GeminiDB Redis API Pub/Sub
Huawei Cloud GeminiDB Redis is fully compatible with Pub/Sub of open-source Redis. This section describes how to configure this model.
Pub/Sub
SUBSCRIBE, UNSUBSCRIBE, and PUBLISH implement the publish–subscribe pattern. In this pattern, publishers do not directly send messages to a specific subscriber but publish them to a channel. All subscribers who are interested in this channel can receive the messages. Pub/Sub enables the decoupling of the publisher and subscribers, eliminating the need for publishers to know their subscribers.
Application Scenarios
Pub/Sub plays an important role in many scenarios, for example:
- Real-time chat
In IM applications, messages need to be quickly transferred. With Pub/Sub, users can subscribe to their own chat channels. After message are published, the subscribes on this channel receive the messages immediately. In this manner, real-time performance and high efficiency can be achieved.
- Real-time notification system
On e-commerce websites or social media platforms, users need to receive notifications such as order status updates, comments, and likes in real time. With Pub/Sub of GeminiDB Redis API, the system can immediately publish a notification when the status changes, and all related users will receive the notification in a timely manner.
- Monitoring and log system
In the microservice architecture, the Pub/Sub model can be used for status monitoring and log collection between services. Services can publish status information or log messages to specific channels. The monitoring service or log collection service can subscribe to these channels to implement real-time monitoring and data collection.
- Real-time gaming messages
In an online game, data between players each time an action occurs needs to be synchronized in time. Pub/Sub can be used for message transfer and game event notification to ensure that all players receive status updates at the same time.
- Data stream processing
Real-time processing and analysis are key to data stream applications. With Pub/Sub, data producers can publish data streams, and consumers can subscribe to these streams for real-time processing and analysis.
Basic operations
For example, to subscribe to "channel11" and "ch:00," clients can run the following command:
SUBSCRIBE channel11 ch:00
These clients will receive messages on these channels from other clients in the sequence in which the messages were sent.
Advanced function:
Pub/Sub supports pattern matching. Clients may subscribe to glob-style patterns to receive all the messages sent to channel names matching a given pattern. For example:
PSUBSCRIBE news.*
Subscribers will receive all messages sent to channels such as news.art.figurative and news.music.jazz.
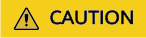
- Message loss: Pub/Sub does not ensure message durability. Therefore, messages may be lost when the network is faulty or a subscriber is not connected.
- Performance: In a high-concurrency environment, the Pub/Sub performance may be limited. Performance need to be tested and improved based on specific scenarios.
- If both SUBSCRIBE and PSUBSCRIBE are executed, duplicate messages may be received. Check whether the business logic is correct.
Java Sample Code (Jedis)
Message publisher
import redis.clients.jedis.Jedis; public class GeminiDBPubClient { private Jedis jedis; public GeminiDBPubClient(String ip, int port, String password){ jedis = new Jedis(ip, port); // The instance password for GeminiDB. String authString = jedis.auth(password); if (!authString.equals("OK")) { System.err.println("AUTH Failed: " + authString); return; } } public void pub(String channel, String message){ System.out.println(" >>> Publish > Channel: " + channel + " > Sent Message: " + message); jedis.publish(channel, message); } public void close(String channel){ System.out.println(" >>> Publish End > Channel:" + channel + " > Message:quit"); // The message publisher has finished sending, sending a "quit" message. jedis.publish(channel, "quit"); } }
Message subscriber
import redis.clients.jedis.Jedis; import redis.clients.jedis.JedisPubSub; public class GeminiDBSubClient extends Thread { private Jedis jedis; private String channel; private JedisPubSub listener; public GeminiDBSubClient(String ip, int port, String password){ jedis = new Jedis(host,port); // The instance password for GeminiDB. String authString = jedis.auth(password); //password if (!authString.equals("OK")) { System.err.println("AUTH Failed: " + authString); return; } } public void setChannelAndListener(JedisPubSub listener, String channel){ this.listener=listener; this.channel=channel; } private void subscribe(){ if(listener==null || channel==null){ System.err.println("Error:SubClient> listener or channel is null"); } System.out.println(" >>> Subscribe > Channel:" + channel); // The receiver will block the process while listening for subscribed messages until it receives a "quit" message (passive mode) or actively cancels the subscription. jedis.subscribe(listener, channel); } public void unsubscribe(String channel){ System.out.println(" >>> Unsubscribe > Channel:" + channel); listener.unsubscribe(channel); } @Override public void run(){ try { System.out.println("----------Subscribe Start-------"); subscribe(); System.out.println("----------Subscribe End-------"); } catch(Exception e){ e.printStackTrace(); } } }
Message listener
import redis.clients.jedis.JedisPubSub; public class GeminiDBListener extends JedisPubSub { @Override public void onMessage(String channel, String message) { System.out.println(" <<< Subscribe < Channel:" + channel + " > Receive Message:" + message ); // When the received message is "quit," unsubscribe (passive mode). if(message.equalsIgnoreCase("quit")){ this.unsubscribe(channel); } } @Override public void onPMessage(String pattern, String channel, String message) { // TODO Auto-generated method stub } @Override public void onSubscribe(String channel, int subscribedChannels) { // TODO Auto-generated method stub } @Override public void onUnsubscribe(String channel, int subscribedChannels) { // TODO Auto-generated method stub } @Override public void onPUnsubscribe(String pattern, int subscribedChannels) { // TODO Auto-generated method stub } @Override public void onPSubscribe(String pattern, int subscribedChannels) { // TODO Auto-generated method stub } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot