.
- userName: username. (Optional) string[256] type.
- signature: signature. For details about how to generate a signature, see Access Authentication. (Mandatory) string[512] type.
- ctime: (mandatory) authentication signature UTC timestamp, in seconds. The type is string.
- role: (mandatory) user role, which can be used to identify the media direction. The type is number. The enumerated values of role are as follows:
- 0: joiner, who can send and receive audio and video.
- 2: player, who only receives others' audio and video but does not send their own audio and video.
[Response Parameters]
Promise<void>: returns a Promise object.
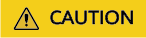
- The value of roomId can contain letters, digits, hyphens (-), and underscores (_).
- The value of userId can contain letters, digits, hyphens (-), and underscores (_).
leave
async leave(): Promise<void>
[Function Description]
Leaves a room after a call ends.
[Request Parameters]
None
[Response Parameters]
Promise<void>: returns a Promise object.
publish
async publish(stream: Stream, option?: PublishOption): Promise<void>
[Function Description]
Publishes the local stream after a user joins a room and create a local stream. Only joiner can publish local streams.
[Request Parameters]
- stream: (mandatory) local stream object. The type is Stream.
- option: (optional) whether to proactively publish video streams. The type is PublishOption. If this parameter is not passed, video streams are not proactively published.
PublishOption is defined as:
{
autoPushVideo: (optional) whether to proactively publish video streams. The type is Boolean. The default value is false.
}
[Response Parameters]
Promise<void>: returns a Promise object.
unpublish
async unpublish(stream: Stream): Promise<void>
[Function Description]
Cancels the publication of a local stream.
[Request Parameters]
stream: (mandatory) local stream object. The type is Stream.
[Response Parameters]
Promise<void>: returns a Promise object.
subscribe
async subscribe(stream: RemoteStream, option?: SubscribeOption): Promise<void>
[Function Description]
Subscribes to a remote audio and video media stream. When a user subscribes to a remote media stream, the user will receive the Client.on('stream-subscribed') event notification. Then, the user can play the stream.
- stream: (mandatory) remote stream object, which can be obtained from the stream-added event. The type is RemoteStream.
- option: (optional) video or audio subscription option. If this parameter is not passed, the audio and video with the highest resolution are subscribed. The type is SubscribeOption.
SubscribeOption is defined as:
{
- video: (optional) whether to subscribe to video. The type is Boolean. The default value is false.
- audio: (optional) whether to subscribe to audio. The type is Boolean. The default value is false.
- resolutionIds: (optional) resolution IDs of the videos to be subscribed to. The type is string[]. This parameter is valid only when video is set to true. The value can be obtained by calling getStreamInfo. If resolutionIds is not passed, the video with the highest resolution is subscribed by default. This parameter is added in version 1.8.0.
}
[Response Parameters]
Promise<void>: returns a Promise object.
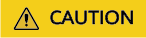
The video and audio parameters in SubscribeOption cannot be set to false at the same time.
unsubscribe
async unsubscribe(stream: Stream,option?: SubscribeOption): Promise<void>
[Function Description]
Unsubscribes from a remote audio and video media stream.
[Request Parameters]
- stream: (mandatory) remote stream object, which can be obtained from the stream-added event. The type is RemoteStream.
- option: (optional) unsubscription option. If this parameter is not passed, audio and videos of all resolutions will be unsubscribed from. The type is SubscribeOption.
SubscribeOption is defined as: {
- video: (optional) whether to unsubscribe from video. The type is Boolean. The default value is false.
- audio: (optional) whether to unsubscribe from audio. The type is Boolean. The default value is false.
- resolutionIds: (optional) resolution IDs of the videos to be unsubscribed from. The type is string[]. This parameter is valid only when video is set to true. The value can be obtained by calling getStreamInfo. If resolutionIds is not passed, videos of all resolutions will be unsubscribed from.
}
[Response Parameters]
Promise<void>: returns a Promise object.
batchSubscribe
async batchSubscribe(subscribeInfos: SubscribeParam[]): Promise<void>
[Function Description]
Subscribes to videos of multiple users in batches. When a user subscribes to a remote media stream, the user will receive the Client.on('stream-subscribed') event notification. Then, users can play the subscribed stream.
[Request Parameters]
subscribeInfos: (mandatory) information of all remote users' video to be subscribed to. The type is SubscribeParam[].
SubscribeParam is defined as: {
- userId: (mandatory) ID of the user to be subscribed to. The type is string.
- resolutionIds: (optional) resolution of user videos to be subscribed to. The type is string[]. If resolutionIds is not passed, the video with the highest resolution is subscribed by default.
- minResolution: (optional) minimum resolution when adaptive video resolution is enabled. The type is ResolutionType. Enumerated values of ResolutionType are FHD, HD, SD, and LD.
}
[Response Parameters]
Promise<void>: returns a Promise object.
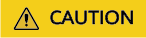
- This API is used to subscribe to the video of a remote user but not the audio of the remote user.
- Different from the incremental subscription mode of the subscribe API, the input parameters of this API form the list of all users to be subscribed to.
switchRole
async switchRole(role: number,authorization: Authorization): Promise<void>
[Function Description]
Switches the user role after a user joins a room.
- role: (mandatory) The type is number.
- 0: joiner, who can send and receive audio and video.
- 2: player, who only receives others' audio and video but does not send their own audio and video.
- authorization: authentication information. This parameter is mandatory. The type is Authorization. This parameter is added in version 2.0.0. Authorization is defined as follows:
- signature: (mandatory) authentication signature string. The type is string[512]. For details about how to generate a signature, see Access Authentication.
- ctime: (mandatory) authentication signature UTC timestamp, in seconds. The type is string.
[Response Parameters]
Promise<void>: returns a Promise object.
on
on(event: string, handler: function): void
[Function Description]
Registers the callback for a client object event.
- event: (mandatory) event name. The type is string. For details, see ClientEvent.
- handler: (mandatory) event processing method. The type is function.
[Response Parameters]
None
off
off(event: string, handler: function): void
[Function Description]
Deregisters the callback for a client object event.
- event: (mandatory) event name. The type is string. For details, see ClientEvent.
- handler: (mandatory) event processing method. The type is function.
[Response Parameters]
None
getConnectionState
getConnectionState(): ConnectionState
[Function Description]
Obtains the client connection state.
[Request Parameters]
None
[Response Parameters]
- CONNECTING
- CONNECTED
- RECONNECTING
- DISCONNECTED
getTransportStats
getTransportStats(): Promise<TransportStats>
[Function Description]
Obtains the network transmission statistics. This API can be called only after publish is called. In the dual-stream scenario, statistics of the video with the highest resolution collected by cameras are obtained by default.
[Request Parameters]
None
[Response Parameters]
The type of response parameters is TransportStats. Currently, TransportStats supports the following attributes: {
- bytesSent: number of sent bytes. The type is number.
- bytesReceived: number of received bytes. The type is number.
- sendBitrate: output bit rate, in kbit/s. The type is number.
- recvBitrate: input bit rate, in kbit/s. The type is number.
- rtt: round-trip time (RTT) from the SDK to the edge server, in milliseconds. The type is number.
}
getLocalAudioStats
getLocalAudioStats(): Promise<Map<string, LocalAudioStats>>
[Function Description]
Obtains statistics of local audio that has been sent.
[Request Parameters]
None
[Response Parameters]
- string: user ID.
- LocalAudioStats: local audio statistics, including the following attributes: {
- bytesSent: number of sent bytes. The type is number.
- packetsSent: number of sent packets. The type is number.
}
getLocalVideoStats
getLocalVideoStats(): Promise<Map<string, AllLocalVideoStats>>
[Function Description]
Obtains statistics of local video that has been sent.
[Request Parameters]
None
[Response Parameters]
- string: user ID.
- AllLocalVideoStats: local video statistics, including the following attributes: {
- mainStream: local video statistics. The type is LocalVideoStats[].
- subStream: local presentation statistics. The type is LocalVideoStats.
}
LocalVideoStats contains the following attributes: {- bytesSent: number of sent bytes. The type is number.
- packetsSent: number of sent packets. The type is number.
- framesEncoded: number of encoded frames. The type is number.
- framesSent: number of sent frames. The type is number.
- frameWidth: video width. The type is number.
- frameHeight: video height. The type is number.
}
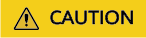
getLocalVideoStats and getLocalAudioStats APIs can be called only after the local stream is published and subscribed to by a remote end.
getRemoteAudioStats
getRemoteAudioStats(): Promise<Map<string, RemoteAudioStats>>
[Function Description]
Obtains remote audio statistics.
[Request Parameters]
None
[Response Parameters]
- string: user ID.
- RemoteAudioStats: remote audio statistics indicators, which can be:
- bytesReceived: number of received bytes. The type is number.
- packetsReceived: number of received packets. The type is number.
- packetsLost: number of lost packets. The type is number.
getRemoteVideoStats
getRemoteVideoStats(): Promise<Map<string, AllRemoteVideoStats>>
[Function Description]
Obtains remote video statistics.
[Request Parameters]
None
[Response Parameters]
- string: user ID.
- AllRemoteVideoStats: remote video statistics indicators, which can be: {
- mainStream: remote video statistics, including statistics about high-quality and low-quality streams. The type is RemoteVideoStats[].
- subStream: remote presentation statistics. The type is RemoteVideoStats.
}
RemoteVideoStats contains the following attributes: {
- bytesReceived: number of received bytes. The type is number.
- packetsReceived: number of received packets. The type is number.
- packetsLost: number of lost packets. The type is number.
- framesDecoded: number of decoded frames. The type is number.
- frameWidth: video width. The type is number.
- frameHeight: video height. The type is number.
}
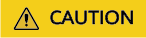
The resolution data of the remote video cannot be obtained on the Firefox browser.
enableTopThreeAudioMode
enableTopThreeAudioMode(enable: boolean): boolean
[Function Description]
Specifies whether to enable the top-N-audio mode (top three loudest participants) before joining a meeting.
[Request Parameters]
enable: (mandatory) The type is Boolean. The value true indicates that the top-N-audio mode is enabled, and the value false indicates that the top-N-audio mode is disabled. The default value is false.
[Response Parameters]
The type is Boolean. true indicates successful; false indicates failed.
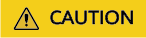
This API needs to be called before users join the room. This API is added in version 1.2.0.
setVolume4TopThree
setVolume4TopThree(volume: number): void
[Function Description]
Configures the audio volume after the top-N-audio mode (top three loudest participants) is enabled.
[Request Parameters]
volume: (mandatory) audio volume. The type is number. The value range is [0, 100].
[Response Parameters]
None
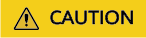
This API needs to be called after enableTopThreeAudioMode is called. This API is added in version 1.4.0.
muteAudio4TopThree
muteAudio4TopThree(enable: boolean): void
[Function Description]
Enables or disables the audio track in top-N-audio mode (tho three loudest participants) after the top-N-audio mode is enabled.
[Request Parameters]
enable: (mandatory) whether to disable audio tracks of the top-N-audio mode. The type is Boolean. true indicates disabled; false indicates enabled. The default value is false.
[Response Parameters]
None
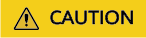
This API needs to be called after enableTopThreeAudioMode is called. This API is added in version 1.4.0.
enableStreamStateDetection
async enableStreamStateDetection(enable: boolean, interval: number): Promise<void>
[Function Description]
Enables or disables video stream state detection. After this function is enabled, the system can detect whether any remote users who are subscribed to by the local user in the room have no video stream. If a remote user has no video stream, the local user will receive the stream-interrupted event. If the video stream of a remote user is restored, the local user will receive the stream-recovered event.
[Request Parameters]
- enable: (mandatory) whether to enable video stream state detection. The type is Boolean. true indicates enabled; false indicates disabled. The default value is false.
- interval: (mandatory) duration for determining whether there is no video stream, in seconds. The type is number. The value range is [1, 60]. The recommended value is 3s.
[Response Parameters]
Promise<void>: returns a Promise object.
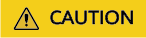
This API needs to be called after users join the room. This API is added in version 1.4.0.
changeUserName
async changeUserName(userName: string): Promise<boolean>
[Function Description]
Modifies the nickname of a user. Other users in the room will receive the remote-user-name-changed event notification.
[Request Parameters]
userName: (mandatory) new nickname of the user. The type is string[256].
[Response Parameters]
Promise<boolean>: Promise object. true indicates that the nickname is modified; false indicates that the nickname fails to be modified.
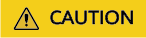
This API needs to be called after users join the room. This API is added in version 1.5.0.
enableRtcStats
async enableRtcStats(enable: boolean, interval: number): Promise<void>
[Function Description]
Configures whether to enable the RTC audio and video stream statistics event. This API is added in version 2.0.3.
[Request Parameters]
enable: (mandatory) whether to enable the RTC audio and video stream statistics event. The type is Boolean. The value true indicates that the event is enabled, and the value false indicates that the event is disabled.
interval: (mandatory) interval of generating the RTC audio and video stream statistics event, in milliseconds. The type is number. This parameter is valid only when enable is set to true.
[Response Parameters]
Promise<void>: returns a Promise object.
setProxyServer
setProxyServer(server: string): void
[Function Description]
Configures the signaling proxy server. This API is used to deploy a reverse proxy server (such as Nginx) in an enterprise. It is newly added in version 2.0.3.
[Request Parameters]
servers: (mandatory) list of reverse proxy servers. The type is string. Proxy server format: http://ip:port / https://domain:port.
[Response Parameters]
None
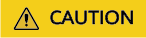
setProxyServer and setTurnServer APIs must be called before calling the join API.
setTurnServer
setTurnServer(turnServerConfig: TurnServerConfig): void
[Function Description]
Configures a proxy server. This API is used to deploy a reverse proxy server (such as Nginx) in an enterprise. It is newly added in version 2.0.3.
[Request Parameters]
turnServerConfig: proxy server configuration. (Mandatory) TurnServerConfig type.
TurnServerConfig is defined as follows: {
- turnServers: reverse proxy server address. (Mandatory) string[] type.
- udpPort: UDP port. (Optional) Number type.
- userName: reverse proxy server username. (Optional) String type.
- credential: reverse proxy server password. (Optional) String type.
}
[Response Parameters]
None
setNetworkBandwidth
setNetworkBandwidth(bandwidthParam: NetworkBandwidth): void
[Function Description]
Configures the maximum media bandwidth. This API is added in version 2.0.5.
[Request Parameters]
NetworkBandwidth is defined as: {
maxBandwidth: (mandatory) the maximum total media bandwidth. The value ranges from 3072 to 51200 in kbit/s. The type is number.
}
[Response Parameters]
None
renewSignature
renewSignature(ctime: string, signature: string): boolean
[Function Description]
Updates a signature.
[Request Parameters]
- ctime: time when the signature authentication expires. The value is the current UTC time (UNIX timestamp) of the system plus the authentication expiration time (recommended: 2 hours; maximum: < 12 hours). The unit is second. (Mandatory) string type.
- signature: signature. For details about how to generate a signature, see Access Authentication. (Mandatory) The value is of the string[512] type.
[Response Parameters]
boolean: A Boolean value is returned, indicating whether the signature has been updated.
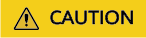
This API is added in version 2.0.8.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot