Optimizing the Jedis Connection Pool
Overview
JedisPool is the connection pool of the Jedis client. This section describes how to configure JedisPool for better Redis performance and resource utilization.
Using JedisPool
The following Maven dependency is for Jedis 5.1.3.
<dependency> <groupId>redis.clients</groupId> <artifactId>jedis</artifactId> <version>5.1.3</version> </dependency>
Jedis manages resource pools using Apache Commons-pool2. The key parameter GenericObjectPoolConfig (resource pool) is required to define JedisPool. This parameter can be used as follows. For details, see JedisPool Parameters.
GenericObjectPoolConfig jedisPoolConfig = new GenericObjectPoolConfig(); jedisPoolConfig.setMaxTotal(...); jedisPoolConfig.setMaxIdle(...); jedisPoolConfig.setMinIdle(...); jedisPoolConfig.setMaxWaitMillis(...);
JedisPool is initialized as follows:
// redisHost is the IP address of the Redis instance. redisPort is the port of the Redis instance. redisPassword is the password of the Redis instance. timeout is the connection or read/write timeout. JedisPool jedisPool = new JedisPool(jedisPoolConfig, redisHost, redisPort, timeout, redisPassword); // Execution Jedis jedis = null; try { jedis = jedisPool.getResource(); // Specific command jedis.set("key", "value"); } catch (Exception e) { logger.error(e.getMessage(), e); } finally { // With JedisPool, Jedis connections will be returned to the resource pool. if (jedis != null) jedis.close(); }
JedisPool Parameters
JedisPool manages Jedis connections in a connection pool, which ensures resource control and thread security. GenericObjectPoolConfig can improve Redis performance at lower costs. Table 1 and Table 2 describe parameters and their configuration suggestions.
Parameter |
Description |
Default Value |
Suggestion |
---|---|---|---|
maxTotal |
Maximum number of connections in a resource pool |
8 |
|
maxIdle |
Maximum number of idle connections allowed in a resource pool |
8 |
|
minIdle |
Minimum number of idle connections allowed in a resource pool |
0 |
|
blockWhenExhausted |
Whether a caller awaits when a resource pool is used up
maxWaitMillis is valid only when this parameter is set to true. |
true |
Use the default value. |
maxWaitMillis |
Maximum time that a caller waits after a resource pool is used up, in milliseconds. The value -1 indicates that the caller keeps waiting. |
-1 |
Specify a time. |
testOnBorrow |
Whether to test validity of connections borrowed from a resource pool (ping). Invalid connections will be removed.
|
false |
Set to false during heavy service hours, saving resources a ping. |
testOnReturn |
Whether to test validity of connections returned to a resource pool (ping). Invalid connections will be removed.
|
false |
Set to false during heavy service hours, saving resources a ping. |
jmxEnabled |
Whether to enable JMX monitoring.
|
true |
Enable it, also for the application. |
Table 2 describes the parameters for testing idle Jedis objects.
Parameter |
Description |
Default Value |
Suggestion |
---|---|---|---|
testWhileIdle |
Whether to test validity of idle connections using ping. Invalid ones will be destroyed. |
false |
true |
timeBetweenEvictionRunsMillis |
Interval of looking for idle resources, in milliseconds. -1: disabled |
-1 |
Specify an interval, use the default value, or use the JedisPoolConfig. |
minEvictableIdleTimeMillis |
Minimum idle duration in a resource pool, in milliseconds. Resources that are idle longer than this will be removed. |
1,800,000 (30 minutes) |
Specify it as required. Generally, use the default value. JedisPoolConfig can be used. |
numTestsPerEvictionRun |
Number of idle resources to be tested each time. |
3 |
Adjust this parameter as required. The value -1 indicates that all idle connections are tested. |
Jedis provides JedisPoolConfig which inherits certain idle connection testing settings of GenericObjectPoolConfig.
public class JedisPoolConfig extends GenericObjectPoolConfig { public JedisPoolConfig() { setTestWhileIdle(true); setMinEvictableIdleTimeMillis(60000); setTimeBetweenEvictionRunsMillis(30000); setNumTestsPerEvictionRun(-1); }}
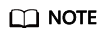
All of the default values are available in org.apache.commons.pool2.impl.BaseObjectPoolConfig.
