Setting CORS Rules
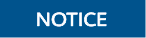
If you have any questions during development, post them on the Issues page of GitHub.
You can call set_bucket_cors_configuration() to set CORS rules for a bucket. If the bucket is configured with CORS rules, the newly set ones will overwrite the existing ones. Sample code is as follows:
Parameter Description
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
option |
The context of the bucket. For details, see Configuring option. |
Mandatory |
Bucket parameter |
obs_cors_conf_info |
obs_bucket_cors_conf * |
Mandatory |
For details about CORS rules, see the following table. |
conf_num |
unsigned int |
Mandatory |
Number of array members in the array obs_cors_conf_info. |
handler |
obs_response_handler* |
Mandatory |
Callback function |
callback_data |
void * |
Optional |
Callback data |
The following table describes the CORS rule structure obs_bucket_cors_conf.
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
id |
const char * |
Optional |
Object names in a bucket |
allowed_method |
const char ** |
Mandatory |
Method allowed by a CORS rule |
allowed_method_number |
unsigned int |
Mandatory |
Number of allowed_method |
allowed_origin |
const char ** |
Mandatory |
Indicates an origin that is allowed by a CORS rule. It is a character string and can contain a wildcard (*), and allows one wildcard character (*) at most. |
allowed_origin_number |
unsigned int |
Mandatory |
Number of allowed_origin. |
allowed_header |
const char ** |
Optional |
Indicates which headers are allowed in a PUT Bucket CORS request via the Access-Control-Request-Headers. If a request contains Access-Control-Request- Headers, only a CORS request that matches the configuration of allowed_header is considered as a valid request. Each allowed_header can contain at most one wildcard (*) and cannot contain spaces. |
allowed_header_number |
unsigned int |
Optional |
Number of allowed_header |
max_age_seconds |
const char * |
Optional |
Response time of CORS that can be cached by a client. It is expressed in seconds. Each CORS rule can contain only one max_age_seconds. It can be set to a negative value. |
expose_header |
const char ** |
Optional |
Indicates a supplemented header in CORS responses. The header provides additional information for clients. It cannot contain spaces. |
expose_header_number |
unsigned int |
Optional |
Number of expose_header. |
Sample Code
static void test_set_bucket_cors() { obs_options option; obs_status ret_status = OBS_STATUS_BUTT; obs_bucket_cors_conf bucketCorsConf; // Set option. init_obs_options(&option); option->bucket_options.hostName = "<your-endpoint>"; option->bucket_options.bucketName = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option->bucket_options.access_key = getenv("ACCESS_KEY_ID"); option->bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set callback function. obs_response_handler response_handler = { NULL, &response_complete_callback }; char *id_1= "1"; // Specify the browser's cache time of the returned results of OPTIONS requests for specific resources, in seconds. char *max_age_seconds = "100"; // Specify the request method, which can be GET, PUT, DELETE, POST, or HEAD. const char* allowedMethod_1[5] = {"GET","PUT","HEAD","POST","DELETE"}; // Specify the origin of the cross-domain request. const char* allowedOrigin_1[2] = {"obs.example.com", "www.example.com"}; // Specify response headers that users can access using application programs. const char* allowedHeader_1[2] = {"header-1", "header-2"}; // Additional headers carried in the response const char* exposeHeader_1[2] = {"hello", "world"}; memset(&bucketCorsConf, 0, sizeof(obs_bucket_cors_conf)); bucketCorsConf.id = id_1; bucketCorsConf.max_age_seconds = max_age_seconds; bucketCorsConf.allowed_method = allowedMethod_1; bucketCorsConf.allowed_method_number = 5; bucketCorsConf.allowed_origin = allowedOrigin_1; bucketCorsConf.allowed_origin_number = 2; bucketCorsConf.allowed_header = allowedHeader_1; bucketCorsConf.allowed_header_number = 2; bucketCorsConf.expose_header = exposeHeader_1; bucketCorsConf.expose_header_number = 2; set_bucket_cors_configuration(&option, &bucketCorsConf, 1, &response_handler, &ret_status); if (OBS_STATUS_OK == ret_status) { printf("set_bucket_cors success.\n"); } else { printf("set_bucket_cors faied(%s).\n", obs_get_status_name(ret_status)); } }

allowed_origin, allowed_method, and allowed_header can contain at most one wildcard (*). Wildcard characters (*) indicate that all origins, operations, or headers are allowed.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot