Performing Secondary Development After Interconnection
You can perform secondary development as required. Currently, the following examples are provided.
- Custom authentication information obtaining class: used to obtain IAM authentication information for accessing LakeFormation.
- Custom user information obtaining class: used to obtain the information of the user who accesses LakeFormation.
Custom Authentication Information Obtaining Class
The IdentityGenerator class is used to obtain IAM authentication information (token, permanent AK/SK, and temporary AK/SK and securityToken) for accessing LakeFormation.
LakeFormation provides a default class for obtaining authentication information. The AK/SK is obtained from the configuration file to generate authentication information.
- Develop code.
The implementation project is as follows. Add the lakeformation-lakecat-client dependency to the POM file of the Maven project.
<dependency> <groupId>com.huawei.lakeformation</groupId> <artifactId>lakeformation-lakecat-client</artifactId> <version>${lakeformation.version}</version> </dependency> //Add a class for obtaining authentication information to implement the IdentityGenerator API. /* * Copyright (c) Huawei Technologies Co., Ltd. 2023-2023. All rights reserved. */ package com.huawei.cloud.dalf.lakecat.examples; import com.huawei.cloud.dalf.lakecat.client.ConfigCenter; import com.huawei.cloud.dalf.lakecat.client.identity.Identity; import com.huawei.cloud.dalf.lakecat.client.identity.IdentityGenerator; import java.util.Collections; /** * Identity information generator example * */ public class LakeFormationExampleIdentityGenerator implements IdentityGenerator { public String token; @Override public void initialize(ConfigCenter configCenter) { //Perform initialization. } @Override public Identity generateIdentity() { //Return the IAM authentication information. } }
- Configure integration.
Use Maven to pack the code and place the JAR package in the hive-xxx/lib directory. xxx indicates the Hive kernel version.
Add the following configuration to hive-site.xml:
<!--Authentication information obtaining class. The value is for reference only.--> <property> <name>lakecat.auth.identity.util.class</name> <value>com.huawei.cloud.dalf.lakecat.examples.LakeFormationExampleIdentityGenerator</value> </property>
- Restart the Hive service.
Custom User Information Obtaining Class
The AuthenticationManager class is used to obtain the information of the user who accesses LakeFormation, which may be an IAM user or a local LDAP user. The default user information obtaining class obtains the user information using UserGroupInformation.getCurrentUser().
In addition to the default user information obtaining class introduced here, you can implement other user information obtaining method.
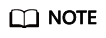
If user authentication information is used to access LakeFormation, the user information must be consistent with the user identity information (that is, the username and source must be consistent).
- Develop code.
The implementation project is as follows. Add the lakeformation-lakecat-client dependency to the POM file of the Maven project.
<dependency> <groupId>com.huawei.lakeformation</groupId> <artifactId>lakeformation-lakecat-client</artifactId> <version>${lakeformation.version}</version> </dependency> User information obtaining class, which implements the AuthenticationManager API. /* * Copyright (c) Huawei Technologies Co., Ltd. 2023-2023. All rights reserved. */ package com.huawei.cloud.dalf.lakecat.examples; import com.huawei.cloud.dalf.lakecat.client.ConfigCenter; import com.huawei.cloud.dalf.lakecat.client.identity.AuthenticationManager; import com.huawei.cloud.dalf.lakecat.client.model.Principal; public class ExampleAuthenticationManager implements AuthenticationManager { @Override public void initialize(ConfigCenter configCenter) { //Perform initialization. } @Override public Principal getCurrentUser() { //Return the information about the current user. } }
- Configure integration.
Use Maven to pack the code and place the JAR package in the hive-xxx/lib directory. xxx indicates the Hive kernel version.
Add the following configuration to hive-site.xml:
<!--Optional parameter. Authentication manager implementation class, which is used to obtain the information of the current user. The value configured here is for reference only.--> <property> <name>lakeformation.authentication.manager.class</name> <value>com.huawei.cloud.dalf.lakecat.examples.ExampleAuthenticationManager</value> </property> <!--Optional parameter, which specifies whether to specify the current user as the resource owner during resource creation. The default value is false.--> <property> <name>lakeformation.owner.designate</name> <value>true</value> </property> </configuration>
- Restart the Hive service.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot