Deleting Objects
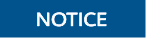
If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the .
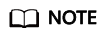
Exercise caution when performing this operation. If the versioning function is disabled for the bucket where the object is located, the object cannot be restored after being deleted.
Deleting a Single Object
You can call ObsClient.deleteObject to delete a single object. Sample code is as follows:
// Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY_ID. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); String endPoint = "https://your-endpoint"; // Create an instance of ObsClient. ObsClient obsClient = new ObsClient(ak, sk, endPoint); obsClient.deleteObject("bucketname", "objectname");
Deleting Objects in a Batch
You can call ObsClient.deleteObjects to delete objects in a batch.
A maximum of 1000 objects can be deleted each time. Two response modes are supported: verbose (detailed) and quiet (brief).
- In verbose mode (default mode), the returned response includes the deletion result of each requested object.
- In quiet mode, the returned response includes only results of objects failed to be deleted.
Sample code:
// Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY_ID. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); String endPoint = "https://your-endpoint"; ObsClient obsClient = new ObsClient(ak, sk, endPoint); ListVersionsRequest request = new ListVersionsRequest("bucketname"); // Delete 100 objects at a time. request.setMaxKeys(100); ListVersionsResult result; do { result = obsClient.listVersions(request); DeleteObjectsRequest deleteRequest = new DeleteObjectsRequest("bucketname"); for(VersionOrDeleteMarker v : result.getVersions()) { deleteRequest.addKeyAndVersion(v.getKey(), v.getVersionId()); } DeleteObjectsResult deleteResult = obsClient.deleteObjects(deleteRequest); // Obtain the successfully deleted objects. Log.i("DeletesObjects",deleteResult.getDeletedObjectResults()); // Obtain the list of objects failed to be deleted. Log.i("DeletesObjects", deleteResult.getErrorResults()); request.setKeyMarker(deleteResult.getNextKeyMarker()); request.setVersionIdMarker(deleteResult.getNextVersionIdMarker()); }while(result.isTruncated());
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.