Suggestions on Key Parameters
- maxTotal
Consider the following factors:
- The Redis concurrency required by services
- Execution duration on a client
- Redis resources, such as the number of shards
- maxTotal must be within the maximum number of Redis connections. For details about the maximum Redis connections, see How Do I View Current Concurrent Connections and Maximum Connections of a DCS Redis Instance?.
- Resource overhead. For example, control idle connections while avoiding frequently releasing and creating connections.
Assume that the average duration of executing a command is 1 ms. This execution covers the resource borrowing and returning, and network transmission. The QPS of a connection is about 1000 (1s/1 ms). The expected QPS per instance is 50,000 (Total service QPS/Number of Redis shards). Ideally, the required resource pool size (maxTotal) is 50 (50,000/1000).
In reality, more resources need to be reserved. Therefore, maxTotal can be greater. However, a large maxTotal allows many connections, which consume client and server resources. Furthermore, large commands with high QPS may block Redis and a large resource pool does not work.
- maxIdle and minIdle
maxIdle is the maximum number of connections required by services. maxTotal reserves resources. Do not use tiny maxIdle. Otherwise, new connection overhead occurs. minIdle controls idle resource testing.
Setting maxIdle to the same as maxTotal achieves an optimal connection pool and avoids performance impact caused by connection pool scaling. This combination fits in peak service hours, but causes resource wastes when the number of concurrent connections is small or maxIdle is excessively high.
The connection pool size of a node can be estimated based on the total QPS and the client scale.
- Using monitoring for appropriate values
In actual environments, an optimal parameter can be obtained through monitoring, such as JMX.
Common Errors
- Insufficient resources
The following two cases indicate that resources cannot be obtained from the pool. This is not necessarily due to small resource pools (see Suggestions on Key Parameters). The network, resource pool parameter settings, resource pool monitoring (JMX), code (for example, jedis.close() is not executed), slow queries, and DNS may also be the cause.
- Timeout:
redis.clients.jedis.exceptions.JedisConnectionException: Could not get a resource from the pool ...Caused by: java.util.NoSuchElementException: Timeout waiting for idle object at org.apache.commons.pool2.impl.GenericObjectPool.borrowObject
- When a resource pool is used up, it does not wait for resource release if blockWhenExhausted is set to false:
redis.clients.jedis.exceptions.JedisConnectionException: Could not get a resource from the pool ...Caused by: java.util.NoSuchElementException: Pool exhausted at org.apache.commons.pool2.impl.GenericObjectPool.borrowObject
- Timeout:
- JedisPool preheating
A project may time out after start for some reasons (for example, small timeout interval). When the maximum number of resources and minimum number of idle resources are defined by JedisPool, Jedis connections are not created in the connection pool. In initial use, if no resource in the pool is used, a new Jedis connection is created and then added to the resource pool. This process takes some time. Therefore, you are advised to preheat JedisPool based on the minimum number of idle connections after defining JedisPool. The following is an example:
List<Jedis> minIdleJedisList = new ArrayList<Jedis>(jedisPoolConfig.getMinIdle()); for (int i = 0; i < jedisPoolConfig.getMinIdle(); i++) { Jedis jedis = null; try { jedis = pool.getResource(); minIdleJedisList.add(jedis); jedis.ping(); } catch (Exception e) { logger.error(e.getMessage(), e); } finally { } } for (int i = 0; i < jedisPoolConfig.getMinIdle(); i++) { Jedis jedis = null; try { jedis = minIdleJedisList.get(i); jedis.close(); } catch (Exception e) { logger.error(e.getMessage(), e); } finally { } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot