Creating a Bucket
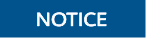
If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the .
You can call ObsClient.createBucket to create a bucket.
Creating a Bucket with Parameters Specified
When creating a bucket, you can specify the ACL, storage class, and location for the bucket. OBS provides three storage classes for buckets. For details, see Storage Class. Sample code is as follows:
// Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY_ID. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); String endPoint = "https://your-endpoint"; // Create an ObsClient instance. ObsClient obsClient = new ObsClient(ak, sk, endPoint); ObsBucket obsBucket = new ObsBucket(); obsBucket.setBucketName("bucketname"); // Set the access permission for the bucket to public-read. (The default value is private.) obsBucket.setAcl(AccessControlList.REST_CANNED_PUBLIC_READ); // Set the storage class to Archive. obsBucket.setBucketStorageClass(StorageClassEnum.COLD); // Set the bucket location. obsBucket.setLocation("bucketlocation"); // Create a bucket. try{ // The bucket is successfully created. HeaderResponse response =obsClient.createBucket(obsBucket); Log.i("CreateBucket", response.getRequestId()); } catch (ObsException e) { // Failed to create a bucket. Log.e("CreateBucket", "Response Code: " + e.getResponseCode()); Log.e("CreateBucket", "Error Message: " + e.getErrorMessage()); Log.e("CreateBucket", "Error Code: " + e.getErrorCode()); Log.e("CreateBucket", "Request ID: " + e.getErrorRequestId()); Log.e("CreateBucket", "Host ID: " + e.getErrorHostId()); }
Creating a Bucket Directly
Sample code:
// Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY_ID. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); String endPoint = "https://your-endpoint"; // Create an ObsClient instance. ObsClient obsClient = new ObsClient(ak, sk, endPoint); // Create a bucket. try{ // The bucket is successfully created. HeaderResponse response = obsClient.createBucket("bucketname"); Log.i("CreateBucket", response.getRequestId()); } catch (ObsException e) { // Failed to create a bucket. Log.e("CreateBucket", "Response Code: " + e.getResponseCode()); Log.e("CreateBucket", "Error Message: " + e.getErrorMessage()); Log.e("CreateBucket", "Error Code: " + e.getErrorCode()); Log.e("CreateBucket", "Request ID: " + e.getErrorRequestId()); Log.e("CreateBucket", "Host ID: " + e.getErrorHostId()); }
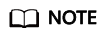
- Bucket names are globally unique. Ensure that the bucket you create is named differently from any other bucket.
- A bucket name must comply with the following rules:
- Contains 3 to 63 characters, chosen from lowercase letters, digits, hyphens (-), and periods (.), and starts with a digit or letter.
- Cannot be an IP address or similar.
- Cannot start or end with a hyphen (-) or period (.)
- Cannot contain two consecutive periods (.), for example, my..bucket.
- Cannot contain periods (.) and hyphens (-) adjacent to each other, for example, my-.bucket or my.-bucket.
- If you create buckets of the same name in a region, no error will be reported and the bucket properties comply with those set in the first creation request.
- The bucket created in the previous example is of the default ACL (private), in the OBS Standard storage class, and in the default region.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.